Introduction:
Toast messages are a common way to provide temporary, non-intrusive notifications or feedback to users in mobile apps. In Flutter, you can display toast messages to provide important information, success messages, error notifications, or other types of feedback. Toast messages typically appear as a small pop-up at the bottom of the screen and automatically disappear after a short duration. In this step-by-step guide, you will learn how to show toast messages in Flutter. By following this tutorial, you will be able to implement toast messages using the Fluttertoast package and customize their appearance and behavior.
Content:
1. Set up a new Flutter project:
Ensure that you have Flutter installed and set up on your machine. Create a new Flutter project using the following command in your terminal:
flutter create toast_app
2. Add the Fluttertoast package:
Open the pubspec.yaml
file in your Flutter project and add the fluttertoast
package as a dependency:
dependencies:
fluttertoast: ^8.0.8
Save the file and run the following command in your terminal to fetch the package:
flutter pub get
3. Import the Fluttertoast package:
In the Dart file where you want to show the toast message, import the fluttertoast
package:
import 'package:fluttertoast/fluttertoast.dart';
4. Show a toast message:
To display a toast message, call the Fluttertoast.showToast()
method with the desired parameters. Here’s an example:
Fluttertoast.showToast(
msg: 'This is a toast message',
toastLength: Toast.LENGTH_SHORT,
gravity: ToastGravity.BOTTOM,
backgroundColor: Colors.black.withOpacity(0.8),
textColor: Colors.white,
);
5. Customize the toast message:
You can customize the appearance and behavior of the toast message by specifying different parameters. For example, you can set the toastLength
to Toast.LENGTH_LONG
for a longer duration, modify the gravity
to position the toast at the top, center, or bottom of the screen, change the backgroundColor
and textColor
to match your app’s design, and more.
Sample Code:
import 'package:flutter/material.dart';
import 'package:fluttertoast/fluttertoast.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Toast Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Toast Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
showToast();
},
child: Text('Show Toast'),
),
),
);
}
void showToast() {
Fluttertoast.showToast(
msg: 'Hello this is a Toast! meassege,',
toastLength: Toast.LENGTH_SHORT,
gravity: ToastGravity.BOTTOM,
backgroundColor: Colors.black.withOpacity(0.8),
textColor: Colors.white,
);
}
}
Output:
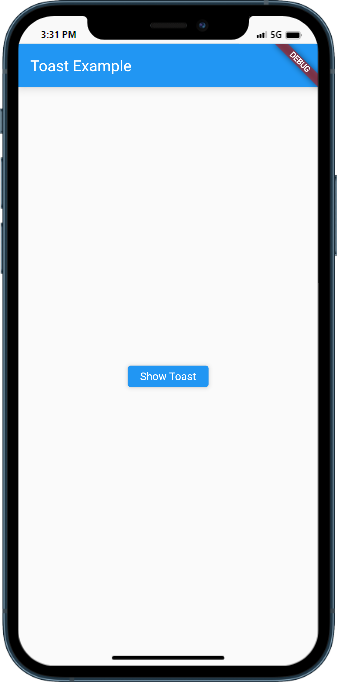
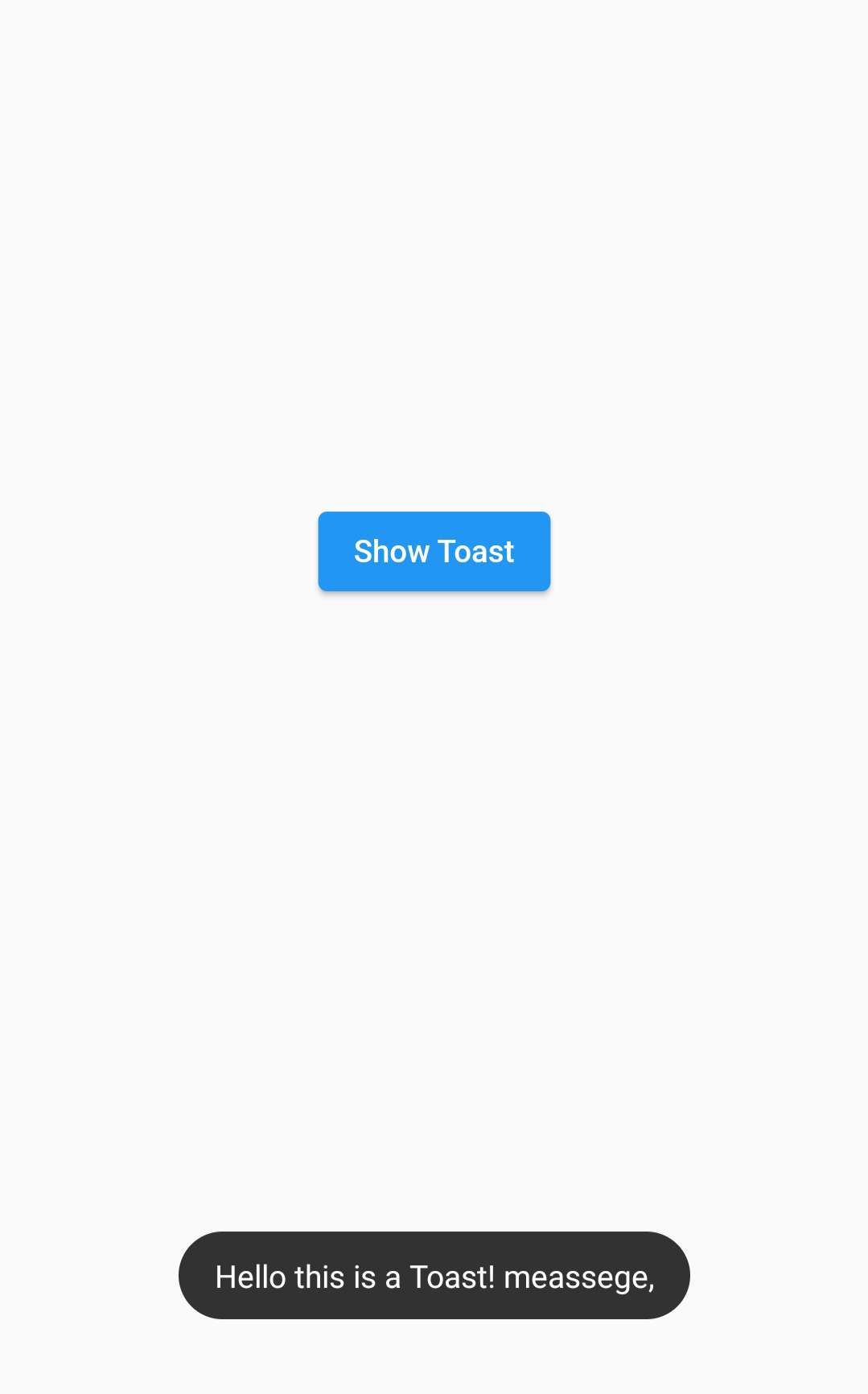
Conclusion:
Congratulations! You have successfully learned how to show toast messages in a Flutter app. By following this step-by-step guide, you can now implement toast messages using the Fluttertoast package and customize their appearance and behavior as per your app’s requirements. Toast messages are an effective way to provide temporary notifications or feedback to users in a non-intrusive manner. Utilize toast messages to enhance the user experience and provide important information or notifications in your Flutter app.