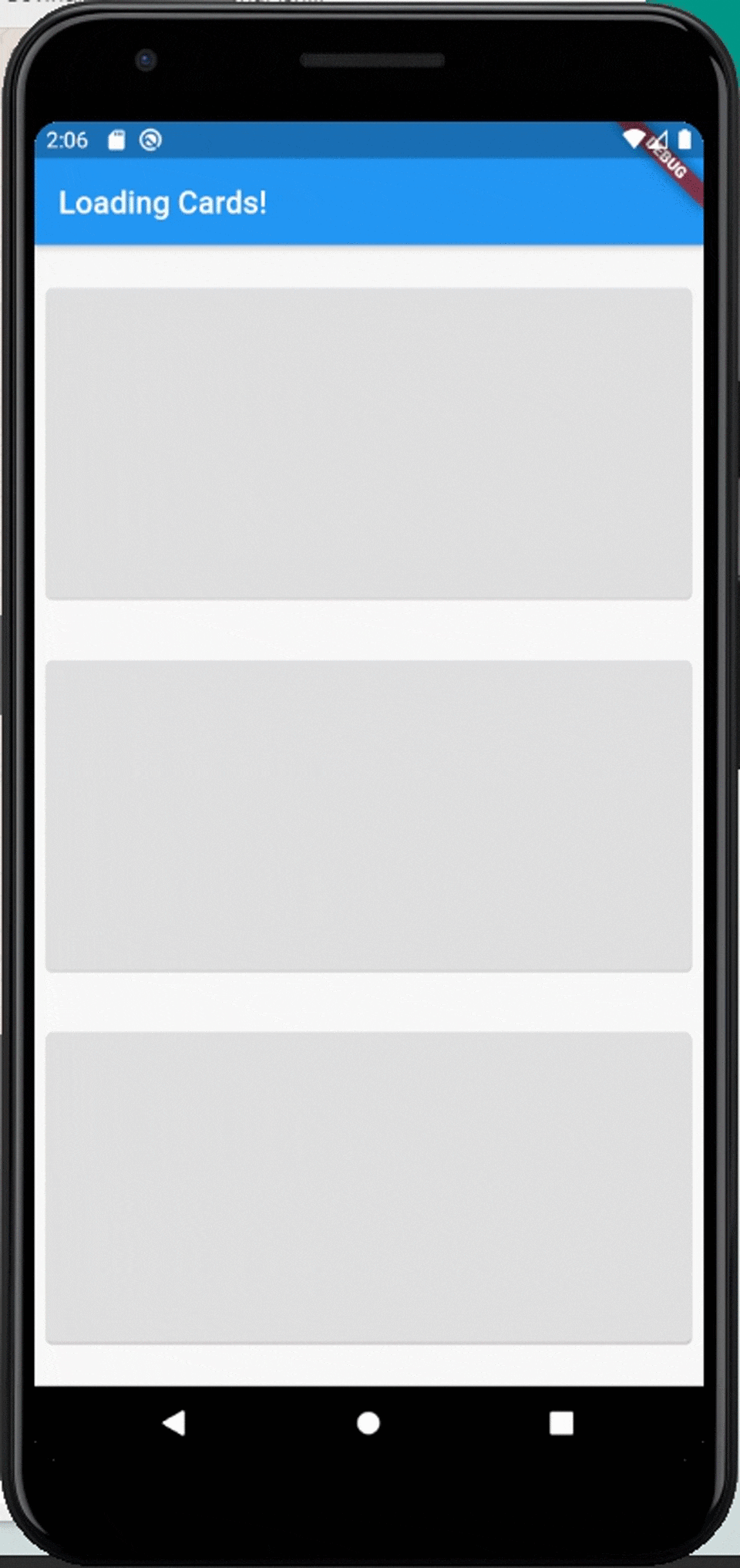
Introduction:
In Flutter, shimmer animations are used to indicate that content is loading or to provide a subtle animation effect. This tutorial will guide you through implementing a shimmer animation in Flutter using the shimmer
package.
Content:
Step 1. Add Dependencies:
Include the shimmer
package in your pubspec.yaml
file. Open the file and add the following dependency:
dependencies:
shimmer: ^2.0.0
Save the file and run flutter pub get
to fetch the package.
Step 2. Import Packages:
Import the required package in your Dart file:
import 'package:shimmer/shimmer.dart';
Step 3. Implement the Shimmer Animation:
Wrap the widget that you want to animate with the Shimmer
widget. This widget provides various parameters to customize the shimmer effect, such as duration, gradient, and direction.
Shimmer(
duration: Duration(seconds: 2), // Duration of the animation
color: Colors.grey, // Color of the shimmer effect
enabled: true, // Whether the animation is currently running
direction: ShimmerDirection.ltr, // Direction of the shimmer animation (left to right)
child: Container(
height: 100.0,
width: 200.0,
color: Colors.grey[300],
),
)
Step 4. Use the Shimmer Animation:
Add the Shimmer
widget to your app’s UI. You can place it inside a ListView
, GridView
, or any other layout widget to animate multiple items.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Shimmer Animation Example',
home: Scaffold(
appBar: AppBar(
title: Text('Shimmer Animation Example'),
),
body: Center(
child: Shimmer(
duration: Duration(seconds: 2),
color: Colors.grey,
enabled: true,
direction: ShimmerDirection.ltr,
child: Container(
height: 100.0,
width: 200.0,
color: Colors.grey[300],
child: Center(
child: Text('Shimmer'),
),
),
),
),
),
);
}
Sample Code:
// ignore_for_file: sort_child_properties_last, prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:shimmer_animation/shimmer_animation.dart';
class ShimmerAnimaation extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text("Shimmer Animation"),
backgroundColor: Colors.blue[200],
),
body: Padding(
padding: EdgeInsets.symmetric(horizontal: 5, vertical: 5),
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.start,
children: [
SizedBox(
height: 10,
),
Text(
"Restoration of a Defender 90 - without talking | Directors",
style: TextStyle(color: Colors.black, fontSize: 14, fontWeight: FontWeight.bold),
),
SizedBox(
height: 10,
),
Shimmer(
// This is the ONLY required parameter
child: Container(
decoration: BoxDecoration(color: Colors.grey[400], borderRadius: BorderRadius.circular(6)),
height: 190,
),
// This is the default value
duration: Duration(seconds: 1),
// This is NOT the default value. Default value: Duration(seconds: 0)
interval: Duration(seconds: 1),
// This is the default value
color: Colors.white,
// This is the default value
colorOpacity: 0.3,
// This is the default value
enabled: true,
// This is the default value
direction: ShimmerDirection.fromLTRB(),
),
SizedBox(
height: 20,
),
Text(
"CAMPING WITH A CAMPER TRUCK WITH STOVE IN HEAVY SNOW",
style: TextStyle(color: Colors.black, fontSize: 12, fontWeight: FontWeight.bold),
),
SizedBox(
height: 10,
),
Shimmer(
// This is the ONLY required parameter
child: Container(
height: 190,
decoration: BoxDecoration(color: Colors.grey[400], borderRadius: BorderRadius.circular(6)),
),
// This is the default value
duration: Duration(seconds: 1),
// This is NOT the default value. Default value: Duration(seconds: 0)
interval: Duration(seconds: 1),
// This is the default value
color: Colors.white,
// This is the default value
colorOpacity: 0.3,
// This is the default value
enabled: true,
// This is the default value
direction: ShimmerDirection.fromLTRB(),
),
SizedBox(
height: 20,
),
Text(
"Abandoned MONSTER Truck: Chevy 2500HD | First Wash in 12 Years! | Car Detailing Restoration",
style: TextStyle(color: Colors.black, fontSize: 14, fontWeight: FontWeight.bold),
),
SizedBox(
height: 10,
),
Shimmer(
// This is the ONLY required parameter
child: Container(
height: 190,
decoration: BoxDecoration(color: Colors.grey[400], borderRadius: BorderRadius.circular(6)),
),
// This is the default value
duration: Duration(seconds: 1),
// This is NOT the default value. Default value: Duration(seconds: 0)
interval: Duration(seconds: 1),
// This is the default value
color: Colors.white,
// This is the default value
colorOpacity: 0.3,
// This is the default value
enabled: true,
// This is the default value
direction: ShimmerDirection.fromLTRB(),
),
SizedBox(
height: 20,
),
],
),
),
),
);
}
}
Output:
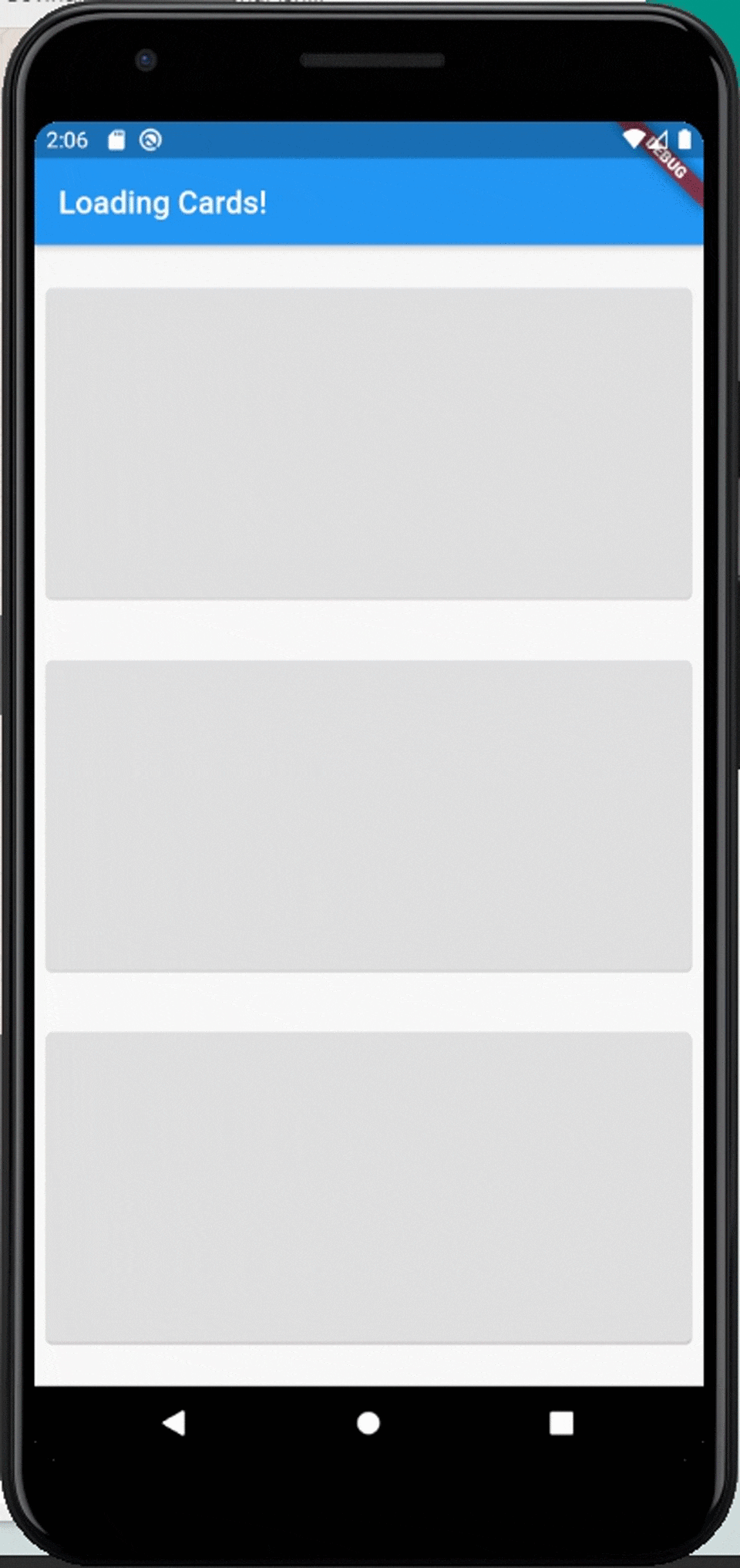
Conclusion:
By following these steps, you can easily implement a shimmer animation in Flutter using the shimmer
package. Use shimmer animations to enhance your app’s loading screens or to add subtle visual effects to your UI.