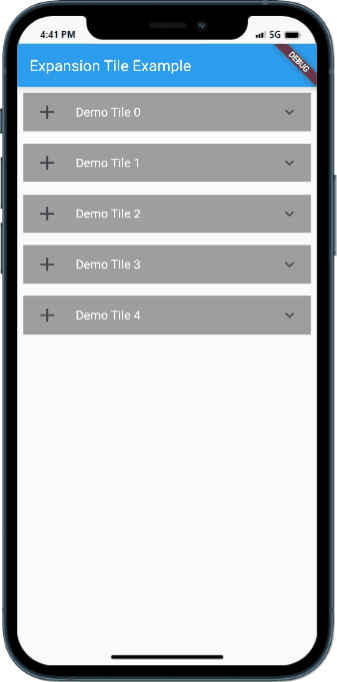
Introduction
The ExpansionTile widget in Flutter allows you to create expandable and collapsible list items, providing a convenient way to display hierarchical information. With ExpansionTile, users can expand or collapse a tile to reveal or hide additional content. In this tutorial, you will learn how to implement an ExpansionTile in your Flutter app.
Content
To implement an ExpansionTile in Flutter, follow these steps:
Step 1.Import Packages
Import the necessary packages in your Dart file:
import 'package:flutter/material.dart';
Step 2.Build ExpansionTile
Create an ExpansionTile widget within your Flutter widget tree. Customize the properties of the ExpansionTile based on your requirements. The key properties to set are title
, children
, initiallyExpanded
, and onExpansionChanged
.
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('ExpansionTile Example'),
),
body: ListView(
children: <Widget>[
ExpansionTile(
title: Text('Tile Title'),
children: <Widget>[
// Add your child widgets here
Text('Child Widget 1'),
Text('Child Widget 2'),
],
initiallyExpanded: false,
onExpansionChanged: (expanded) {
// Handle expansion state change
},
),
],
),
);
}
Customize the title
property to display the main title of the tile. Add child widgets within the children
property to include any additional content that should be revealed when the tile is expanded. Adjust the initiallyExpanded
property to control the initial state of the tile, whether expanded or collapsed. Handle the onExpansionChanged
callback to perform any actions when the tile’s expansion state changes.
Step 3.Add Desired UI Elements
Enhance your ExpansionTile by adding any desired UI elements inside the children
property. You can include images, text, buttons, or any other widgets to provide rich and interactive content within the expanded view of the tile.
Sample Code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Expansion Tile Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Expansion Tile Example'),
),
body: ListView.builder(
itemCount: 5,
itemBuilder: (context, index) {
return Padding(
padding: EdgeInsets.all(8.0),
child: MyExpansionTile(title: 'Demo Tile $index'),
);
},
),
);
}
}
class MyExpansionTile extends StatefulWidget {
final String title;
const MyExpansionTile({required this.title});
@override
_MyExpansionTileState createState() => _MyExpansionTileState();
}
class _MyExpansionTileState extends State<MyExpansionTile> {
bool _isExpanded = false;
@override
Widget build(BuildContext context) {
return ExpansionTile(
collapsedBackgroundColor: Colors.grey,
leading: Icon(
Icons.add,
size: 30,
),
collapsedTextColor: Colors.white,
backgroundColor: Color.fromARGB(255, 255, 254, 169),
title: Text(widget.title),
children: [
Container(
child: Text(
'hello larner?',
style: TextStyle(
color: Colors.black,
fontSize: 14,
fontWeight: FontWeight.w700,
),
),
),
SizedBox(
height: 20,
),
Container(
height: 200,
width: 300,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(10),
image: DecorationImage(
image: NetworkImage(
"https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTgCkN7PS2ctbBt5j_R-A2eDuYdkmkO0M7avhgolvEn0jDHm_-OLVwS-KcvEkwUsWhOVAA&usqp=CAU"),
fit: BoxFit.fill)),
)
],
onExpansionChanged: (value) {
setState(() {
_isExpanded = value;
});
},
);
}
}
Output:
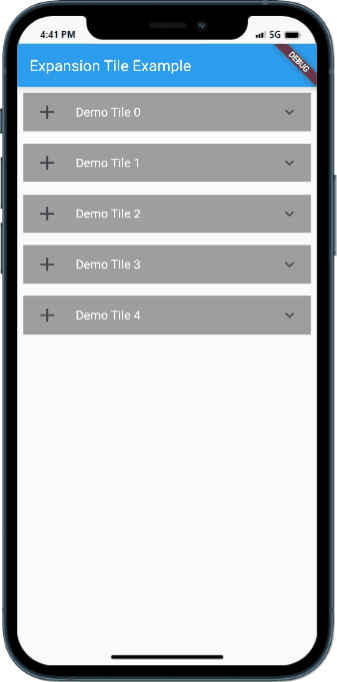
Conclusion:
By following these steps, you can easily implement an ExpansionTile in Flutter to create expandable and collapsible list items. Use the ExpansionTile widget to display hierarchical information and provide a user-friendly interactive experience in your Flutter app.