Introduction
In Flutter, adding padding and margin to a widget is crucial for controlling the spacing and layout of your app’s user interface. Padding refers to the space inside a widget, while margin refers to the space outside a widget. This tutorial will walk you through the steps of adding padding and margin to a widget in Flutter.
Content:
1. add padding a widget in Flutter:
To add padding a widget in Flutter, follow these steps:
- Identify the Widget: Determine the widget to which you want to apply padding .
- Using Padding: Wrap the widget with a
Padding
widget and set thepadding
property to define the desired padding values. Thepadding
property accepts an instance of theEdgeInsets
class, allowing you to specify padding for each side (top, right, bottom, left).Example:
Container( padding: EdgeInsets.only( left: 50, right: 50, bottom: 50, top: 50), // Add padding to only wich sides you want color: Colors.purple, // Set container background color child: Container( child: Text( 'Contaienr with only left,right,bottom,top, side padding'), ), ),
- Using Container: Alternatively, you can utilize a
Container
widget to add padding. Wrap the widget with aContainer
and set thepadding
property based on your requirements.Example:
Container( padding: EdgeInsets.all(60), // Add padding to vertical sides color: Colors.blue, // Set container background color child: Container( child: Text('Contaienr with EdgeInsets.all(60), all side padding'), ), ),
- Adjust Values: Customize the padding and margin values to achieve the desired spacing and layout. Experiment with different values to achieve the desired visual appearance.
2. add margin a widget in Flutter:
To add margin a widget in Flutter, follow these steps:
- Identify the Widget: Determine the widget to which you want to apply margin .
- Using margin : Wrap the widget with a margin widget and set the margin property to define the desired margin values. The margin property accepts an instance of the
EdgeInsets
class, allowing you to specify margin for each side (top, right, bottom, left).Example:
Container( margin: EdgeInsets.only( left: 50, right: 50, bottom: 50, top: 50), // Add margin to only wich sides you want color: Colors.purple, // Set container background color child: Container( child: Text( 'Contaienr with only left,right,bottom,top, side margin '), ), ),
- Using Container: Alternatively, you can utilize a
Container
widget to add margin . Wrap the widget with aContainer
and set the margin property based on your requirements.Example:
Container( margin: EdgeInsets.all(60), // Add margin to vertical sides color: Colors.blue, // Set container background color child: Container( child: Text('Contaienr with EdgeInsets.all(60), all side margin '), ), ),
- Adjust Values: Customize the margin and margin values to achieve the desired spacing and layout. Experiment with different values to achieve the desired visual appearance.
Sample Code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Add Padding and Margin'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Padding",
style: TextStyle(
fontWeight: FontWeight.w700,
color: Colors.black,
fontSize: 22),
),
SizedBox(
height: 10,
),
//first method
Container(
padding: EdgeInsets.all(20), // Add margin to vertical sides
color: Colors.blue, // Set container background color
child: Container(
child:
Text('Contaienr with EdgeInsets.all(60), all side padding'),
),
),
SizedBox(
height: 20,
),
// Second method
Container(
padding: EdgeInsets.symmetric(
vertical: 40,
horizontal:
40), // Add margin to vertical and horizontal sides
color: Colors.red, // Set container background color
child: Container(
child: Text('Contaienr with vertical and horizontal padding'),
),
),
SizedBox(
height: 20,
),
// third method
Container(
padding: EdgeInsets.only(
left: 10,
right: 10,
bottom: 10,
top: 10), // Add margin to only wich sides you want
color: Color.fromARGB(
255, 117, 245, 119), // Set container background color
child: Container(
child: Text(
'Contaienr with only left,right,bottom,top, side padding'),
),
),
SizedBox(
height: 20,
),
Text(
"Margin",
style: TextStyle(
fontWeight: FontWeight.w700,
color: Colors.black,
fontSize: 22),
),
SizedBox(
height: 10,
),
Container(
margin: EdgeInsets.all(20), // Add margin to vertical sides
color: Colors.blue, // Set container background color
child: Container(
child:
Text('Contaienr with EdgeInsets.all(60), all side Margin'),
),
),
SizedBox(
height: 20,
),
// Second method
Container(
margin: EdgeInsets.symmetric(
vertical: 40,
horizontal:
40), // Add margin to vertical and horizontal sides
color: Colors.red, // Set container background color
child: Container(
child: Text('Contaienr with vertical and horizontal Margin'),
),
),
SizedBox(
height: 20,
),
// third method
Container(
margin: EdgeInsets.only(
left: 10,
right: 10,
bottom: 10,
top: 10), // Add margin to only wich sides you want
color: Color.fromARGB(
255, 117, 245, 119), // Set container background color
child: Container(
child: Text(
'Contaienr with only left,right,bottom,top, side Margin'),
),
),
],
),
),
);
}
}
Output:
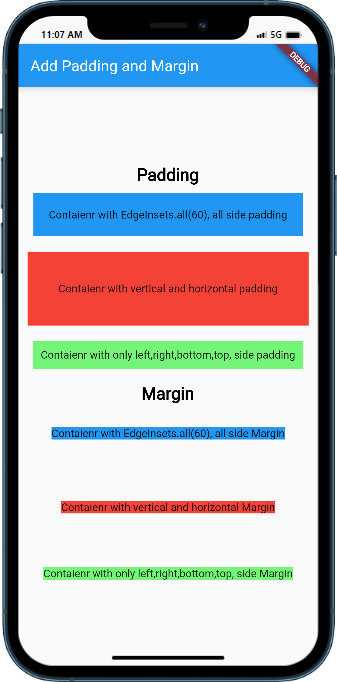
Conclusion:
By using the Padding
widget or the Container
widget with appropriate properties, you can easily add padding and margin to a widget in Flutter. Remember that padding affects the space inside the widget, while margin affects the space outside the widget. Explore different values to achieve the desired spacing and layout in your app’s UI.