Introduction:
A captivating splash screen can make a lasting impression on users and set the tone for your Flutter app. An animated splash screen not only adds visual appeal but also provides a seamless transition into your app’s main interface. In this step-by-step guide, you will learn how to create an animated splash screen in Flutter, complete with animations and transitions. Elevate your app’s user experience by incorporating a stunning splash screen that engages and captivates your users right from the start.
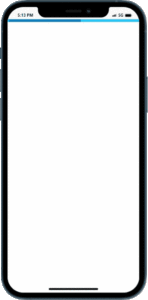
Content:
1. Set up a new Flutter project:
Before we begin, ensure that you have Flutter installed and set up on your machine. Create a new Flutter project using the following command in your terminal:
flutter create animated_splash_screen
2. Prepare the animation assets:
To create an animated splash screen, you will need animation assets such as images or GIFs. Prepare your animation assets by either designing them or sourcing them from reliable resources. Make sure the assets are compatible with Flutter and optimized for performance.
3. Implement the animated splash screen:
In your Flutter project, create a new Dart file called splash_screen.dart
. This file will contain the code for your animated splash screen. Implement the following code in the splash_screen.dart
file:
import 'package:flutter/material.dart';
class SplashScreen extends StatefulWidget {
@override
_SplashScreenState createState() => _SplashScreenState();
}
class _SplashScreenState extends State<SplashScreen>
with SingleTickerProviderStateMixin {
AnimationController? _animationController;
Animation<double>? _animation;
@override
void initState() {
super.initState();
_animationController = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
);
_animation =
CurvedAnimation(parent: _animationController!, curve: Curves.easeIn);
_animationController!.forward();
Future.delayed(const Duration(seconds: 3), () {
// Navigate to the home screen or the next screen
});
}
@override
void dispose() {
_animationController!.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: FadeTransition(
opacity: _animation!,
child: Container(
// Your animation widget here
),
),
);
}
}
Replace the Container
widget with your animation widget. You can use an Image
widget, AnimatedContainer
, or any other animation widget of your choice to display your animation assets.
4. Update the main app widget:
Open the main.dart
file and update the main app widget (MyApp
) as follows:
import 'package:flutter/material.dart';
import 'splash_screen.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Animated Splash Screen',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: SplashScreen(),
);
}
}
5. Customize the splash screen:
Customize the splash screen by adding your own animation assets, branding elements, and transitions. Experiment with different animations, durations, and effects to create a unique and engaging splash screen for your app.
6. Test the animated splash screen:
Save your changes and run the app using the following command in your terminal:
flutter run
7. Observe the animated splash screen in action:
Upon running the app, you will see your animated splash screen displayed for the specified duration. Once the animation completes, you can navigate to the home screen or the next screen in your app.
Sample Code:
import 'package:flutter/material.dart';
import 'package:flutter_sequence_animation/flutter_sequence_animation.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Animated Splash Screen',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: SplashScreen(),
);
}
}
class SplashScreen extends StatefulWidget {
@override
_SplashScreenState createState() => _SplashScreenState();
}
class _SplashScreenState extends State<SplashScreen>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late SequenceAnimation _sequenceAnimation;
@override
void initState() {
super.initState();
_controller = AnimationController(
vsync: this,
duration: Duration(milliseconds: 2000),
);
_sequenceAnimation = SequenceAnimationBuilder()
.addAnimatable(
animatable: Tween<double>(begin: 0.0, end: 1.0),
from: Duration(milliseconds: 0),
to: Duration(milliseconds: 1000),
tag: 'opacity',
)
.addAnimatable(
animatable: Tween<double>(begin: -40.0, end: 50.0),
from: Duration(milliseconds: 10),
to: Duration(milliseconds: 1000),
curve: Curves.easeOut,
tag: 'translate',
)
.animate(_controller);
_controller.addStatusListener((status) {
if (status == AnimationStatus.completed) {
Navigator.pushReplacement(
context,
MaterialPageRoute(builder: (context) => HomeScreen()),
);
}
});
_controller.forward();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors. white,
body: Center(
child: AnimatedBuilder(
animation: _controller,
builder: (BuildContext context, Widget? child) {
return Opacity(
opacity: _sequenceAnimation['opacity'].value,
child: Transform.translate(
offset: Offset(0, _sequenceAnimation['translate'].value),
child: Image.network("https://png.pngtree.com/png-vector/20190303/ourmid/pngtree-modern-abstract-3d-logo-png-image_771012.jpg",fit: BoxFit.fill,height: 400,width: 400,)
),
);
},
),
),
);
}
}
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Home'),
),
body: Center(
child: Text('Welcome to the Home Screen!'),
),
);
}
}
Output:
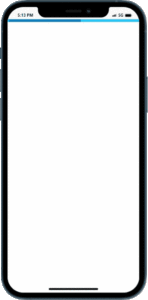
Conclusion:
Congratulations! You have successfully created an animated splash screen in your Flutter app. By following this step-by-step guide, you have learned how to implement an engaging animated introduction that enhances the user experience. Make a lasting impression on your app users right from the start with a captivating animated splash screen.