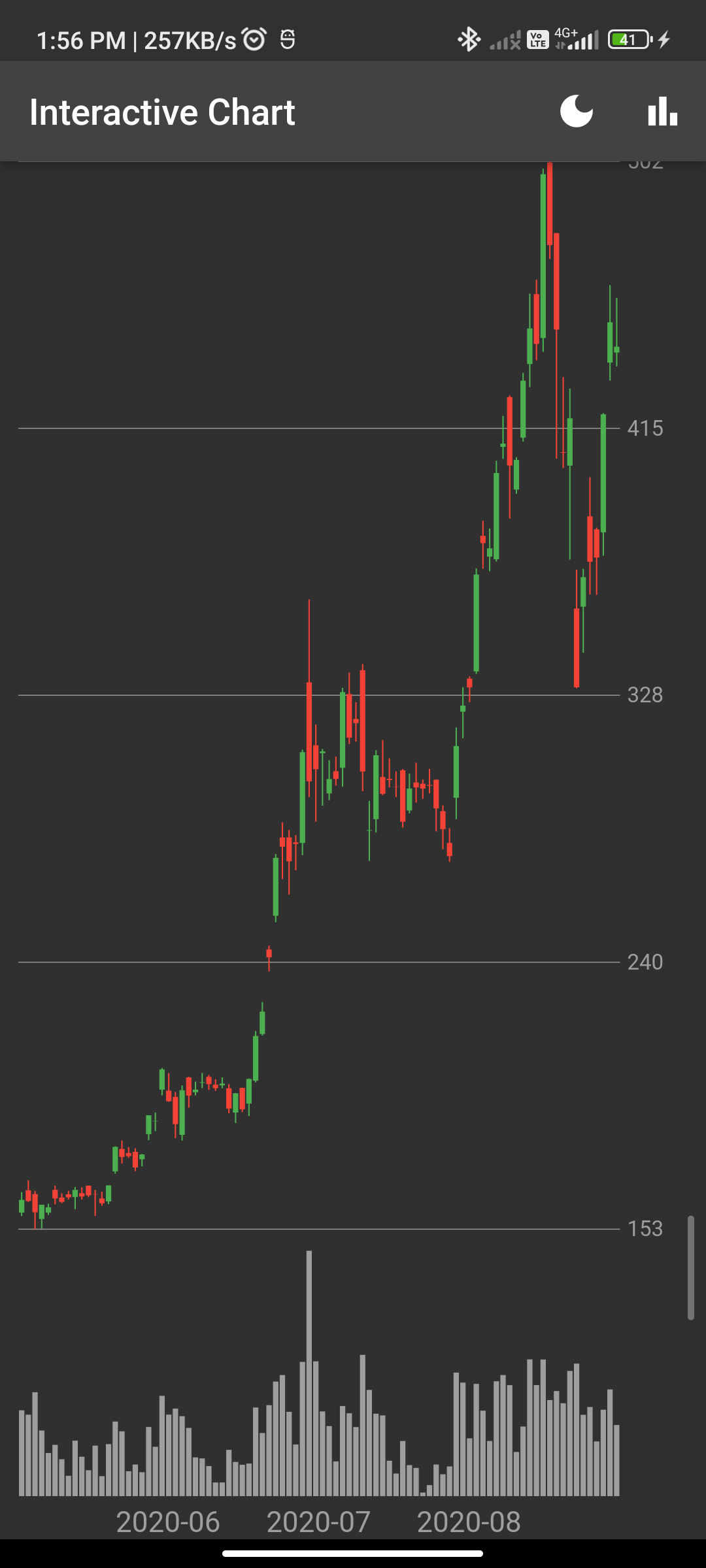
Introduction:
Spider charts, also known as radar charts, are effective visualizations for displaying multivariate data points. In Flutter, you can implement a Spider Interactive Chart using the `interactive_chart` package. This step-by-step guide will help you integrate and customize a spider interactive chart in your Flutter app.
Content:
Step 1: Add Dependency Ensure you have the `interactive_chart` package added to your `pubspec.yaml` file.
Run the following command in your terminal:
interactive_chart: ^2.0.0
Run flutter pub get
to fetch the package.
Step 2: Import Dependencies
In your Dart file, import the necessary packages:
import 'package:flutter/material.dart';
import 'package:interactive_chart/interactive_chart.dart';
Step 3: Create Data Model
Define a data model for the chart items:
class ChartItem {
final String label;
final double value;
ChartItem(this.label, this.value);
}
Step 4: Implement Spider Interactive Chart
Use the following Dart code as an example for implementing the spider interactive chart:
void main() {
runApp(SpiderInteractiveChartApp());
}
class SpiderInteractiveChartApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
List<ChartItem> data = [
ChartItem('Math', 80),
ChartItem('Science', 90),
ChartItem('Art', 70),
ChartItem('History', 85),
ChartItem('Geography', 75),
ChartItem('Physical Education', 95),
];
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Spider Interactive Chart Example'),
),
body: Center(
child: InteractiveChart(
data: data,
maxValue: 100,
initialAngle: 0,
interactive: true,
showLabels: true,
strokeWidth: 2.5,
color: Colors.blue,
width: 300,
height: 300,
),
),
),
);
}
}
Sample Code:
import 'package:flutter/material.dart';
import 'package:interactive_chart/interactive_chart.dart';
// import 'mock_data.dart';
class InterectiveChart extends StatefulWidget {
@override
_InterectiveChartState createState() => _InterectiveChartState();
}
class _InterectiveChartState extends State<InterectiveChart> {
List<CandleData> _data = MockDataTesla.candles;
bool _darkMode = true;
bool _showAverage = false;
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
theme: ThemeData(
brightness: _darkMode ? Brightness.dark : Brightness.light,
),
home: Scaffold(
appBar: AppBar(
title: Text("Interactive Chart"),
actions: [
IconButton(
icon: Icon(_darkMode ? Icons.dark_mode : Icons.light_mode),
onPressed: () => setState(() => _darkMode = !_darkMode),
),
IconButton(
icon: Icon(
_showAverage ? Icons.show_chart : Icons.bar_chart_outlined,
),
onPressed: () {
setState(() => _showAverage = !_showAverage);
if (_showAverage) {
_computeTrendLines();
} else {
_removeTrendLines();
}
},
),
],
),
body: SafeArea(
minimum: const EdgeInsets.only(left: 10, right: 0),
child: InteractiveChart(
/** Only [candles] is required */
candles: _data,
/** Uncomment the following for examples on optional parameters */
/** Example styling */
// style: ChartStyle(
// priceGainColor: Colors.teal[200]!,
// priceLossColor: Colors.blueGrey,
// volumeColor: Colors.teal.withOpacity(0.8),
// trendLineStyles: [
// Paint()
// ..strokeWidth = 2.0
// ..strokeCap = StrokeCap.round
// ..color = Colors.deepOrange,
// Paint()
// ..strokeWidth = 4.0
// ..strokeCap = StrokeCap.round
// ..color = Colors.orange,
// ],
// priceGridLineColor: Colors.red[200]!,
// priceLabelStyle: TextStyle(color: Colors.blue[200]),
// timeLabelStyle: TextStyle(color: Colors.blue[200]),
// selectionHighlightColor: Colors.red.withOpacity(0.2),
// overlayBackgroundColor: Colors.red[900]!.withOpacity(0.6),
// overlayTextStyle: TextStyle(color: Colors.red[100]),
// timeLabelHeight: 32,
// volumeHeightFactor: 0.2, // volume area is 20% of total height
// ),
/** Customize axis labels */
// timeLabel: (timestamp, visibleDataCount) => "📅",
priceLabel: (price) => "${price.round()} ",
/** Customize overlay (tap and hold to see it)
** Or return an empty object to disable overlay info. */
// overlayInfo: (candle) => {
// "💎": "🤚 ",
// "Hi": "${candle.high?.toStringAsFixed(2)}",
// "Lo": "${candle.low?.toStringAsFixed(2)}",
// },
/** Callbacks */
onTap: (candle) => print("user tapped on $candle"),
onCandleResize: (width) => print("each candle is $width wide"),
),
),
),
);
}
_computeTrendLines() {
final ma7 = CandleData.computeMA(_data, 7);
final ma30 = CandleData.computeMA(_data, 30);
final ma90 = CandleData.computeMA(_data, 90);
for (int i = 0; i < _data.length; i++) {
_data[i].trends = [ma7[i], ma30[i], ma90[i]];
}
}
_removeTrendLines() {
for (final data in _data) {
data.trends = [];
}
}
}
class MockDataTesla {
static const List<dynamic> _rawData = [
// (Price data for Tesla Inc, taken from Yahoo Finance)
// timestamp, open, high, low, close, volume
[1555939800, 53.80, 53.94, 52.50, 52.55, 60735500],
[1556026200, 52.03, 53.12, 51.15, 52.78, 54719500],
[1556112600, 52.77, 53.06, 51.60, 51.73, 53637500],
[1556199000, 51.00, 51.80, 49.21, 49.53, 109247000],
[1556285400, 49.30, 49.34, 46.23, 47.03, 111803500],
[1556544600, 47.17, 48.80, 46.43, 48.29, 83572500],
[1556631000, 48.41, 48.84, 47.40, 47.74, 47323000],
[1556717400, 47.77, 48.00, 46.30, 46.80, 53522000],
[1556803800, 49.10, 49.43, 47.54, 48.82, 90796500],
[1556890200, 48.77, 51.32, 48.70, 51.01, 118534000],
[1557149400, 50.00, 51.67, 49.70, 51.07, 54169500],
[1559223000, 37.75, 38.45, 37.40, 37.64, 39632500],
[1559309400, 37.02, 37.98, 36.82, 37.03, 52033500],
[1559568600, 37.10, 37.34, 35.40, 35.79, 65322000],
[1559655000, 36.22, 38.80, 35.92, 38.72, 69037500],
[1559741400, 39.74, 40.26, 38.37, 39.32, 67554000],
[1559827800, 40.89, 42.20, 40.36, 41.19, 101211000],
[1559914200, 41.00, 42.17, 40.70, 40.90, 80017500],
[1560173400, 42.05, 43.39, 41.80, 42.58, 52925000],
[1560259800, 43.83, 44.18, 42.70, 43.42, 58267500],
[1560346200, 44.59, 44.68, 41.80, 41.85, 75987500],
[1560432600, 42.08, 42.98, 41.50, 42.78, 40841500],
[1560519000, 42.25, 43.33, 42.08, 42.98, 37167000],
[1560778200, 43.10, 45.40, 42.85, 45.01, 61584000],
[1560864600, 45.74, 46.95, 44.51, 44.95, 63579000],
[1560951000, 45.02, 45.55, 44.21, 45.29, 32875500],
[1561037400, 44.60, 45.38, 43.27, 43.92, 59317500],
[1561123800, 43.24, 44.44, 43.10, 44.37, 41010500],
[1561383000, 44.65, 45.17, 44.20, 44.73, 28754000],
[1561469400, 44.88, 45.07, 43.90, 43.95, 30910500],
[1561555800, 44.06, 45.45, 43.62, 43.85, 42536000],
[1561642200, 43.89, 44.58, 43.47, 44.57, 31698500],
[1561728600, 44.20, 45.03, 44.16, 44.69, 34257000],
[1561987800, 46.04, 46.62, 45.26, 45.43, 41067000],
[1562074200, 45.78, 45.83, 44.44, 44.91, 46295000],
[1562160600, 47.88, 48.31, 46.90, 46.98, 71005500],
[1562333400, 46.91, 47.09, 46.16, 46.62, 35328500],
[1562592600, 46.25, 46.45, 45.73, 46.07, 29402500],
[1562679000, 45.79, 46.20, 45.46, 46.01, 30954000],
[1562765400, 46.83, 47.79, 46.63, 47.78, 45728500],
[1562851800, 47.63, 48.30, 47.16, 47.72, 37572000],
[1562938200, 47.95, 49.08, 47.94, 49.02, 46002500],
[1563197400, 49.60, 50.88, 48.97, 50.70, 55000500],
[1563283800, 49.86, 50.71, 49.59, 50.48, 40745000],
[1563370200, 51.13, 51.66, 50.67, 50.97, 48823500],
[1563456600, 51.01, 51.15, 50.38, 50.71, 23793000],
[1563543000, 51.14, 51.99, 50.92, 51.64, 35242000],
[1563802200, 51.75, 52.43, 50.84, 51.14, 34212000],
[1563888600, 51.34, 52.10, 50.90, 52.03, 25115500],
[1563975000, 51.83, 53.21, 51.63, 52.98, 55364000],
[1564061400, 46.70, 46.90, 45.11, 45.76, 112091500],
[1564147800, 45.38, 46.05, 44.45, 45.61, 50138500],
[1564407000, 45.42, 47.19, 45.21, 47.15, 46366500],
[1564493400, 46.58, 48.67, 46.44, 48.45, 40545000],
[1564579800, 48.60, 49.34, 47.33, 48.32, 45891000],
[1564666200, 48.53, 48.90, 46.35, 46.77, 41297500],
[1564752600, 46.27, 47.25, 45.85, 46.87, 30682500],
[1565011800, 45.92, 46.27, 45.16, 45.66, 35141500],
[1565098200, 46.38, 46.50, 45.15, 46.15, 27821000],
[1565184600, 45.30, 46.71, 45.16, 46.68, 23882500],
[1565271000, 46.89, 47.96, 46.53, 47.66, 26371500],
[1565357400, 47.21, 47.79, 46.76, 47.00, 19491000],
[1565616600, 46.60, 47.15, 45.75, 45.80, 23319500],
[1565703000, 45.76, 47.20, 45.51, 47.00, 24240500],
[1565789400, 46.24, 46.30, 43.34, 43.92, 47813000],
[1565875800, 44.17, 44.31, 42.31, 43.13, 40798000],
[1565962200, 43.33, 44.45, 43.20, 43.99, 25492500],
[1566221400, 44.84, 45.57, 44.34, 45.37, 26548000],
[1566307800, 45.52, 45.82, 44.91, 45.17, 20626000],
[1566394200, 44.40, 44.64, 43.52, 44.17, 38971500],
[1566480600, 44.56, 45.08, 43.64, 44.43, 32795000],
[1566567000, 43.99, 44.23, 42.20, 42.28, 42693000],
[1566826200, 42.72, 43.00, 42.31, 43.00, 25259500],
[1566912600, 43.15, 43.76, 42.41, 42.82, 27081000],
[1566999000, 42.74, 43.45, 42.46, 43.12, 16127500],
[1567085400, 43.80, 44.68, 43.60, 44.34, 25897500],
[1567171800, 45.83, 46.49, 44.84, 45.12, 46603000],
[1567517400, 44.82, 45.79, 44.63, 45.00, 26770500],
[1567603800, 45.38, 45.69, 43.84, 44.14, 28805000],
[1567690200, 44.50, 45.96, 44.17, 45.92, 36976500],
[1567776600, 45.44, 45.93, 45.03, 45.49, 20947000],
[1568035800, 46.00, 46.75, 45.85, 46.36, 24013500],
[1568122200, 46.16, 47.11, 45.79, 47.11, 24418500],
[1568208600, 47.48, 49.63, 47.20, 49.42, 50214000],
[1568295000, 49.54, 50.70, 48.88, 49.17, 42906000],
[1568381400, 49.39, 49.69, 48.97, 49.04, 26565500],
[1568640600, 49.20, 49.49, 48.23, 48.56, 23640500],
[1568727000, 48.49, 49.12, 48.07, 48.96, 19327000],
[1568813400, 49.00, 49.63, 48.47, 48.70, 20851000],
[1568899800, 49.20, 49.59, 48.97, 49.32, 23979000],
[1568986200, 49.30, 49.39, 47.63, 48.12, 31765000],
[1569245400, 48.00, 49.04, 47.84, 48.25, 21701000],
[1569331800, 48.30, 48.40, 44.52, 44.64, 64457500],
[1569418200, 44.91, 45.80, 43.67, 45.74, 47135500],
[1569504600, 46.13, 48.66, 45.48, 48.51, 59422500],
[1569591000, 48.44, 49.74, 47.75, 48.43, 55582000],
[1569850200, 48.60, 48.80, 47.22, 48.17, 29399000],
[1569936600, 48.30, 49.19, 47.83, 48.94, 30813000],
[1570023000, 48.66, 48.93, 47.89, 48.63, 28157000],
[1570109400, 46.37, 46.90, 44.86, 46.61, 75422500],
[1570195800, 46.32, 46.96, 45.61, 46.29, 39975000],
[1570455000, 45.96, 47.71, 45.71, 47.54, 40321000],
[1570541400, 47.17, 48.79, 46.90, 48.01, 43391000],
[1570627800, 48.26, 49.46, 48.13, 48.91, 34472000],
[1570714200, 49.06, 49.86, 48.32, 48.95, 31416500],
[1570800600, 49.43, 50.22, 49.36, 49.58, 42377000],
[1571059800, 49.58, 51.71, 49.43, 51.39, 51025000],
[1571146200, 51.54, 52.00, 50.82, 51.58, 32164000],
[1571232600, 51.48, 52.42, 51.38, 51.95, 33420500],
[1571319000, 52.50, 52.96, 52.03, 52.39, 23846500],
[1571405400, 52.14, 52.56, 51.02, 51.39, 28749000],
[1571664600, 51.67, 51.90, 50.04, 50.70, 25101500],
[1571751000, 50.86, 51.67, 50.17, 51.12, 23004000],
[1571837400, 50.90, 51.23, 50.27, 50.94, 26305500],
[1571923800, 59.67, 60.99, 57.84, 59.94, 148604500],
[1572010200, 59.54, 66.00, 59.22, 65.63, 150030500],
[1572269400, 65.51, 68.17, 64.52, 65.54, 94351500],
[1572355800, 64.00, 64.86, 62.95, 63.24, 63421500],
[1572442200, 62.60, 63.76, 61.99, 63.00, 48209000],
[1572528600, 62.62, 63.80, 62.60, 62.98, 25335000],
[1572615000, 63.26, 63.30, 61.96, 62.66, 31919500],
[1572877800, 62.96, 64.39, 61.85, 63.49, 43935000],
[1572964200, 63.92, 64.70, 63.22, 63.44, 34717000],
[1573050600, 63.60, 65.34, 62.90, 65.32, 39704500],
[1573137000, 65.83, 68.30, 65.60, 67.11, 72336500],
[1573223400, 66.90, 67.49, 66.50, 67.43, 30346000],
[1573482600, 68.79, 69.84, 68.40, 69.02, 49933500],
[1573569000, 69.38, 70.07, 68.81, 69.99, 36797000],
[1573655400, 71.00, 71.27, 69.04, 69.22, 42100500],
[1573741800, 69.22, 70.77, 68.58, 69.87, 32324500],
[1573828200, 70.13, 70.56, 69.67, 70.43, 24045000],
[1574087400, 70.58, 70.63, 69.22, 70.00, 22002000],
[1574173800, 70.35, 72.00, 69.56, 71.90, 38624000],
[1574260200, 72.00, 72.24, 69.91, 70.44, 33625500],
[1574346600, 70.90, 72.17, 70.80, 70.97, 30550000],
[1574433000, 68.03, 68.20, 66.00, 66.61, 84353000],
[1574692200, 68.86, 68.91, 66.89, 67.27, 61697500],
[1574778600, 67.05, 67.10, 65.42, 65.78, 39737000],
[1574865000, 66.22, 66.79, 65.71, 66.26, 27778000],
[1575037800, 66.22, 66.25, 65.50, 65.99, 12328000],
[1575297000, 65.88, 67.28, 65.74, 66.97, 30372500],
[1575383400, 66.52, 67.58, 66.44, 67.24, 32868500],
[1580999400, 139.98, 159.17, 137.40, 149.79, 199404000],
[1581085800, 146.11, 153.95, 146.00, 149.61, 85317500],
[1581345000, 160.00, 164.00, 150.48, 154.26, 123446000],
[1581431400, 153.76, 156.70, 151.60, 154.88, 58487500],
[1581517800, 155.57, 157.95, 152.67, 153.46, 60112500],
[1581604200, 148.37, 163.60, 147.00, 160.80, 131446500],
[1581690600, 157.44, 162.59, 157.10, 160.01, 78468500],
[1582036200, 168.32, 172.00, 166.47, 171.68, 81908500],
[1582122600, 184.70, 188.96, 180.20, 183.48, 127115000],
[1582209000, 182.39, 182.40, 171.99, 179.88, 88174500],
[1582295400, 181.40, 182.61, 176.09, 180.20, 71574000],
[1582554600, 167.80, 172.70, 164.44, 166.76, 75961000],
[1582641000, 169.80, 171.32, 157.40, 159.98, 86452500],
[1582727400, 156.50, 162.66, 155.22, 155.76, 70427500],
[1582813800, 146.00, 147.95, 133.80, 135.80, 121386000],
[1582900200, 125.94, 138.10, 122.30, 133.60, 121114500],
[1583159400, 142.25, 148.74, 137.33, 148.72, 100975000],
[1583245800, 161.00, 161.40, 143.22, 149.10, 128920000],
[1583332200, 152.79, 153.30, 144.95, 149.90, 75245000],
[1583418600, 144.75, 149.15, 143.61, 144.91, 54263500],
[1583505000, 138.00, 141.40, 136.85, 140.70, 63314500],
[1583760600, 121.08, 132.60, 121.00, 121.60, 85368500],
[1583847000, 131.89, 133.60, 121.60, 129.07, 77972000],
[1583933400, 128.04, 130.72, 122.60, 126.85, 66612500],
[1584019800, 116.18, 118.90, 109.25, 112.11, 94545500],
[1584106200, 119.00, 121.51, 100.40, 109.32, 113201500],
[1584365400, 93.90, 98.97, 88.43, 89.01, 102447500],
[1584451800, 88.00, 94.37, 79.20, 86.04, 119973000],
[1584538200, 77.80, 80.97, 70.10, 72.24, 118931000],
[1584624600, 74.94, 90.40, 71.69, 85.53, 150977500],
[1584711000, 87.64, 95.40, 85.16, 85.51, 141427500],
[1584970200, 86.72, 88.40, 82.10, 86.86, 82272500],
[1585056600, 95.46, 102.74, 94.80, 101.00, 114476000],
[1585143000, 109.05, 111.40, 102.22, 107.85, 106113500],
[1585229400, 109.48, 112.00, 102.45, 105.63, 86903500],
[1585315800, 101.00, 105.16, 98.81, 102.87, 71887000],
[1585575000, 102.05, 103.33, 98.25, 100.43, 59990500],
[1585661400, 100.25, 108.59, 99.40, 104.80, 88857500],
[1585747800, 100.80, 102.79, 95.02, 96.31, 66766000],
[1585834200, 96.21, 98.85, 89.28, 90.89, 99292000],
[1585920600, 101.90, 103.10, 93.68, 96.00, 112810500],
[1586179800, 102.24, 104.20, 99.59, 103.25, 74509000],
[1586266200, 109.00, 113.00, 106.47, 109.09, 89599000],
[1586352600, 110.84, 111.44, 106.67, 109.77, 63280000],
[1586439000, 112.42, 115.04, 111.42, 114.60, 68250000],
[1586784600, 118.03, 130.40, 116.11, 130.19, 112377000],
[1586871000, 139.79, 148.38, 138.49, 141.98, 152882500],
[1586957400, 148.40, 150.63, 142.00, 145.97, 117885000],
[1587043800, 143.39, 151.89, 141.34, 149.04, 103289500],
[1587130200, 154.46, 154.99, 149.53, 150.78, 65641000],
[1587389400, 146.54, 153.11, 142.44, 149.27, 73733000],
[1587475800, 146.02, 150.67, 134.76, 137.34, 101045500],
[1587562200, 140.80, 146.80, 137.74, 146.42, 70827500],
[1587648600, 145.52, 146.80, 140.63, 141.13, 66183500],
[1587735000, 142.16, 146.15, 139.64, 145.03, 66060000],
[1587994200, 147.52, 159.90, 147.00, 159.75, 103407000],
[1588080600, 159.13, 161.00, 151.34, 153.82, 76110000],
[1588167000, 158.03, 160.64, 156.63, 160.10, 81080000],
[1588253400, 171.04, 173.96, 152.70, 156.38, 142359500],
[1588339800, 151.00, 154.55, 136.61, 140.26, 162659000],
[1588599000, 140.20, 152.40, 139.60, 152.24, 96185500],
[1588685400, 157.96, 159.78, 152.44, 153.64, 84958500],
[1588771800, 155.30, 157.96, 152.22, 156.52, 55616000],
[1588858200, 155.44, 159.28, 154.47, 156.01, 57638500],
[1588944600, 158.75, 164.80, 157.40, 163.88, 80432500],
[1589203800, 158.10, 164.80, 157.00, 162.26, 82598000],
[1589290200, 165.40, 168.66, 161.60, 161.88, 79534500],
[1589376600, 164.17, 165.20, 152.66, 158.19, 95327500],
[1589463000, 156.00, 160.67, 152.80, 160.67, 68411000],
[1589549400, 158.07, 161.01, 157.31, 159.83, 52592000],
[1589808600, 165.56, 166.94, 160.78, 162.73, 58329000],
[1589895000, 163.03, 164.41, 161.22, 161.60, 48182500],
[1589981400, 164.10, 165.20, 162.36, 163.11, 36546500],
[1590067800, 163.20, 166.50, 159.20, 165.52, 61273000],
[1590154200, 164.43, 166.36, 162.40, 163.38, 49937500],
[1590499800, 166.90, 166.92, 163.14, 163.77, 40448500],
[1590586200, 164.17, 165.54, 157.00, 164.05, 57747500],
[1590672600, 162.70, 164.95, 160.34, 161.16, 36278000],
[1590759000, 161.75, 167.00, 160.84, 167.00, 58822500],
[1591018200, 171.60, 179.80, 170.82, 179.62, 74697500],
[1591104600, 178.94, 181.73, 174.20, 176.31, 67828000],
[1591191000, 177.62, 179.59, 176.02, 176.59, 39747500],
[1591277400, 177.98, 179.15, 171.69, 172.88, 44438500],
[1591363800, 175.57, 177.30, 173.24, 177.13, 39059500],
[1591623000, 183.80, 190.00, 181.83, 189.98, 70873500],
[1591709400, 188.00, 190.89, 184.79, 188.13, 56941000],
[1591795800, 198.38, 205.50, 196.50, 205.01, 92817000],
[1591882200, 198.04, 203.79, 194.40, 194.57, 79582500],
[1591968600, 196.00, 197.60, 182.52, 187.06, 83817000],
[1592227800, 183.56, 199.77, 181.70, 198.18, 78486000],
[1592314200, 202.37, 202.58, 192.48, 196.43, 70255500],
[1592400600, 197.54, 201.00, 196.51, 198.36, 49454000],
[1592487000, 200.60, 203.84, 198.89, 200.79, 48759500],
[1592573400, 202.56, 203.19, 198.27, 200.18, 43398500],
[1592832600, 199.99, 201.78, 198.00, 198.86, 31812000],
[1592919000, 199.78, 202.40, 198.80, 200.36, 31826500],
[1593005400, 198.82, 200.18, 190.63, 192.17, 54798000],
[1593091800, 190.85, 197.20, 187.43, 197.20, 46272500],
[1593178200, 198.96, 199.00, 190.97, 191.95, 44274500],
[1593437400, 193.80, 202.00, 189.70, 201.87, 45132000],
[1593523800, 201.30, 217.54, 200.75, 215.96, 84592500],
[1593610200, 216.60, 227.07, 216.10, 223.93, 66634500],
[1593696600, 244.30, 245.60, 237.12, 241.73, 86250500],
[1594042200, 255.34, 275.56, 253.21, 274.32, 102849500],
[1594128600, 281.00, 285.90, 267.34, 277.97, 107448500],
[1594215000, 281.00, 283.45, 262.27, 273.18, 81556500],
[1594301400, 279.40, 281.71, 270.26, 278.86, 58588000],
[1594387800, 279.20, 309.78, 275.20, 308.93, 116688000],
[1594647000, 331.80, 359.00, 294.22, 299.41, 194927000],
[1594733400, 311.20, 318.00, 286.20, 303.36, 117090500],
[1594819800, 308.60, 310.00, 291.40, 309.20, 81839000],
[1594906200, 295.43, 306.34, 293.20, 300.13, 71504000],
[1594992600, 302.69, 307.50, 298.00, 300.17, 46650000],
[1595251800, 303.80, 330.00, 297.60, 328.60, 85607000],
[1595338200, 327.99, 335.00, 311.60, 313.67, 80536000],
[1595424600, 319.80, 325.28, 312.40, 318.47, 70805500],
[1595511000, 335.79, 337.80, 296.15, 302.61, 121642500],
[1595597400, 283.20, 293.00, 273.31, 283.40, 96983000],
[1595856600, 287.00, 309.59, 282.60, 307.92, 80243500],
[1595943000, 300.80, 312.94, 294.88, 295.30, 79043500],
[1596029400, 300.20, 306.96, 297.40, 299.82, 47134500],
[1596115800, 297.60, 302.65, 294.20, 297.50, 38105000],
[1596202200, 303.00, 303.41, 284.20, 286.15, 61041000],
[1596461400, 289.84, 301.96, 288.88, 297.00, 44046500],
[1596547800, 299.00, 305.48, 292.40, 297.40, 42075000],
[1596634200, 298.60, 299.97, 293.66, 297.00, 24739000],
[1596720600, 298.17, 303.46, 295.45, 297.92, 29961500],
[1596807000, 299.91, 299.95, 283.00, 290.54, 44482000],
[1597066200, 289.60, 291.50, 277.17, 283.71, 37611500],
[1597152600, 279.20, 284.00, 273.00, 274.88, 43129000],
[1597239000, 294.00, 317.00, 287.00, 310.95, 109147000],
[1597325400, 322.20, 330.24, 313.45, 324.20, 102126500],
[1597411800, 333.00, 333.76, 325.33, 330.14, 62888000],
[1597671000, 335.40, 369.17, 334.57, 367.13, 101211500],
[1597757400, 379.80, 384.78, 369.02, 377.42, 82372500],
[1597843800, 373.00, 382.20, 368.24, 375.71, 61026500],
[1597930200, 372.14, 404.40, 371.41, 400.37, 103059000],
[1598016600, 408.95, 419.10, 405.01, 410.00, 107448000],
[1598275800, 425.26, 425.80, 385.50, 402.84, 100318000],
[1598362200, 394.98, 405.59, 393.60, 404.67, 53294500],
[1598448600, 412.00, 433.20, 410.73, 430.63, 71197000],
[1598535000, 436.09, 459.12, 428.50, 447.75, 118465000],
[1598621400, 459.02, 463.70, 437.30, 442.68, 100406000],
[1598880600, 444.61, 500.14, 440.11, 498.32, 118374400],
[1598967000, 502.14, 502.49, 470.51, 475.05, 89841100],
[1599053400, 478.99, 479.04, 405.12, 447.37, 96176100],
[1599139800, 407.23, 431.80, 402.00, 407.00, 87596100],
[1599226200, 402.81, 428.00, 372.02, 418.32, 110321900],
[1599571800, 356.00, 368.74, 329.88, 330.21, 115465700],
[1599658200, 356.60, 369.00, 341.51, 366.28, 79465800],
[1599744600, 386.21, 398.99, 360.56, 371.34, 84930600],
[1599831000, 381.94, 382.50, 360.50, 372.72, 60717500],
[1600090200, 380.95, 420.00, 373.30, 419.62, 83020600],
[1600176600, 436.56, 461.94, 430.70, 449.76, 97298200],
[1600263000, 439.87, 457.79, 435.31, 441.76, 72279300],
];
static List<CandleData> get candles => _rawData
.map((row) => CandleData(
timestamp: row[0] * 1000,
open: row[1]?.toDouble(),
high: row[2]?.toDouble(),
low: row[3]?.toDouble(),
close: row[4]?.toDouble(),
volume: row[5]?.toDouble(),
))
.toList();
}
Output:
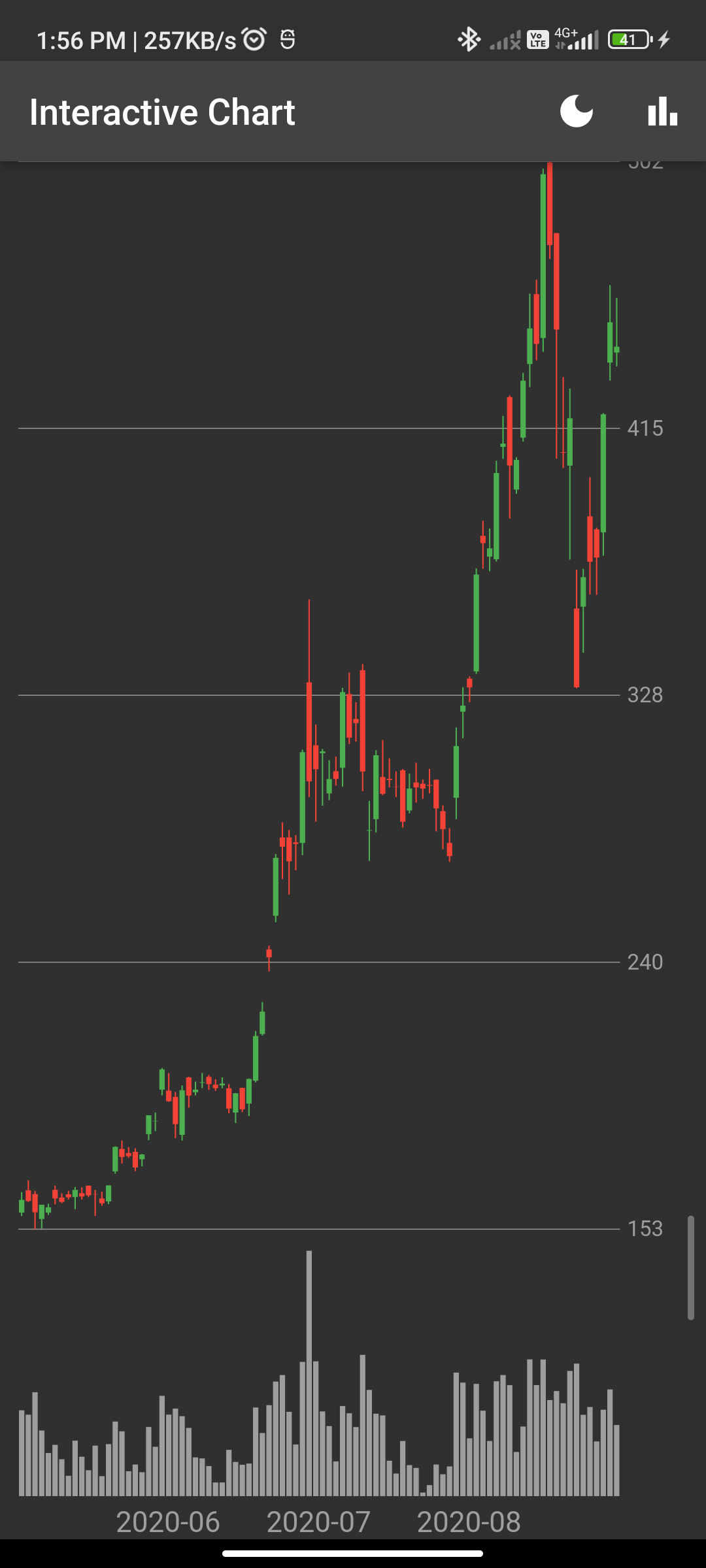
Conclusion:
Congratulations! You’ve successfully implemented a Spider Interactive Chart in your Flutter app using the interactive_chart
package. Customize the chart’s appearance and behavior as needed for your specific requirements.
Feel free to experiment and explore additional features provided by the interactive_chart
package to enhance your data visualization capabilities.