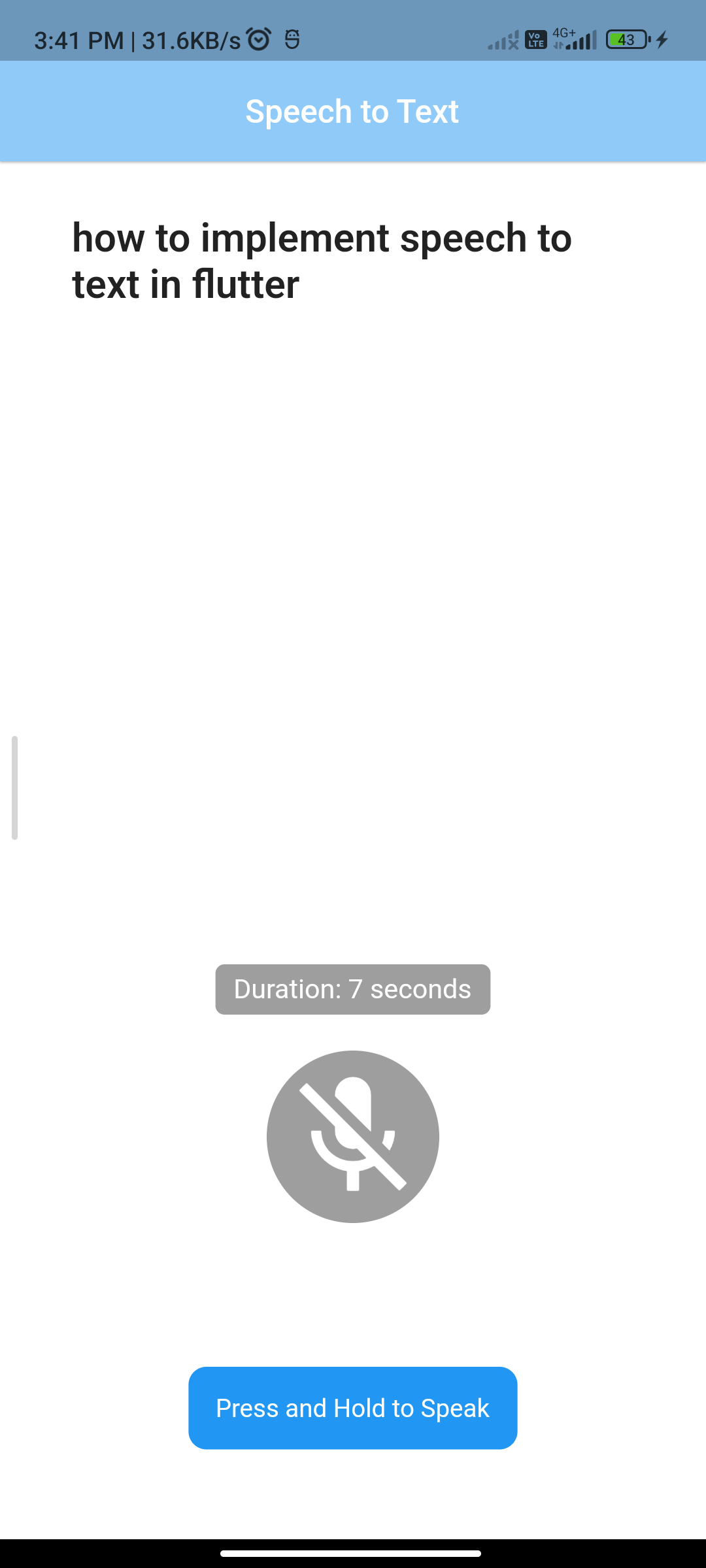
Introduction
Implementing speech-to-text functionality in a Flutter app enables users to input text using their voice. In this guide, we’ll explore how to integrate speech-to-text in Flutter using the ‘speech_to_text’ package.
Content
Step 1: Create a New Flutter Project
Ensure that you have Flutter installed and set up on your machine. Create a new Flutter project using the following command in your terminal:
flutter create speech_to_text_app
Step 2: Add the ‘speech_to_text’ Dependency
Open the pubspec.yaml
file in your Flutter project and add the ‘speech_to_text’ dependency:
dependencies:
speech_to_text: ^5.2.0
Save the file and run flutter pub get
in your terminal.
Step 3: Add the required permission in the AndroidManifest.xml
Path:–android/app/main/AndroidManifest.xml
<uses-permission android:name="android.permission.RECORD_AUDIO" />
Step 4: Implement Speech to Text in Flutter
Open the lib/main.dart
file and replace its content with the following code:
import 'package:flutter/material.dart';
import 'package:speech_to_text/speech_to_text.dart' as stt;
void main() {
runApp(SpeechToTextApp());
}
class SpeechToTextApp extends StatefulWidget {
@override
_SpeechToTextAppState createState() => _SpeechToTextAppState();
}
class _SpeechToTextAppState extends State<SpeechToTextApp> {
final stt.SpeechToText _speech = stt.SpeechToText();
String _text = 'Press the button and start speaking...';
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Speech to Text Example"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(_text),
SizedBox(height: 20),
ElevatedButton(
onPressed: _listen,
child: Text("Start Listening"),
),
],
),
),
),
);
}
void _listen() async {
if (!_speech.isListening) {
bool available = await _speech.initialize(
onStatus: (status) {
print('Status: $status');
},
onError: (errorNotification) {
print('Error: $errorNotification');
},
);
if (available) {
_speech.listen(
onResult: (result) {
setState(() {
_text = result.recognizedWords;
});
},
);
}
} else {
_speech.stop();
}
}
}
Step 5: Run the App
Save your changes and run the app using the following command in your terminal:
flutter run
Sample Code:
// ignore_for_file: prefer_const_constructors
import 'dart:async';
import 'dart:ffi';
import 'package:flutter/material.dart';
import 'package:speech_to_text/speech_to_text.dart' as stt;
import 'package:speech_to_text/speech_to_text.dart';
class SpeechToText extends StatefulWidget {
@override
_SpeechToTextState createState() => _SpeechToTextState();
}
class _SpeechToTextState extends State<SpeechToText> {
stt.SpeechToText _speech = stt.SpeechToText();
bool _isListening = false;
String _text = '';
int _duration = 0;
late Timer _timer;
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
centerTitle: true,
elevation: 1,
title: Text(
'Speech to Text',
style: TextStyle(fontSize: 18, color: Colors.white),
),
backgroundColor: Colors.blue[200],
),
body: Container(
padding: EdgeInsets.all(20.0),
child: Column(
children: [
Expanded(
child: SingleChildScrollView(
child: Center(
child: Container(
width: double.infinity,
margin: EdgeInsets.symmetric(horizontal: 10),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(10), color: Colors.white60),
padding: EdgeInsets.symmetric(horizontal: 10, vertical: 10),
child: Text(
_text,
style: TextStyle(
fontSize: 22.0,
fontWeight: FontWeight.w600,
),
),
),
),
),
),
Column(
children: [
Center(
child: Container(
padding: EdgeInsets.symmetric(horizontal: 10, vertical: 5),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(5), color: Colors.grey),
child: Text(
'Duration: $_duration seconds',
style: TextStyle(fontSize: 15.0, color: Colors.white),
),
),
),
SizedBox(
height: 20,
),
GestureDetector(
onLongPress: () {
_startListening();
},
onLongPressEnd: (details) {
_stopListening();
},
child: Column(
children: [
Ink(
decoration: const ShapeDecoration(
color: Colors.grey,
shape: CircleBorder(),
),
child: IconButton(
color: _isListening ? Colors.green : Colors.white,
icon: _isListening ? Icon(Icons.mic) : Icon(Icons.mic_off),
iconSize: 80,
onPressed: () {
_isListening ? _stopListening() : _startListening();
},
)),
SizedBox(
height: 80,
),
Container(
decoration: BoxDecoration(color: _isListening ? Colors.green : Colors.blue, borderRadius: BorderRadius.circular(10)),
padding: EdgeInsets.all(15.0),
child: Text(
_isListening ? 'Listening...' : 'Press and Hold to Speak',
style: TextStyle(color: Colors.white),
),
),
],
),
),
SizedBox(
height: 30,
),
],
)
],
),
),
);
}
void _startListening() async {
bool available = await _speech.initialize(
onStatus: (status) {
if (_speech.isListening) {
_startTimer();
} else {
_stopTimer();
}
},
onError: (errorNotification) {
print('error: $errorNotification');
},
);
if (available) {
setState(() {
_isListening = true;
_duration = 0; // Reset the duration when starting a new session
});
_speech.listen(
onResult: (result) {
setState(() {
_text = result.recognizedWords;
});
},
);
}
}
void _stopListening() {
setState(() {
_isListening = false;
_stopTimer();
_speech.stop();
});
}
void _startTimer() {
_timer = Timer.periodic(Duration(seconds: 1), (Timer timer) {
setState(() {
_duration++;
});
});
}
void _stopTimer() {
if (_timer.isActive) {
_timer.cancel(); // Stop the timer if it's active
}
}
@override
void dispose() {
_stopTimer(); // Stop the timer when the widget is disposed
super.dispose();
}
}
Output:
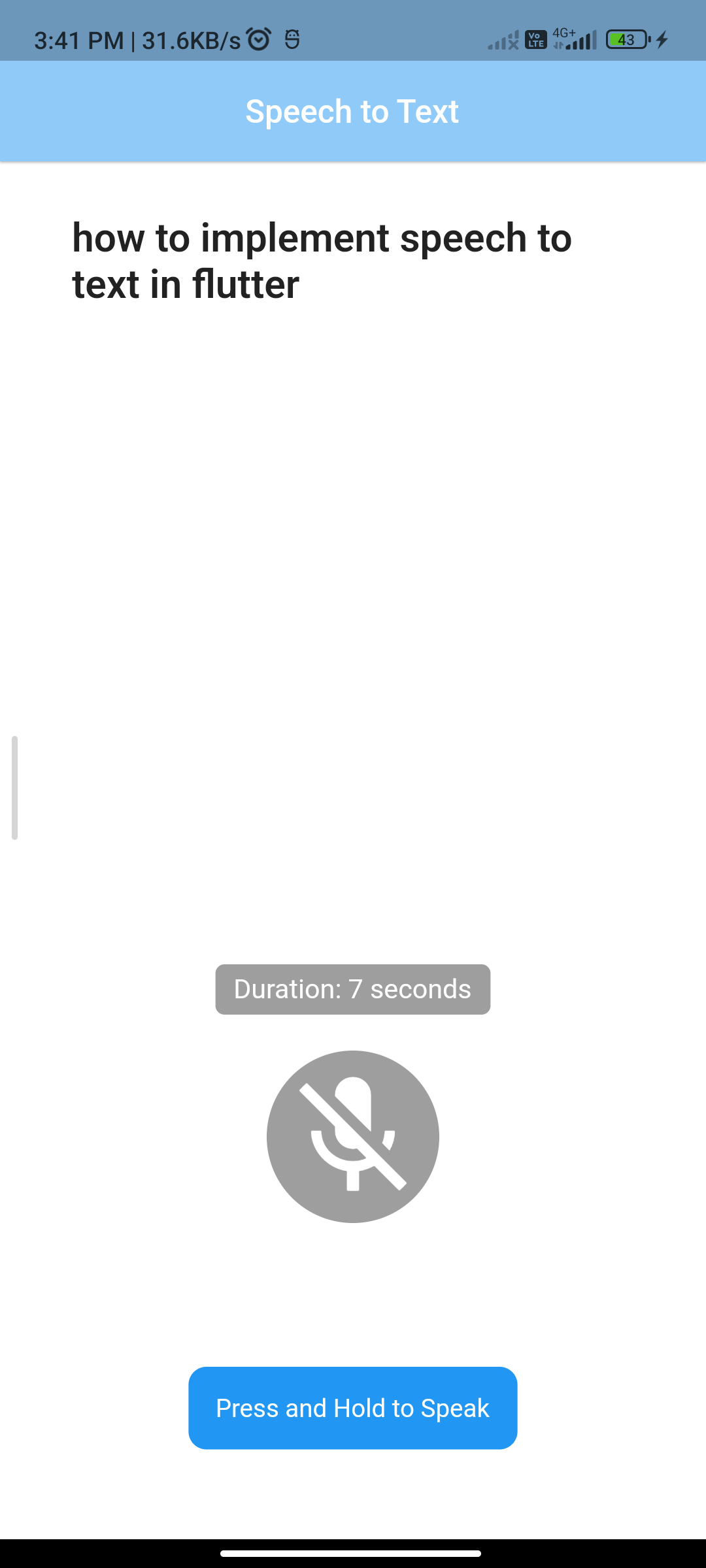
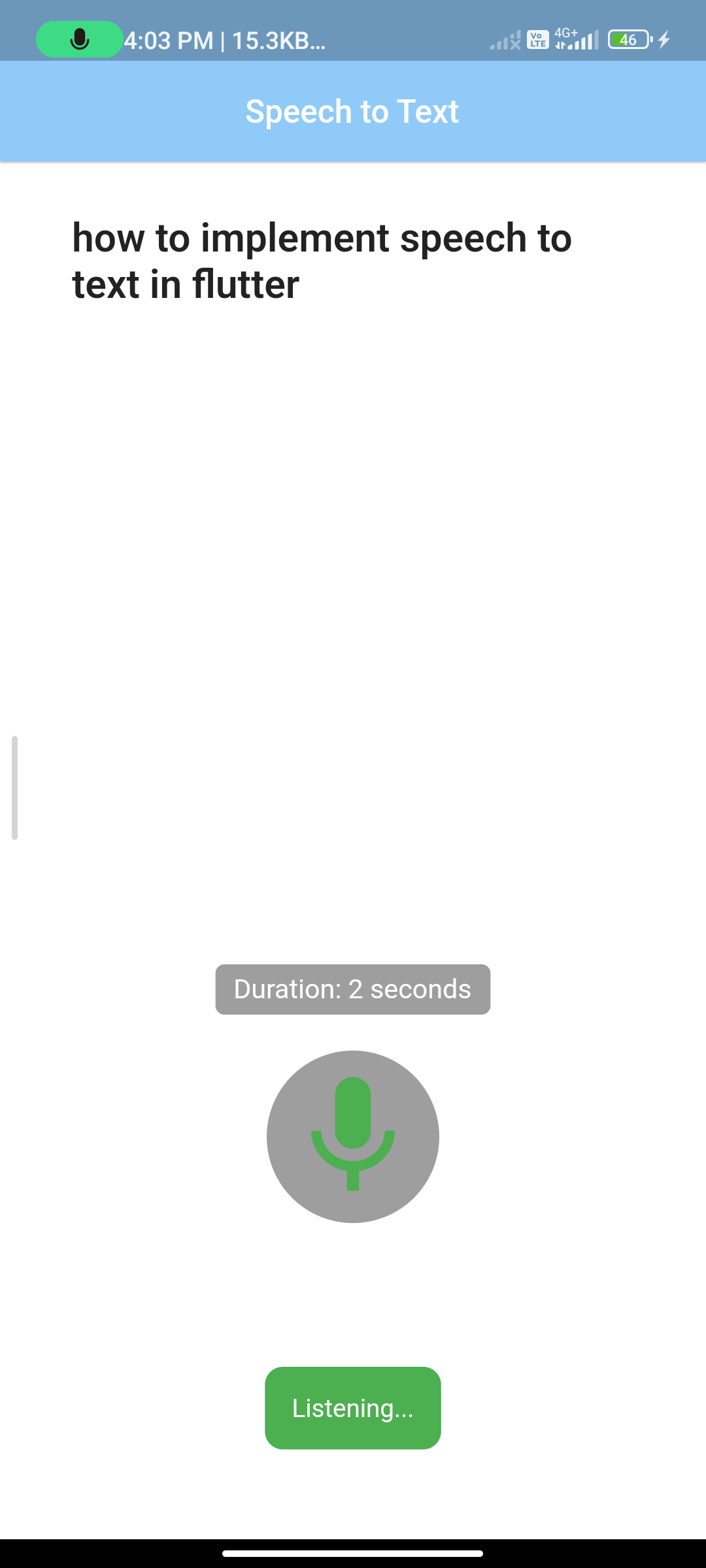
Conclusion:
In this guide, we’ve demonstrated how to implement speech-to-text functionality in a Flutter app using the ‘speech_to_text’ package. The example app provides a button to start listening, and the recognized speech is displayed on the screen. You can further enhance and customize the user interface and integrate speech-to-text into specific features of your Flutter application.