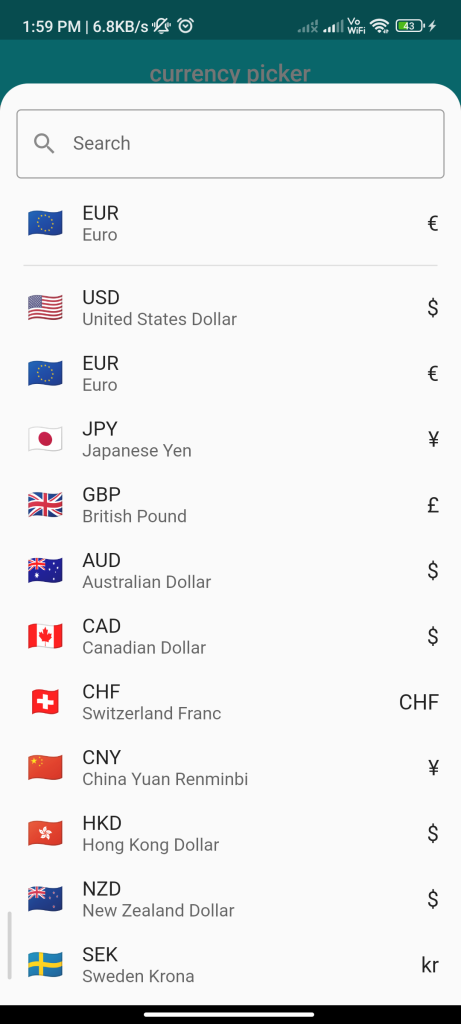
Introduction
The currency_picker
package allows you to create a currency picker in your Flutter app, enabling users to select a currency from a list. This guide will demonstrate how to use this package to implement a currency picker in your app.
Content
1.Add the currency_picker
dependency:
Open your pubspec.yaml
file and add the currency_picker
dependency.
dependencies:
currency_picker: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the currency_picker
package in your Dart file.
import 'package:currency_picker/currency_picker.dart';
3.Use the showCurrencyPicker
method:
Use the showCurrencyPicker
method to display the currency picker dialog.
void showCurrencyPicker(BuildContext context) {
showCurrencyList(
context: context,
onSelect: (Currency currency) {
print('Selected currency: ${currency.currencyCode}');
},
);
}
Call this method to show the currency picker dialog. The onSelect
callback will be called when a currency is selected.
4.Customize the currency picker:
You can customize the currency picker dialog by providing additional parameters to the showCurrencyList
method.
void showCurrencyPicker(BuildContext context) {
showCurrencyList(
context: context,
showFlag: true,
showCurrencyName: true,
showCurrencyCode: true,
onSelect: (Currency currency) {
print('Selected currency: ${currency.currencyCode}');
},
);
}
Set the showFlag
, showCurrencyName
, and showCurrencyCode
parameters to true
to show the flag, currency name, and currency code, respectively.
Sample Code
import 'package:currency_picker/currency_picker.dart';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Demo for currency picker package',
theme: ThemeData.light(),
darkTheme: ThemeData.light(),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
Currency? _selectedCurrency;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Color.fromARGB(255, 19, 222, 230),
centerTitle: true,
title: const Text('currency picker'),
),
body: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Center(
child: ElevatedButton(
onPressed: () {
showCurrencyPicker(
context: context,
showFlag: true,
showSearchField: true,
showCurrencyName: true,
showCurrencyCode: true,
favorite: ['eur'],
onSelect: (Currency currency) {
print('Select currency: ${currency.name}');
print('Select currency: ${currency.code}');
print('Select currency: ${currency.symbol}');
setState(() {
_selectedCurrency = currency;
});
},
);
},
child: const Text('Show currency picker'),
),
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Selected Currency name-:',
style: TextStyle(fontSize: 16, color: Colors.black),
),
_selectedCurrency != null
? Text(
'${_selectedCurrency!.name}',
style: TextStyle(fontSize: 16, color: Colors.blue),
)
: Container(),
],
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Selected Currency code-:',
style: TextStyle(fontSize: 16, color: Colors.black),
),
_selectedCurrency != null
? Text(
'${_selectedCurrency!.code}',
style: TextStyle(fontSize: 16, color: Colors.blue),
)
: Container(),
],
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Selected Currency symbol-:',
style: TextStyle(fontSize: 16, color: Colors.black),
),
_selectedCurrency != null
? Text(
'${_selectedCurrency!.symbol}',
style: TextStyle(fontSize: 16, color: Colors.blue),
)
: Container(),
],
),
],
),
);
}
}
Output
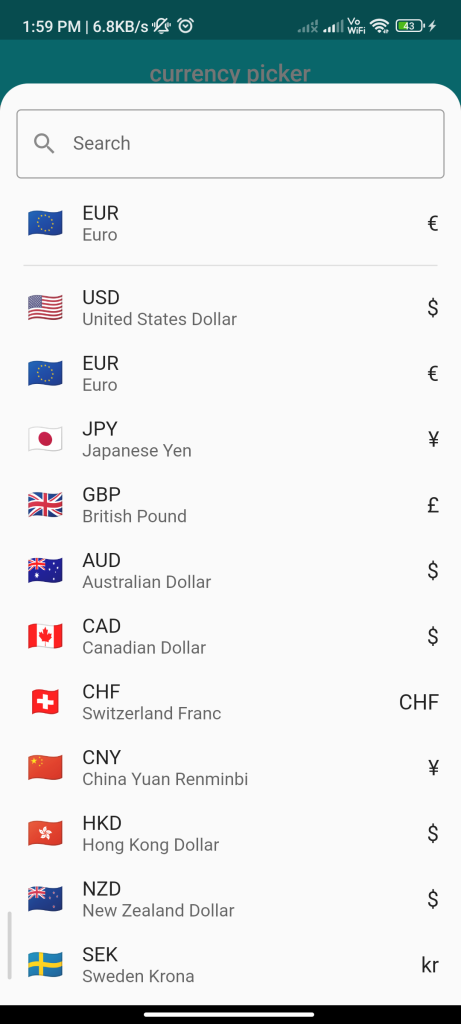
Conclusion
By following these steps, you can implement a currency picker in your Flutter app using the currency_picker
package. This allows you to provide users with a convenient way to select a currency for their transactions or preferences.