Introduction:
Carousels or sliders are widely used in mobile app interfaces to display multiple items or images in a dynamic and engaging manner. In Flutter, you can easily implement a carousel/slider to showcase content such as product images, news articles, or featured items. This tutorial will guide you through the process of implementing a carousel/slider in Flutter.
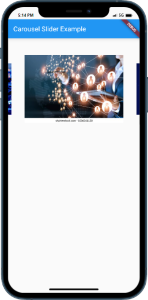
Content:
1. Create a new Flutter project:
Before we begin, make sure you have Flutter installed and set up on your machine. Create a new Flutter project by running the following command in your terminal:
flutter create carousel_slider_example
2. Open the main.dart file:
Once the project is created, navigate to the lib
folder and open the main.dart
file.
3. Import the necessary packages:
To work with carousels/sliders, import the following packages at the top of the main.dart
file:
import 'package:flutter/material.dart';
import 'package:carousel_slider/carousel_slider.dart';
4. Implement the carousel/slider:
Inside the main.dart
file, implement the carousel/slider by following these steps:
a. Declare a list of carousel items: Add a list of widgets that represent the items in the carousel/slider at the top of the main.dart
file:
List<Widget> carouselItems = [
Image.asset('assets/image1.jpg'),
Image.asset('assets/image2.jpg'),
Image.asset('assets/image3.jpg'),
];
b. Define the main function: Inside the main.dart
file, locate the main()
function and modify it as follows:
void main() {
runApp(MyApp());
}
c. Create the MyApp class: Define the MyApp
class, which extends StatelessWidget
:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Carousel/Slider Example',
home: HomeScreen(),
);
}
}
d. Create the HomeScreen class: Define the HomeScreen
class, which extends StatefulWidget
:
class HomeScreen extends StatefulWidget {
@override
_HomeScreenState createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Carousel/Slider Example'),
),
body: Center(
child: CarouselSlider(
items: carouselItems,
options: CarouselOptions(
height: 200.0,
enlargeCenterPage: true,
autoPlay: true,
aspectRatio: 16 / 9,
autoPlayCurve: Curves.fastOutSlowIn,
enableInfiniteScroll: true,
autoPlayAnimationDuration: Duration(milliseconds: 800),
viewportFraction: 0.8,
),
),
),
);
}
}
5. Run the app:
Save the changes and run the app using the following command in your terminal:
6. Observe the carousel/slider:
You will see a carousel/slider displayed in the app’s UI, showcasing the items from the carouselItems
list. You can customize the appearance and behavior of the carousel/slider by modifying the options provided in the CarouselOptions
widget.
Conclusion:
In this tutorial, you have learned how to implement a carousel/slider in Flutter. By following the steps outlined in this tutorial, you can create interactive and visually appealing slideshows of content in your Flutter app. Experiment with different options and customize the carousel/slider to suit your app’s requirements. Enhance the user experience and make your app more engaging with the addition of a carousel/slider.
Sample Code:
import 'package:flutter/material.dart';
import 'package:carousel_slider/carousel_slider.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Carousel Slider Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: CarouselSliderExample(),
);
}
}
class CarouselSliderExample extends StatelessWidget {
@override
final List<String> imageList = [
'https://www.shutterstock.com/image-photo/business-woman-drawing-global-structure-260nw-1006041130.jpg',
'https://images.ctfassets.net/pdf29us7flmy/2JqiMYwCbJuqPipSSZsDXA/72dcf2514ff223efa6a38d6a33d32266/networking-tips-Social.png?w=720&q=100&fm=jpg',
'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcR5QVXVqYiAeWeXBb88rZAbMIkI-vHF1U-rfQL7kcq1RrhuBCFia8sf8X-x1j9sXkJuA2Y&usqp=CAU',
];
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Carousel Slider Example'),
),
body: Container(
child: Column(
children: [
SizedBox(height: 50,),
CarouselSlider(
options: CarouselOptions(
height: 200.0,
autoPlay: true,
aspectRatio: 16 / 9,
enlargeCenterPage: true,
),
items: imageList.map((imageUrl) {
return Builder(
builder: (BuildContext context) {
return Container(
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.symmetric(horizontal: 10.0),
decoration: BoxDecoration(
color: Colors.grey,
),
child: Image.network(
imageUrl,
fit: BoxFit.cover,
),
);
},
);
}).toList(),
),
],
),
),
);
}
}
Output :
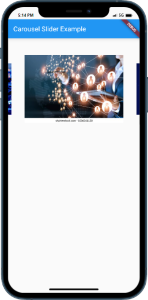