Are you looking to enhance the user experience in your Flutter app by adding a custom dialog box? Dialog boxes are a great way to display important information, prompt user actions, or provide additional context. In this tutorial, we’ll walk you through the process of creating a custom dialog box in Flutter, step by step.
Introduction
Dialog boxes are an essential component of any interactive app. While Flutter provides built-in dialog widgets, creating a custom dialog box allows you to tailor the design and functionality to your specific needs. By following the steps outlined in this tutorial, you’ll be able to create a custom dialog box that aligns with your app’s branding and provides a seamless user experience.
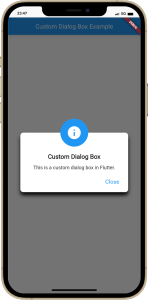
Prerequisites
Before we begin, make sure you have Flutter installed and set up on your development machine. You can follow the official Flutter documentation to get started if you haven’t already.
Step 1: Set up the Project
Start by creating a new Flutter project using the Flutter CLI or your preferred IDE. Open the project in your code editor and navigate to the lib
directory.
Step 2: Implement the CustomDialogBox Widget
Inside the lib
directory, create a new file called custom_dialog_box.dart
. This file will contain the code for our custom dialog box widget. Copy and paste the following code into the custom_dialog_box.dart
file:
import 'package:flutter/material.dart';
class CustomDialogBox extends StatelessWidget {
// TODO: Implement the custom dialog box widget
}
Step 3: Customize the Dialog Box
In the CustomDialogBox
class, we’ll define the structure and appearance of our custom dialog box. Let’s add the necessary code to create a visually appealing and user-friendly dialog box. Replace the // TODO
comment with the following code:
class CustomDialogBox extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Dialog(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
elevation: 0,
backgroundColor: Colors.transparent,
child: contentBox(context),
);
}
Widget contentBox(BuildContext context) {
return Stack(
// TODO: Implement the content of the dialog box
);
}
}
Step 4: Customize the Dialog Box Content
Inside the contentBox
method, we’ll define the content of our dialog box. This can include a title, description, images, buttons, or any other widget you desire. Replace the // TODO
comment with the following code:
Widget contentBox(BuildContext context) {
return Stack(
children: <Widget>[
Container(
padding: EdgeInsets.only(
left: 20,
top: 65,
right: 20,
bottom: 20,
),
margin: EdgeInsets.only(top: 45),
decoration: BoxDecoration(
shape: BoxShape.rectangle,
color: Colors.white,
borderRadius: BorderRadius.circular(10),
boxShadow: [
BoxShadow(
color: Colors.black,
offset: Offset(0, 10),
blurRadius: 10,
),
],
),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(
'Custom Dialog Box',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
),
),
SizedBox(height: 15),
Text(
'This is a custom dialog box in Flutter.',
style: TextStyle(fontSize: 16),
textAlign: TextAlign.center,
),
SizedBox(height: 22),
Align(
alignment: Alignment.bottomRight,
child: TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text(
'Close',
style: TextStyle(fontSize: 18),
),
),
),
],
),
),
Positioned(
top: 0,
left: 20,
right: 20,
child: CircleAvatar(
backgroundColor: Colors.blue,
radius: 45,
child: Icon(
Icons.info,
color: Colors.white,
size: 50,
),
),
),
],
);
}
Step 5: Show the Custom Dialog Box
To show the custom dialog box in your app, navigate to your app’s main page or any other page where you want to trigger the dialog box. Add a button or any other widget that triggers the dialog box when pressed. Replace the existing onPressed
event handler with the following code:
onPressed: () {
showDialog(
context: context,
builder: (BuildContext context) {
return CustomDialogBox();
},
);
},
Final code
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Custom Dialog Box',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Custom Dialog Box Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
showDialog(
context: context,
builder: (BuildContext context) {
return CustomDialogBox();
},
);
},
child: Text('Show Dialog'),
),
),
);
}
}
class CustomDialogBox extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Dialog(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
elevation: 0,
backgroundColor: Colors.transparent,
child: contentBox(context),
);
}
Widget contentBox(BuildContext context) {
return Stack(
children: <Widget>[
Container(
padding: EdgeInsets.only(
left: 20,
top: 65,
right: 20,
bottom: 20,
),
margin: EdgeInsets.only(top: 45),
decoration: BoxDecoration(
shape: BoxShape.rectangle,
color: Colors.white,
borderRadius: BorderRadius.circular(10),
boxShadow: [
BoxShadow(
color: Colors.black,
offset: Offset(0, 10),
blurRadius: 10,
),
],
),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(
'Custom Dialog Box',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.w600,
),
),
SizedBox(height: 15),
Text(
'This is a custom dialog box in Flutter.',
style: TextStyle(fontSize: 16),
textAlign: TextAlign.center,
),
SizedBox(height: 22),
Align(
alignment: Alignment.bottomRight,
child: TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text(
'Close',
style: TextStyle(fontSize: 18),
),
),
),
],
),
),
Positioned(
top: 0,
left: 20,
right: 20,
child: CircleAvatar(
backgroundColor: Colors.blue,
radius: 45,
child: Icon(
Icons.info,
color: Colors.white,
size: 50,
),
),
),
],
);
}
}
In the above code, the CustomDialogBox
class represents the custom dialog box widget. It extends StatelessWidget
and creates a Dialog
widget with a custom content box. The contentBox
method defines the content of the dialog, including the title, description, and a close button.
To use the custom dialog box, you can call showDialog
and pass an instance of the CustomDialogBox
widget as the builder
. This will display the dialog box on the screen.
You can customize the appearance and content of the dialog box by modifying the properties and widgets within the contentBox
method.
Remember to import the necessary packages, such as flutter/material.dart
, to use the required Flutter widgets and classes.
Output
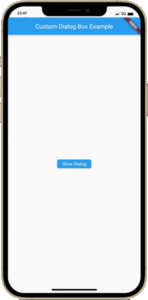
Conclusion
Congratulations! You’ve successfully created a custom dialog box in Flutter. By customizing the appearance and content of the dialog box, you can create unique and interactive experiences for your app users. Feel free to experiment with different designs and add additional functionality based on your specific requirements.
Remember to test your app thoroughly and gather user feedback to ensure the dialog box provides a seamless and intuitive user experience. Happy coding!