User input is a fundamental aspect of app development, enabling users to interact with your Flutter app. Flutter provides a variety of widgets and mechanisms to capture user input, such as text fields, buttons, and gestures. In this comprehensive guide, we will explore how to implement basic interactivity in your Flutter app by handling user input and updating the app’s state dynamically.
Prerequisites
Before we get started, ensure that you have the following prerequisites:
- Flutter SDK installed on your machine. If you haven’t installed Flutter yet, refer to the official Flutter installation guide for your operating system.
- A Flutter project set up and ready for development.
Handling Text Input
Capturing text input from users is a common requirement in many apps. Flutter provides the TextField
widget to handle text input. Let’s see an example of how to implement a basic text input field:
TextField(
onChanged: (value) {
// Handle the text input change here
},
);
By specifying an onChanged
callback, you can listen to changes in the text input and perform actions accordingly.
Responding to Button Presses
Buttons allow users to trigger specific actions within your app. Flutter offers various button widgets, including ElevatedButton
, TextButton
, and IconButton
. Here’s an example of how to implement a button that responds to user presses:
ElevatedButton(
onPressed: () {
// Handle button press here
},
child: Text('Submit'),
);
The onPressed
callback is executed when the button is pressed, allowing you to perform the desired actions.
Handling Gestures
Gestures enable users to interact with your app through touch inputs, such as tapping, swiping, and dragging. Flutter provides gesture recognition widgets, such as GestureDetector
, to handle various gestures. Let’s explore an example of implementing a tap gesture:
GestureDetector(
onTap: () {
// Handle tap gesture here
},
child: Container(
width: 200,
height: 200,
color: Colors.blue,
child: Center(
child: Text('Tap Me'),
),
),
);
In this example, the onTap
callback is triggered when the user taps on the Container
widget, allowing you to respond to the tap gesture accordingly.
Updating the App State
To create dynamic and interactive experiences, you need to update the app’s state in response to user input. Flutter follows a reactive programming model, where the app’s UI reflects the current state. To update the app’s state, you can use a state management solution like setState
or a dedicated state management library like Provider, Riverpod, or Bloc.
Here’s an example of using setState
to update a counter value when a button is pressed:
class CounterApp extends StatefulWidget {
@override
_CounterAppState createState() => _CounterAppState();
}
class _CounterAppState extends State<CounterApp> {
int _counter = 0;
@override
Widget build(BuildContext context) {
return Column(
children: [
Text('Counter: $_counter'),
ElevatedButton(
onPressed: () {
setState(() {
_counter++;
});
},
child: Text('Increment'),
),
],
);
}
}
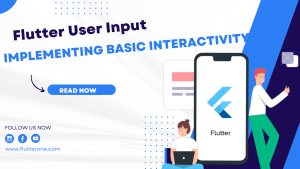
In this example, the _counter
variable represents the app’s state. The onPressed
callback of the button triggers a call to setState
, updating the state and triggering a rebuild of the UI.
Conclusion
Implementing basic interactivity with user input is crucial for creating engaging and dynamic Flutter apps. By leveraging Flutter’s widgets and mechanisms for capturing user input, such as text fields, buttons, and gestures, and updating the app’s state, you can create interactive experiences that respond to user actions.
Experiment with different input widgets, handle various user interactions, and utilize proper state management techniques to build robust and interactive apps with Flutter. With these techniques, you can create user-friendly experiences and empower your users to interact seamlessly with your app. Happy coding and exploring the world of user input in Flutter!