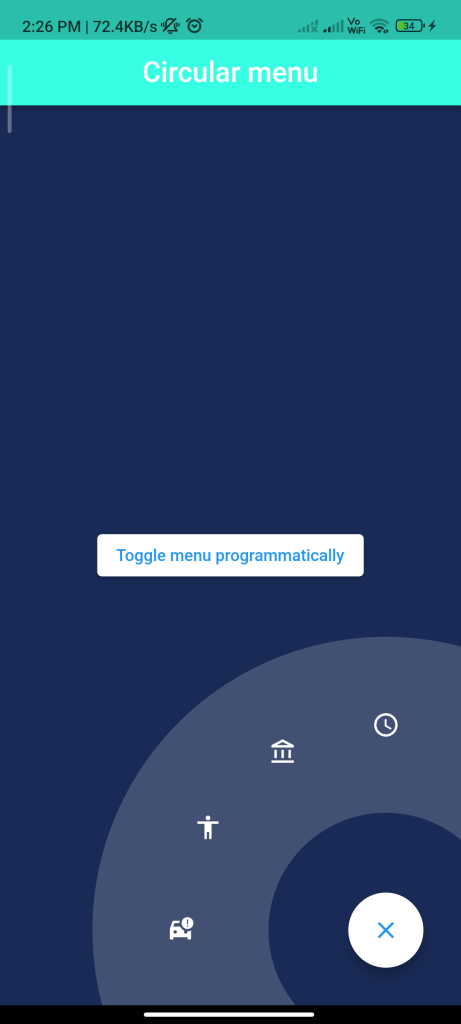
Introduction
The fab_circular_menu_plus
package allows you to create a circular menu of FABs that expand when tapped. This guide will show you how to integrate and use this package to create a FAB circular menu in your Flutter app.
Content
1.Add the fab_circular_menu_plus
dependency:
Open your pubspec.yaml
file and add the fab_circular_menu_plus
dependency.
dependencies:
fab_circular_menu_plus: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the fab_circular_menu_plus
package in your Dart file.
import 'package:fab_circular_menu_plus/fab_circular_menu_plus.dart';
3.Create a FAB circular menu:
Use the FabCircularMenu
widget to create a FAB circular menu with multiple menu items.
FabCircularMenu(
ringColor: Colors.blue,
ringDiameter: 300.0,
ringWidth: 60.0,
fabOpenIcon: Icon(Icons.menu, color: Colors.white),
fabCloseIcon: Icon(Icons.close, color: Colors.white),
children: <Widget>[
IconButton(icon: Icon(Icons.home), onPressed: () {}),
IconButton(icon: Icon(Icons.search), onPressed: () {}),
IconButton(icon: Icon(Icons.notifications), onPressed: () {}),
IconButton(icon: Icon(Icons.settings), onPressed: () {}),
],
)
Customize the ringColor
, ringDiameter
, and ringWidth
parameters to change the appearance of the circular menu. Use the fabOpenIcon
and fabCloseIcon
parameters to set the icons for opening and closing the menu. Add IconButton
widgets as children to define the menu items and their actions.
4.Run the app:
Run your Flutter app to see the FAB circular menu. Tapping the FAB will expand the menu, and tapping outside the menu will close it.
Sample Code
import 'package:flutter/material.dart';
import 'package:fab_circular_menu_plus/fab_circular_menu_plus.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
final GlobalKey<FabCircularMenuPlusState> fabKey = GlobalKey();
MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
final primaryColor = Theme.of(context).primaryColor;
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
centerTitle: true,
backgroundColor: Color.fromARGB(255, 56, 255, 228),
title: Text(
"Circular menu",
style: TextStyle(fontSize: 24),
),
),
body: Container(
color: const Color(0xFF192A56),
child: Center(
child: ElevatedButton(
onPressed: () {
// The menu can be handled programmatically using a key
if (fabKey.currentState?.isOpen ?? false) {
fabKey.currentState?.close();
} else {
fabKey.currentState?.open();
}
},
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all(Colors.white),
),
child: Text('Toggle menu programmatically', style: TextStyle(color: primaryColor)),
),
),
),
floatingActionButton: Builder(
builder: (context) => FabCircularMenuPlus(
key: fabKey,
// Cannot be `Alignment.center`
alignment: Alignment.bottomRight,
ringColor: Colors.white.withAlpha(45),
ringDiameter: 500.0,
ringWidth: 150.0,
fabSize: 64.0,
fabElevation: 8.0,
fabIconBorder: const CircleBorder(),
// Also can use specific color based on wether
// the menu is open or not:
// fabOpenColor: Colors.white
// fabCloseColor: Colors.white
// These properties take precedence over fabColor
fabColor: Colors.white,
fabOpenIcon: Icon(Icons.menu, color: primaryColor),
fabCloseIcon: Icon(Icons.close, color: primaryColor),
fabMargin: const EdgeInsets.all(16.0),
animationDuration: const Duration(milliseconds: 800),
animationCurve: Curves.easeInOutCirc,
onDisplayChange: (isOpen) {
_showSnackBar(context, "The menu is ${isOpen ? "open" : "closed"}");
},
children: <Widget>[
RawMaterialButton(
onPressed: () {
_showSnackBar(context, "You pressed 1");
},
shape: const CircleBorder(),
padding: const EdgeInsets.all(24.0),
child: const Icon(Icons.car_crash, color: Colors.white),
),
RawMaterialButton(
onPressed: () {
_showSnackBar(context, "You pressed 2");
},
shape: const CircleBorder(),
padding: const EdgeInsets.all(24.0),
child: const Icon(Icons.accessibility, color: Colors.white),
),
RawMaterialButton(
onPressed: () {
_showSnackBar(context, "You pressed 3");
},
shape: const CircleBorder(),
padding: const EdgeInsets.all(24.0),
child: const Icon(Icons.account_balance_outlined, color: Colors.white),
),
RawMaterialButton(
onPressed: () {
_showSnackBar(context, "You pressed 4. This one closes the menu on tap");
fabKey.currentState?.close();
},
shape: const CircleBorder(),
padding: const EdgeInsets.all(24.0),
child: const Icon(Icons.access_time_rounded, color: Colors.white),
),
],
),
),
),
);
}
void _showSnackBar(BuildContext context, String message) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text(message),
duration: const Duration(milliseconds: 1000),
),
);
}
}
Output
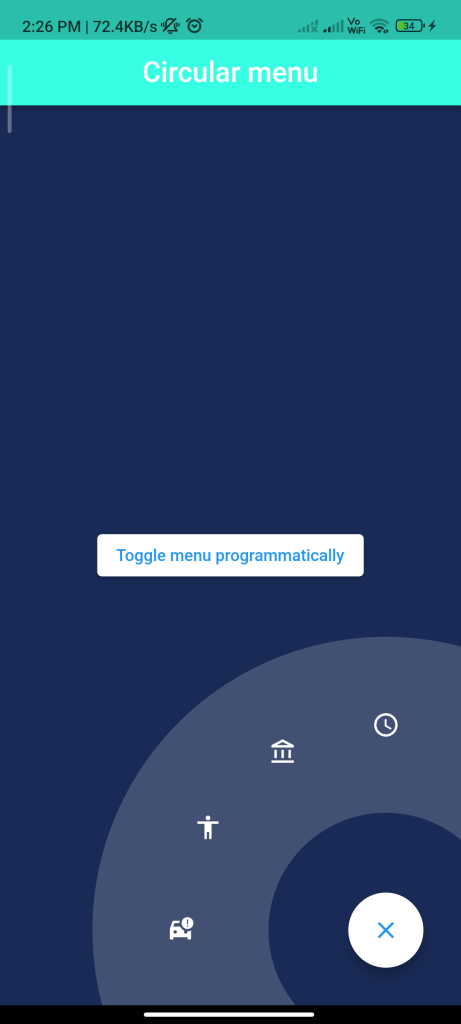
Conclusion
By following these steps, you can easily integrate the fab_circular_menu_plus
package into your Flutter app and create a FAB circular menu. This can be useful for providing quick access to multiple actions in your app.