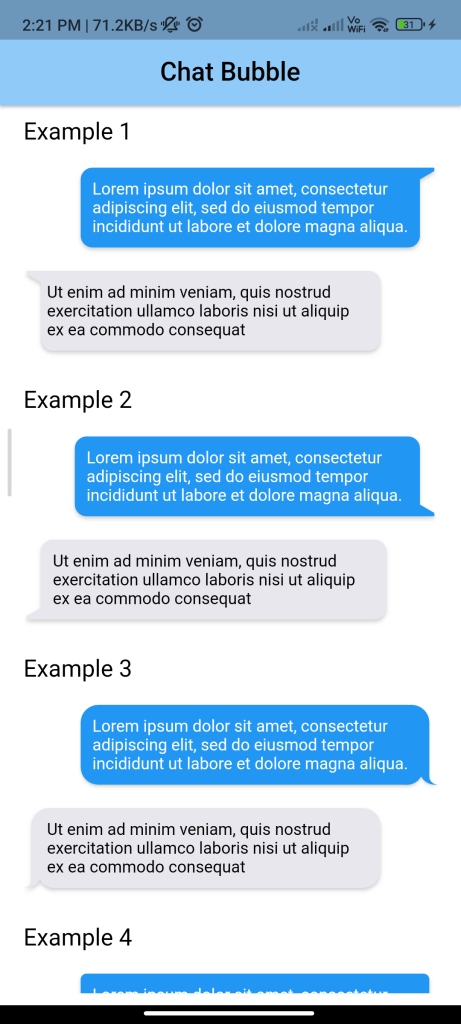
Introduction:
Implementing a chat bubble in Flutter is a common requirement for messaging apps. A chat bubble typically displays a message along with additional information such as the sender’s name, timestamp, and message status. This tutorial will guide you through implementing a basic chat bubble UI in Flutter.
Content:
Step 1: Create a ChatBubble widget:
Define a stateless widget called ChatBubble
to represent a single chat bubble. This widget will take parameters such as the message text, sender name, timestamp, and message status.
class ChatBubble extends StatelessWidget {
final String message;
final String senderName;
final String timestamp;
final bool isSender;
ChatBubble({
required this.message,
required this.senderName,
required this.timestamp,
required this.isSender,
});
@override
Widget build(BuildContext context) {
return Container(
padding: EdgeInsets.all(8.0),
margin: EdgeInsets.symmetric(vertical: 4.0, horizontal: 8.0),
decoration: BoxDecoration(
color: isSender ? Colors.blue : Colors.grey[200],
borderRadius: BorderRadius.circular(8.0),
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
senderName,
style: TextStyle(
fontWeight: FontWeight.bold,
color: isSender ? Colors.white : Colors.black,
),
),
SizedBox(height: 4.0),
Text(
message,
style: TextStyle(color: isSender ? Colors.white : Colors.black),
),
SizedBox(height: 4.0),
Text(
timestamp,
style: TextStyle(
fontSize: 12.0,
color: isSender ? Colors.white70 : Colors.black54,
),
),
],
),
);
}
}
Step 2: Create a ChatScreen widget:
Define a stateful widget called ChatScreen
to display a list of chat bubbles. For simplicity, we’ll use a ListView
to display the chat bubbles vertically.
class ChatScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Chat')),
body: ListView(
padding: EdgeInsets.all(8.0),
children: [
ChatBubble(
message: 'Hello!',
senderName: 'Alice',
timestamp: '10:00 AM',
isSender: false,
),
ChatBubble(
message: 'Hi, how are you?',
senderName: 'Bob',
timestamp: '10:01 AM',
isSender: true,
),
// Add more ChatBubble widgets as needed
],
),
);
}
}
Step 3: Run the app:
Run your Flutter app to see the chat screen with the chat bubbles. You can customize the ChatBubble
widget and ChatScreen
widget further to add more features such as images, attachments, and message status indicators.
Sample Code:
import 'package:flutter/material.dart';
import 'package:flutter_chat_bubble/chat_bubble.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Chat Bubble",
style: TextStyle(fontSize: 22, color: Colors.black, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
// appBar: AppBar(
// title: const Text('Chat Bubble'),
// ),
body: MainScreen(),
),
);
}
}
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.symmetric(vertical: 10.0, horizontal: 20),
child: ListView(
children: <Widget>[
getTitleText("Example 1"),
getSenderView(ChatBubbleClipper1(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper1(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 2"),
getSenderView(ChatBubbleClipper2(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper2(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 3"),
getSenderView(ChatBubbleClipper3(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper3(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 4"),
getSenderView(ChatBubbleClipper4(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper4(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 5"),
getSenderView(ChatBubbleClipper5(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper5(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 6"),
getSenderView(ChatBubbleClipper6(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper6(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 7"),
getSenderView(ChatBubbleClipper7(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper7(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 8"),
getSenderView(ChatBubbleClipper8(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper8(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 9"),
getSenderView(ChatBubbleClipper9(type: BubbleType.sendBubble), context),
getReceiverView(ChatBubbleClipper9(type: BubbleType.receiverBubble), context),
SizedBox(
height: 30,
),
getTitleText("Example 10"),
getSenderView(ChatBubbleClipper10(type: BubbleType.sendBubble), context),
Padding(
padding: EdgeInsets.only(bottom: 10),
child: getReceiverView(ChatBubbleClipper10(type: BubbleType.receiverBubble), context),
)
],
),
);
}
getTitleText(String title) => Text(
title,
style: TextStyle(
color: Colors.black,
fontSize: 20,
),
);
getSenderView(CustomClipper clipper, BuildContext context) => ChatBubble(
clipper: clipper,
alignment: Alignment.topRight,
margin: EdgeInsets.only(top: 20),
backGroundColor: Colors.blue,
child: Container(
constraints: BoxConstraints(
maxWidth: MediaQuery.of(context).size.width * 0.7,
),
child: Text(
"Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.",
style: TextStyle(color: Colors.white),
),
),
);
getReceiverView(CustomClipper clipper, BuildContext context) => ChatBubble(
clipper: clipper,
backGroundColor: Color(0xffE7E7ED),
margin: EdgeInsets.only(top: 20),
child: Container(
constraints: BoxConstraints(
maxWidth: MediaQuery.of(context).size.width * 0.7,
),
child: Text(
"Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat",
style: TextStyle(color: Colors.black),
),
),
);
}
Output:
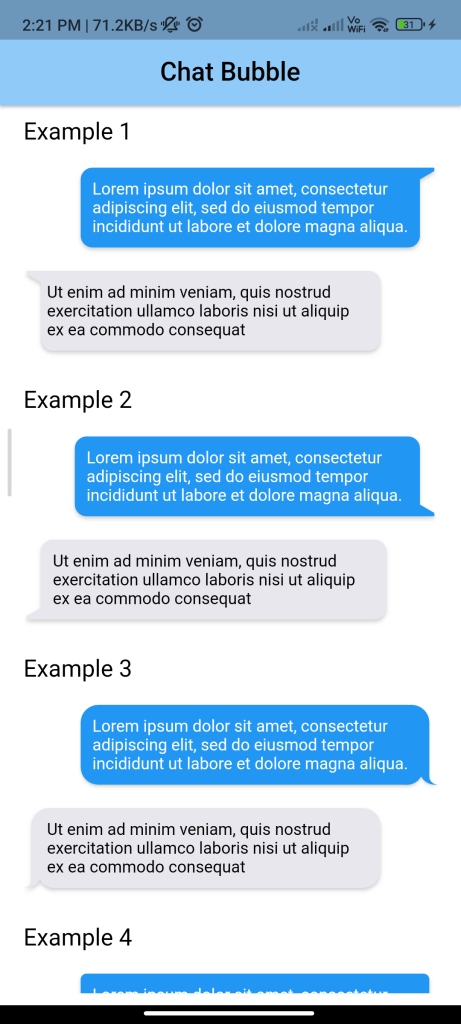
Conclusion:
By following these steps, you can implement a basic chat bubble UI in Flutter. This can serve as a foundation for building more advanced messaging features in your app.