Introduction:
In Flutter, handling text input and performing validation is essential when building interactive forms and capturing user input. Whether it’s validating email addresses, passwords, or other user inputs, Flutter provides various mechanisms to handle text input and ensure data integrity.
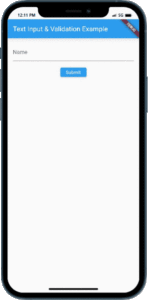
Content:
1. Create a new Flutter project:
Before we begin, make sure you have Flutter installed and set up on your machine. Create a new Flutter project by running the following command in your terminal:
flutter create text_input_example
2. Open the main.dart file:
Once the project is created, navigate to the lib
folder and open the main.dart
file.
3. Import the necessary packages:
To work with text input and validation, import the following packages at the top of the main.dart
file:
import 'package:flutter/material.dart';
4. Define the main function:
Inside the main.dart
file, locate the main()
function and modify it as follows:
void main() => runApp(MyApp());
5. Create a StatefulWidget:
Within the lib
folder, create a new file called input_form.dart
and define a new StatefulWidget
called InputForm
. This will serve as our form screen for capturing user input.
6. Implement the InputForm widget:
Inside the input_form.dart
file, define the InputForm
class as follows:
class InputForm extends StatefulWidget {
@override
_InputFormState createState() => _InputFormState();
}
7. Implement the state class:
Below the InputForm
class, define the _InputFormState
class, which extends State<InputForm>
. Inside this class, we will implement the layout and behavior of our form screen, including text input fields and validation.
a. Declare TextEditingController variables: Declare TextEditingController
variables to control the text input fields and handle user input. For example, if we have a name input field, declare a TextEditingController
as follows:
TextEditingController nameController = TextEditingController();
b. Implement the build()
method: Inside the _InputFormState
class, implement the build()
method, which will return the layout for our form screen. The layout will consist of a Scaffold with input fields and validation logic.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Input Form Example'),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
TextField(
controller: nameController,
decoration: InputDecoration(
labelText: 'Name',
),
),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: () {
// Perform validation and handle input
// Add your code here
},
child: Text('Submit'),
),
],
),
),
);
}
c. Implement validation logic: Inside the onPressed
callback of the submit button, you can perform validation and handle the user input. For example, you can check if the name field is empty or if the entered email address is valid. Add your validation and handling logic accordingly.
8. Integrate InputForm into the main.dart file:
Back in the main.dart
file, modify the MyApp
class to use InputForm
as the home screen:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Input Form Example',
home: InputForm(),
);
}
}
Sample Code :
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Text Input & Validation Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final _formKey = GlobalKey<FormState>();
late String _name;
void _submitForm() {
if (_formKey.currentState != null && _formKey.currentState!.validate()) {
_formKey.currentState!.save();
// Perform further actions, such as form submission or data processing
print('Name: $_name');
}
}
String? _validateName(String? value) {
if (value == null || value.isEmpty) {
return 'Please enter your name.';
}
return null;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Text Input & Validation Example'),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Name',
),
validator: _validateName,
onSaved: (value) => _name = value!,
),
SizedBox(height: 20),
ElevatedButton(
onPressed: _submitForm,
child: Text('Submit'),
),
],
),
),
),
);
}
}
Output:
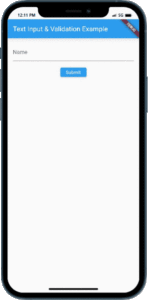
Conclusion:
Congratulations! You have successfully learned how to handle text input and perform validation in Flutter. You created a form screen with input fields, implemented validation logic, and captured user input. Utilize these techniques to build interactive forms and ensure data integrity in your Flutter applications.