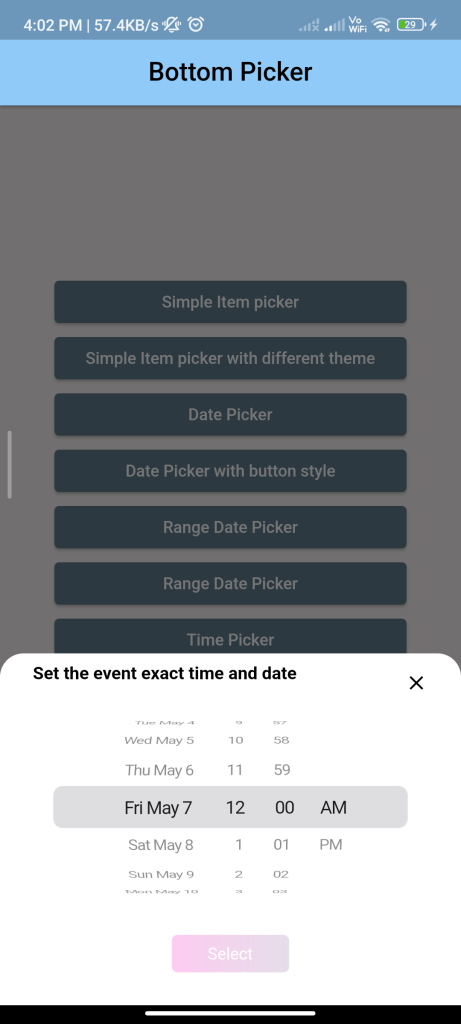
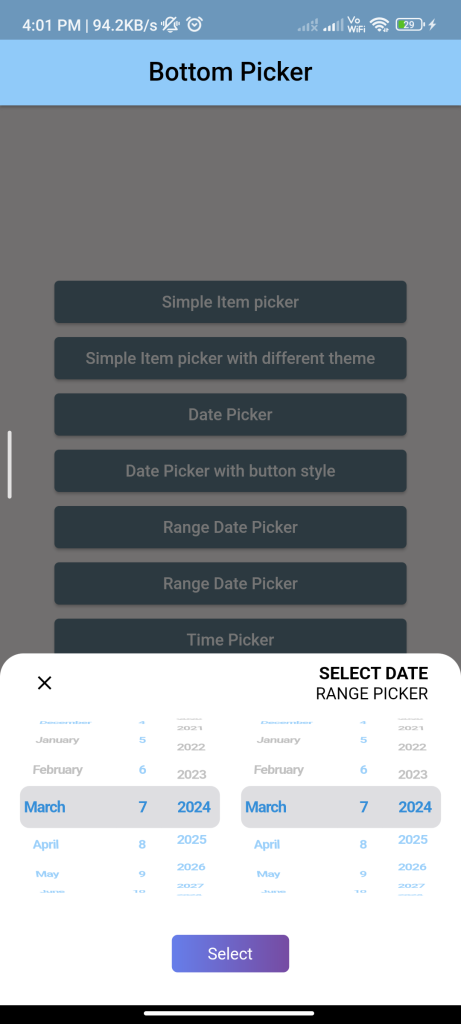
Introduction:
Implementing a bottom picker in Flutter allows users to select a value from a list or range of options displayed at the bottom of the screen. The bottom_picker
package provides a simple way to integrate a bottom picker into your app. This tutorial will guide you through implementing a bottom picker using the bottom_picker
package.
Content:
Step 1: Add the dependency:
Include the bottom_picker
package in your pubspec.yaml
file:
dependencies:
bottom_picker: ^1.0.0
Run flutter pub get
to install the package.
Step 2: Import the package:
Import the bottom_picker
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:bottom_picker/bottom_picker.dart';
Step 3: Create a BottomPicker widget:
Define a stateful widget and implement the BottomPicker
widget to provide a bottom picker:
class BottomPickerScreen extends StatefulWidget {
@override
_BottomPickerScreenState createState() => _BottomPickerScreenState();
}
class _BottomPickerScreenState extends State<BottomPickerScreen> {
String _selectedValue = '';
Future<void> _showPicker(BuildContext context) async {
final value = await BottomPicker.showSinglePicker(
context: context,
items: ['Option 1', 'Option 2', 'Option 3', 'Option 4'],
pickerHeight: 200,
);
if (value != null) {
setState(() {
_selectedValue = value;
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Bottom Picker Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () => _showPicker(context),
child: Text('Open Picker'),
),
),
);
}
}
In this example, the _showPicker
method is called to display the bottom picker when the button is pressed. The BottomPicker.showSinglePicker
method is used to show a single selection picker with a list of options. The selected value is stored in the _selectedValue
variable.
Step 4: Run the app:
Run your Flutter app to see the bottom picker in action. Press the button to open the bottom picker and select an option.
Sample Code:
// ignore_for_file: avoid_print, prefer_const_constructors, prefer_const_literals_to_create_immutables
import 'package:bottom_picker/bottom_picker.dart';
import 'package:bottom_picker/resources/arrays.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class BottomPickerPage extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blueGrey,
),
home: Scaffold(
body: ExampleApp(),
),
);
}
}
class ExampleApp extends StatelessWidget {
final countryList = [
Text('Algeria 🇩🇿'),
Text('Maroco 🇲🇦'),
Text('Tunisia 🇹🇳'),
Text('Palestine 🇵🇸'),
Text('Egypt 🇪🇬'),
Text('Syria 🇸🇾'),
Text('Irak 🇮🇶'),
Text('Mauritania 🇲🇷')
];
final buttonWidth = 300.0;
@override
Widget build(BuildContext context) {
return Container(
color: Color(0xffF6F2F2),
width: double.infinity,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openSimpleItemPicker(context, countryList);
},
child: Text('Simple Item picker'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openSecondSimpleItemPicker(context, countryList);
},
child: Text('Simple Item picker with different theme'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openDatePicker(context);
},
child: Text('Date Picker'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openDatePickerWithButtonStyle(context);
},
child: Text('Date Picker with button style'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openRangeDatePicker(context);
},
child: Text('Range Date Picker'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openArabicRangeDatePicker(context);
},
child: Text('Range Date Picker'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openTimePicker(context);
},
child: Text('Time Picker'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openDateTimePicker(context);
},
child: Text('Date and Time Picker'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openDateTimePickerWithCustomButton(context);
},
child: Text('Bottom picker with custom button'),
),
),
SizedBox(
width: buttonWidth,
child: ElevatedButton(
onPressed: () {
_openPickerWithCustomPickerTextStyle(context);
},
child: Text(
'Bottom picker with custom picker text style',
style: TextStyle(
fontSize: 12,
),
),
),
),
],
),
);
}
void _openSimpleItemPicker(BuildContext context, List<Text> items) {
BottomPicker(
items: items,
title: 'Choose your country',
titleStyle: TextStyle(fontWeight: FontWeight.bold, fontSize: 15),
backgroundColor: Colors.yellow.withOpacity(0.6),
bottomPickerTheme: BottomPickerTheme.morningSalad,
onSubmit: (index) {
print(index);
},
buttonAlignment: MainAxisAlignment.start,
displaySubmitButton: false,
).show(context);
}
void _openSecondSimpleItemPicker(BuildContext context, List<Text> items) {
BottomPicker(
items: items,
selectedItemIndex: 1,
title: 'CHAOS COUNTRY',
titleStyle: TextStyle(fontWeight: FontWeight.bold, fontSize: 15),
onChange: (index) {
print(index);
},
onSubmit: (index) {
print(index);
},
bottomPickerTheme: BottomPickerTheme.morningSalad,
layoutOrientation: LayoutOrientation.rtl,
).show(context);
}
void _openDatePicker(BuildContext context) {
BottomPicker.date(
title: 'Set your Birthday',
dateOrder: DatePickerDateOrder.dmy,
initialDateTime: DateTime(1996, 10, 22),
maxDateTime: DateTime(1998),
minDateTime: DateTime(1980),
pickerTextStyle: TextStyle(
color: Colors.blue,
fontWeight: FontWeight.bold,
fontSize: 12,
),
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.blue,
),
onChange: (index) {
print(index);
},
onSubmit: (index) {
print(index);
},
bottomPickerTheme: BottomPickerTheme.plumPlate,
).show(context);
}
void _openDatePickerWithButtonStyle(BuildContext context) {
BottomPicker.date(
title: 'Set your Birthday',
dateOrder: DatePickerDateOrder.dmy,
initialDateTime: DateTime(1996, 10, 22),
maxDateTime: DateTime(1998),
minDateTime: DateTime(1980),
pickerTextStyle: TextStyle(
color: Colors.blue,
fontWeight: FontWeight.bold,
fontSize: 12,
),
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.blue,
),
onChange: (index) {
print(index);
},
onSubmit: (index) {
print(index);
},
bottomPickerTheme: BottomPickerTheme.plumPlate,
buttonStyle: BoxDecoration(
color: Colors.blue,
borderRadius: BorderRadius.circular(20),
border: Border.all(
color: Colors.blue[200]!,
),
),
buttonWidth: 200,
buttonContent: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 10,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Text(
'Select date',
style: TextStyle(
color: Colors.white,
),
),
Icon(
Icons.arrow_forward_ios,
color: Colors.white,
size: 15,
)
],
),
),
).show(context);
}
void _openRangeDatePicker(BuildContext context) {
BottomPicker.range(
title: 'Set date range',
description: 'Please select a first date and an end date',
dateOrder: DatePickerDateOrder.dmy,
minFirstDate: DateTime.now(),
initialFirstDate: DateTime.now().add(Duration(days: 1)),
pickerTextStyle: TextStyle(
color: Colors.blue,
fontWeight: FontWeight.bold,
fontSize: 12,
),
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.black,
),
descriptionStyle: TextStyle(
color: Colors.black,
),
onRangeDateSubmitPressed: (firstDate, secondDate) {
print(firstDate);
print(secondDate);
},
bottomPickerTheme: BottomPickerTheme.plumPlate,
).show(context);
}
void _openArabicRangeDatePicker(BuildContext context) {
BottomPicker.range(
title: 'SELECT DATE',
description: 'RANGE PICKER',
dateOrder: DatePickerDateOrder.dmy,
layoutOrientation: LayoutOrientation.rtl,
pickerTextStyle: TextStyle(
color: Colors.blue,
fontWeight: FontWeight.bold,
fontSize: 12,
),
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.black,
),
descriptionStyle: TextStyle(
color: Colors.black,
),
onRangeDateSubmitPressed: (firstDate, secondDate) {
print(firstDate);
print(secondDate);
},
bottomPickerTheme: BottomPickerTheme.plumPlate,
).show(context);
}
void _openTimePicker(BuildContext context) {
BottomPicker.time(
title: 'Set your next meeting time',
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.orange,
),
onSubmit: (index) {
print(index);
},
onClose: () {
print('Picker closed');
},
bottomPickerTheme: BottomPickerTheme.orange,
use24hFormat: true,
initialTime: Time(
minutes: 23,
),
maxTime: Time(
hours: 17,
),
).show(context);
}
void _openDateTimePicker(BuildContext context) {
BottomPicker.dateTime(
title: 'Set the event exact time and date',
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.black,
),
onSubmit: (date) {
print(date);
},
onClose: () {
print('Picker closed');
},
minDateTime: DateTime(2021, 5, 1),
maxDateTime: DateTime(2021, 8, 2),
initialDateTime: DateTime(2021, 5, 1),
gradientColors: [
Color(0xfffdcbf1),
Color(0xffe6dee9),
],
).show(context);
}
void _openDateTimePickerWithCustomButton(BuildContext context) {
BottomPicker.dateTime(
title: 'Set the event exact time and date',
titleStyle: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 15,
color: Colors.black,
),
onSubmit: (date) {
print(date);
},
onClose: () {
print('Picker closed');
},
buttonSingleColor: Colors.pink,
minDateTime: DateTime(2021, 5, 1),
maxDateTime: DateTime(2021, 8, 2),
gradientColors: [
Color(0xfffdcbf1),
Color(0xffe6dee9),
],
).show(context);
}
void _openPickerWithCustomPickerTextStyle(BuildContext context) {
BottomPicker(
items: [
Text('Leonardo DiCaprio'),
Text('Johnny Depp'),
Text('Robert De Niro'),
Text('Tom Hardy'),
Text('Ben Affleck'),
],
title: 'Select the actor',
pickerTextStyle: TextStyle(
color: Colors.black,
fontWeight: FontWeight.bold,
),
closeIconColor: Colors.red,
).show(context);
}
}
Output:
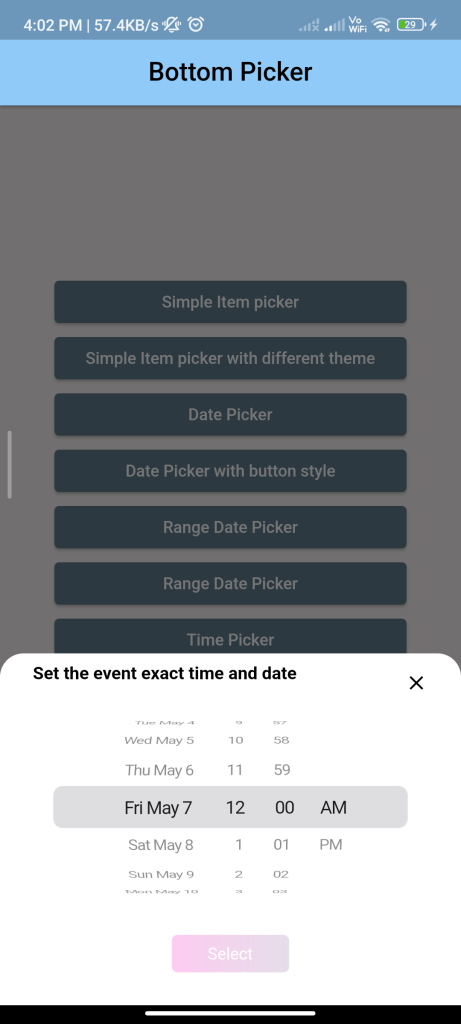
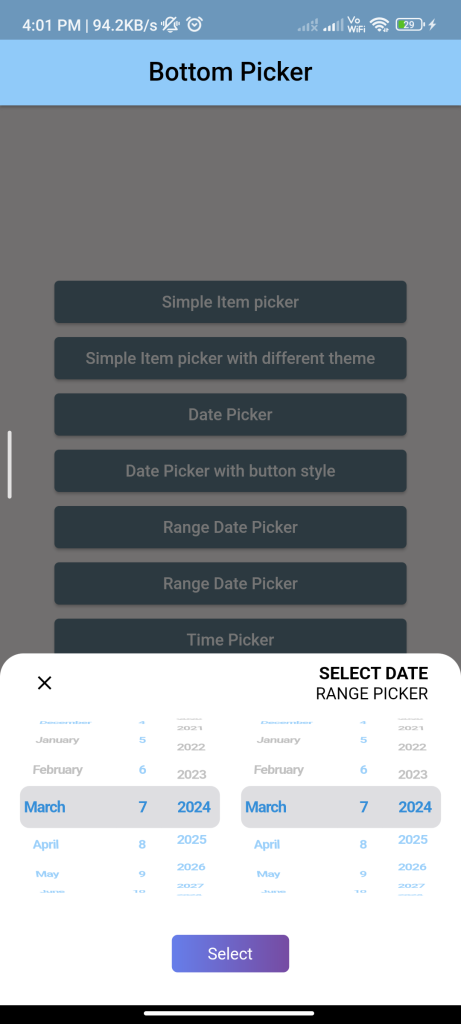
Conclusion:
By following these steps, you can easily implement a bottom picker in Flutter using the bottom_picker
package. This provides a convenient way for users to select a value from a list of options in your app.