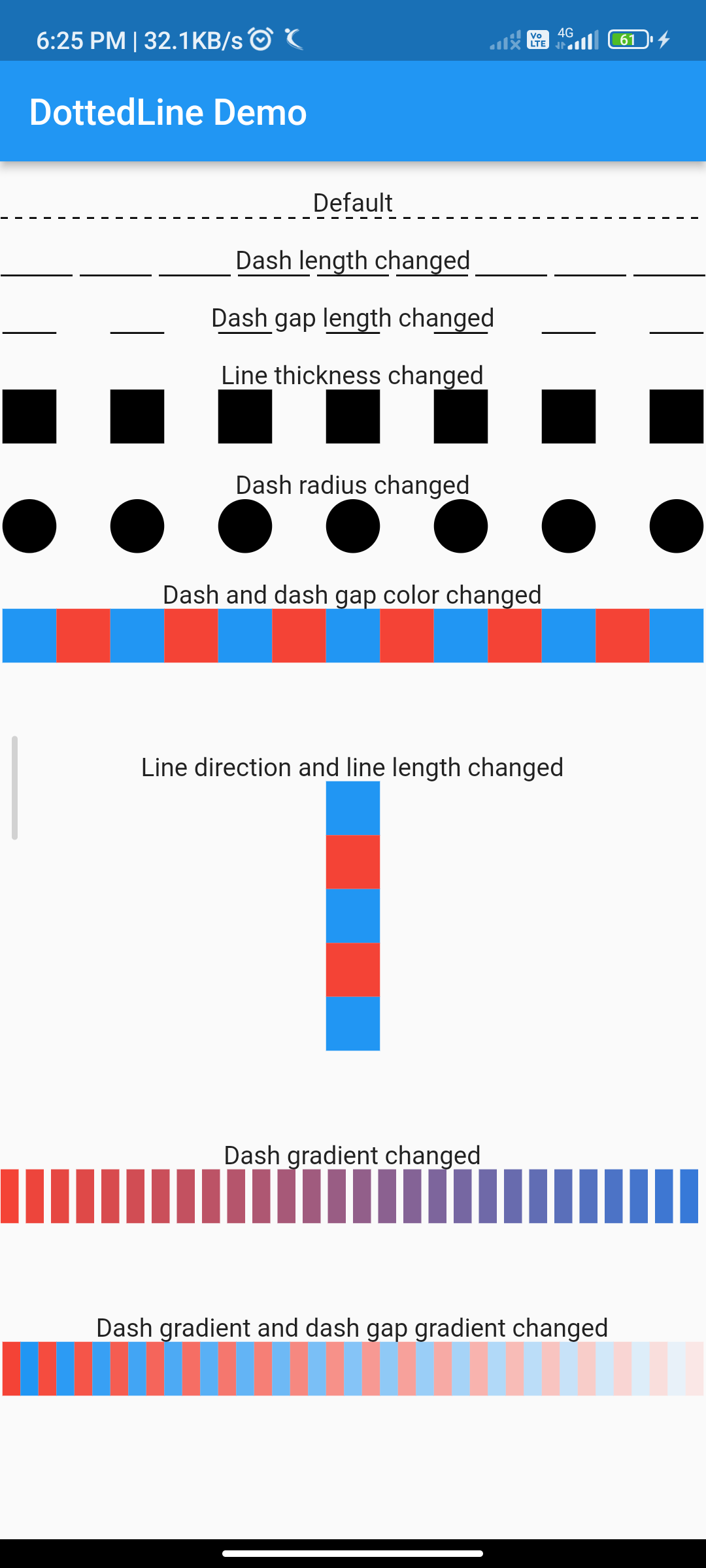
Introduction:
Adding a dotted line in a Flutter app can be useful for visual separation or decorative purposes. In this guide, we’ll walk through the steps to implement a dotted line using the dotted_line
package.
Content:
Step 1: Create a New Flutter Project
Ensure that you have Flutter installed and set up on your machine. Create a new Flutter project using the following command in your terminal:
flutter create dotted_line_app
Step 2: Add the dotted_line
Dependency
Open the pubspec.yaml
file in your Flutter project and add the dotted_line
dependency:
dependencies:
dotted_line: ^2.0.0
Save the file and run flutter pub get
in your terminal.
Step 3: Display Dotted Line in Flutter
Open the lib/main.dart
file and replace its content with the following code:
import 'package:flutter/material.dart';
import 'package:dotted_line/dotted_line.dart';
void main() {
runApp(DottedLineApp());
}
class DottedLineApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Dotted Line Example"),
),
body: Center(
child: DottedLine(
lineLength: 200.0,
lineThickness: 2.0,
lineColor: Colors.black,
dashLength: 4.0,
dashColor: Colors.red,
dashRadius: 0.0,
),
),
),
);
}
}
Step 4: Run the App
Save your changes and run the app using the following command in your terminal:
flutter run