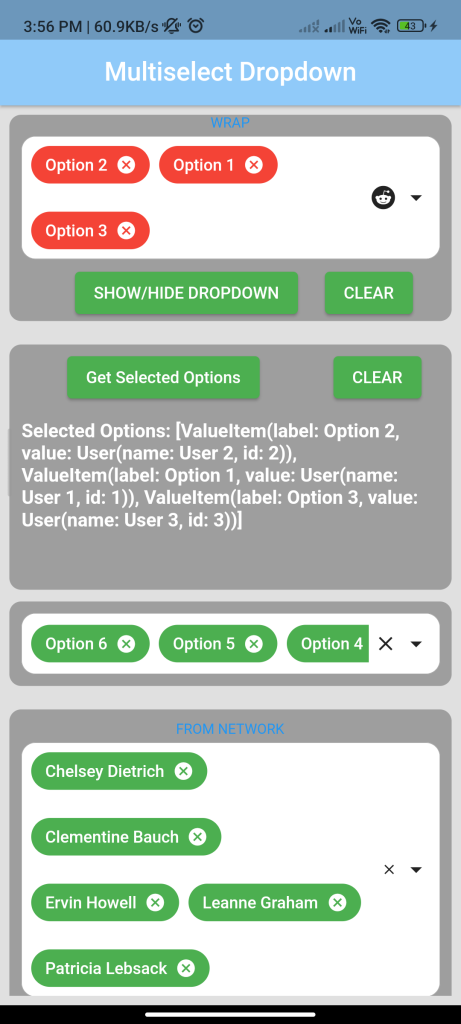
Introduction:
Implementing a multiselect dropdown in Flutter allows users to select multiple options from a dropdown list. The multi_dropdown
package provides a simple way to integrate such a dropdown into your app. This tutorial will guide you through implementing a multiselect dropdown using the multi_dropdown
package.
Content:
Step 1: Add the dependency:
Include the multi_dropdown
package in your pubspec.yaml
file:
dependencies:
multi_dropdown: ^0.1.0
Run flutter pub get
to install the package.
Step 2: Import the package:
Import the multi_dropdown
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:multi_dropdown/multi_dropdown.dart';
Step 3: Create a MultiSelectDropdown widget:
Define a stateful widget and implement the MultiSelectDropdown
widget with your desired options:
class MultiSelectDropdownScreen extends StatefulWidget {
@override
_MultiSelectDropdownScreenState createState() =>
_MultiSelectDropdownScreenState();
}
class _MultiSelectDropdownScreenState
extends State<MultiSelectDropdownScreen> {
List<String> _selectedItems = [];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('MultiSelect Dropdown Example'),
),
body: Center(
child: MultiDropdownFormField(
buttonText: 'Select Items',
value: _selectedItems,
items: ['Option 1', 'Option 2', 'Option 3', 'Option 4'],
onChanged: (values) {
setState(() {
_selectedItems = values.cast<String>();
});
},
),
),
);
}
}
In this example, the MultiDropdownFormField
widget is created with a list of options and a callback to update the selected items. You can customize the appearance and behavior of the dropdown as needed.
Step 4: Run the app:
Run your Flutter app to see the multiselect dropdown in action. You should see a dropdown that allows you to select multiple items from the list.
Sample Code:
import 'package:flutter/material.dart';
import 'package:multi_dropdown/multiselect_dropdown.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
themeMode: ThemeMode.dark,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
static const _headerStyle = TextStyle(
fontSize: 12,
color: Colors.blue,
);
}
class User {
final String name;
final int id;
User({required this.name, required this.id});
@override
String toString() {
return 'User(name: $name, id: $id)';
}
}
class _MyHomePageState extends State<MyHomePage> {
final MultiSelectController<User> _controller = MultiSelectController();
final List<ValueItem> _selectedOptions = [];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Multiselect Dropdown",
style: TextStyle(fontSize: 22, color: Colors.white, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
backgroundColor: Colors.grey.shade300,
body: SafeArea(
child: Padding(
padding: const EdgeInsets.all(8),
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
padding: EdgeInsets.symmetric(horizontal: 10),
decoration: BoxDecoration(color: Colors.grey, borderRadius: BorderRadius.circular(10)),
child: Column(
children: [
const Text('WRAP', style: MyHomePage._headerStyle),
const SizedBox(
height: 4,
),
MultiSelectDropDown<User>(
controller: _controller,
clearIcon: const Icon(Icons.reddit),
onOptionSelected: (options) {},
options: <ValueItem<User>>[
ValueItem(label: 'Option 1', value: User(name: 'User 1', id: 1)),
ValueItem(label: 'Option 2', value: User(name: 'User 2', id: 2)),
ValueItem(label: 'Option 3', value: User(name: 'User 3', id: 3)),
ValueItem(label: 'Option 4', value: User(name: 'User 4', id: 4)),
ValueItem(label: 'Option 5', value: User(name: 'User 5', id: 5)),
],
maxItems: 4,
singleSelectItemStyle: const TextStyle(fontSize: 16, fontWeight: FontWeight.bold),
chipConfig: const ChipConfig(wrapType: WrapType.wrap, backgroundColor: Colors.red),
optionTextStyle: const TextStyle(fontSize: 16),
selectedOptionIcon: const Icon(
Icons.check_circle,
color: Colors.pink,
),
searchEnabled: true,
dropdownBorderRadius: 10,
dropdownBackgroundColor: Colors.white,
selectedOptionBackgroundColor: Colors.orange,
selectedOptionTextColor: Colors.blue,
dropdownMargin: 2,
onOptionRemoved: (index, option) {},
),
const SizedBox(
height: 5,
),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
const SizedBox(
height: 4,
),
ElevatedButton(
onPressed: () {
if (_controller.isDropdownOpen) {
_controller.hideDropdown();
} else {
_controller.showDropdown();
}
},
child: const Text('SHOW/HIDE DROPDOWN'),
),
ElevatedButton(
onPressed: () {
_controller.clearAllSelection();
setState(() {
_selectedOptions.clear();
});
},
child: const Text('CLEAR'),
),
],
),
],
),
),
const SizedBox(
height: 20,
),
Container(
padding: EdgeInsets.symmetric(horizontal: 10),
decoration: BoxDecoration(color: Colors.grey, borderRadius: BorderRadius.circular(10)),
child: Column(
children: [
const SizedBox(
height: 4,
),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
const SizedBox(
width: 8,
),
ElevatedButton(
onPressed: () {
setState(() {
_selectedOptions.clear();
_selectedOptions.addAll(_controller.selectedOptions);
});
},
child: const Text('Get Selected Options'),
),
const SizedBox(
width: 8,
),
const SizedBox(
width: 8,
),
ElevatedButton(
onPressed: () {
_controller.clearAllSelection();
},
child: const Text('CLEAR'),
),
],
),
const SizedBox(
height: 12,
),
Text(
'Selected Options: $_selectedOptions',
style: const TextStyle(
fontSize: 16,
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
const SizedBox(
height: 50,
),
],
),
),
Container(
padding: EdgeInsets.symmetric(horizontal: 10, vertical: 10),
margin: EdgeInsets.symmetric(horizontal: 0, vertical: 10),
decoration: BoxDecoration(color: Colors.grey, borderRadius: BorderRadius.circular(10)),
child: MultiSelectDropDown(
onOptionSelected: (options) {
debugPrint(options.toString());
},
options: const <ValueItem>[
ValueItem(label: 'Option 1', value: '1'),
ValueItem(label: 'Option 2', value: '2'),
ValueItem(label: 'Option 3', value: '3'),
ValueItem(label: 'Option 4', value: '4'),
ValueItem(label: 'Option 5', value: '5'),
ValueItem(label: 'Option 6', value: '6'),
],
selectionType: SelectionType.multi,
chipConfig: const ChipConfig(wrapType: WrapType.scroll),
dropdownHeight: 400,
optionTextStyle: const TextStyle(fontSize: 16),
selectedOptionIcon: const Icon(Icons.check_circle),
),
),
Container(
padding: EdgeInsets.symmetric(horizontal: 10, vertical: 10),
margin: EdgeInsets.symmetric(horizontal: 0, vertical: 10),
decoration: BoxDecoration(color: Colors.grey, borderRadius: BorderRadius.circular(10)),
child: Column(
children: [
const Text('FROM NETWORK', style: MyHomePage._headerStyle),
const SizedBox(
height: 4,
),
MultiSelectDropDown.network(
dropdownHeight: 300,
onOptionSelected: (options) {
debugPrint(options.toString());
},
searchEnabled: true,
networkConfig: NetworkConfig(
url: 'https://jsonplaceholder.typicode.com/users',
method: RequestMethod.get,
headers: {
'Content-Type': 'application/json',
},
),
chipConfig: const ChipConfig(wrapType: WrapType.wrap),
responseParser: (response) {
final list = (response as List<dynamic>).map((e) {
final item = e as Map<String, dynamic>;
return ValueItem(
label: item['name'],
value: item['id'].toString(),
);
}).toList();
return Future.value(list);
},
responseErrorBuilder: ((context, body) {
return const Padding(
padding: EdgeInsets.all(16.0),
child: Text('Error fetching the data'),
);
}),
),
],
),
)
],
),
),
),
));
}
}
Output:
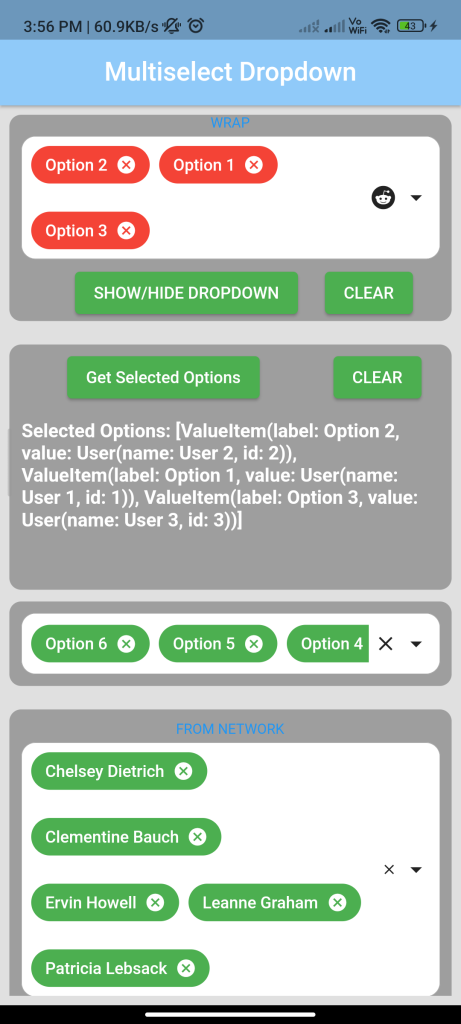
Conclusion:
By following these steps, you can easily implement a multiselect dropdown in Flutter using the multi_dropdown
package. This allows you to add a flexible and user-friendly dropdown to your app for selecting multiple options.