Introduction:
Storing data locally is a common requirement in mobile app development. In Flutter, you can use the Shared Preferences package to easily store and retrieve key-value pairs locally on the device. This tutorial will guide you through the process of implementing local data storage using Shared Preferences in Flutter.
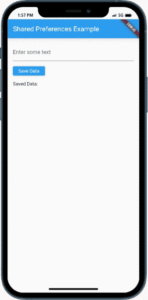
Content:
1. Create a new Flutter project:
Before we begin, make sure you have Flutter installed and set up on your machine. Create a new Flutter project by running the following command in your terminal:
flutter create shared_preferences_example
2. Open the pubspec.yaml file:
Once the project is created, navigate to the root directory of your project and open the pubspec.yaml
file.
3. Add the shared_preferences dependency:
In the pubspec.yaml
file, add the following line under the dependencies
section:
dependencies:
shared_preferences: ^2.0.12
Save the file and run flutter pub get
in your terminal to fetch the package.
4. Import the necessary packages:
To work with Shared Preferences, import the following packages at the top of the main.dart
file:
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
5. Implement the data storage logic:
Inside the main.dart
file, define a SharedPreferences
instance and implement the logic to store and retrieve data using Shared Preferences.
a. Initialize SharedPreferences: Create a method called initSharedPreferences()
to initialize the SharedPreferences
instance:
Future<void> initSharedPreferences() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
// Save the instance to a global variable for easy access
sharedPreferences = prefs;
}
Call this method at the beginning of the main()
function:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await initSharedPreferences();
runApp(MyApp());
}
b. Store data using Shared Preferences: Create a method called storeData()
to store data using Shared Preferences. For example, let’s store a user’s name:
void storeData(String name) {
sharedPreferences.setString('name', name);
}
c. Retrieve data using Shared Preferences: Create a method called retrieveData()
to retrieve data using Shared Preferences. For example, let’s retrieve the stored user name:
String retrieveData() {
return sharedPreferences.getString('name') ?? '';
}
6. Use the data storage logic in your app:
In your app, use the storeData()
and retrieveData()
methods to store and retrieve data respectively. For example, you can update the app’s UI based on the stored user name.
7. Run the app:
Save the changes and run the app using the following command in your terminal:
flutter run
Sample Code:
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
final TextEditingController _textController = TextEditingController();
String _savedData = '';
@override
void initState() {
super.initState();
loadData();
}
void saveData() async {
final SharedPreferences prefs = await SharedPreferences.getInstance();
await prefs.setString('textKey', _textController.text);
setState(() {
_savedData = _textController.text;
});
_textController.clear();
}
void loadData() async {
final SharedPreferences prefs = await SharedPreferences.getInstance();
setState(() {
_savedData = prefs.getString('textKey') ?? '';
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Shared Preferences Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text('Shared Preferences Example'),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
TextField(
controller: _textController,
decoration: InputDecoration(
labelText: 'Enter some text',
),
),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: saveData,
child: Text('Save Data'),
),
SizedBox(height: 16.0),
Text('Saved Data: $_savedData'),
],
),
),
),
);
}
}
Output:
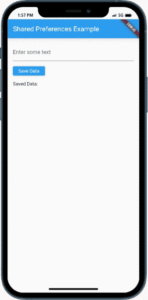
Conclusion:
Congratulations! You have successfully implemented local data storage using Shared Preferences in Flutter. By using the Shared Preferences package, you can easily store and retrieve key-value pairs locally on the device, enabling you to save and retrieve user preferences, settings, and other persistent data in your Flutter app. Start utilizing Shared Preferences to enhance the