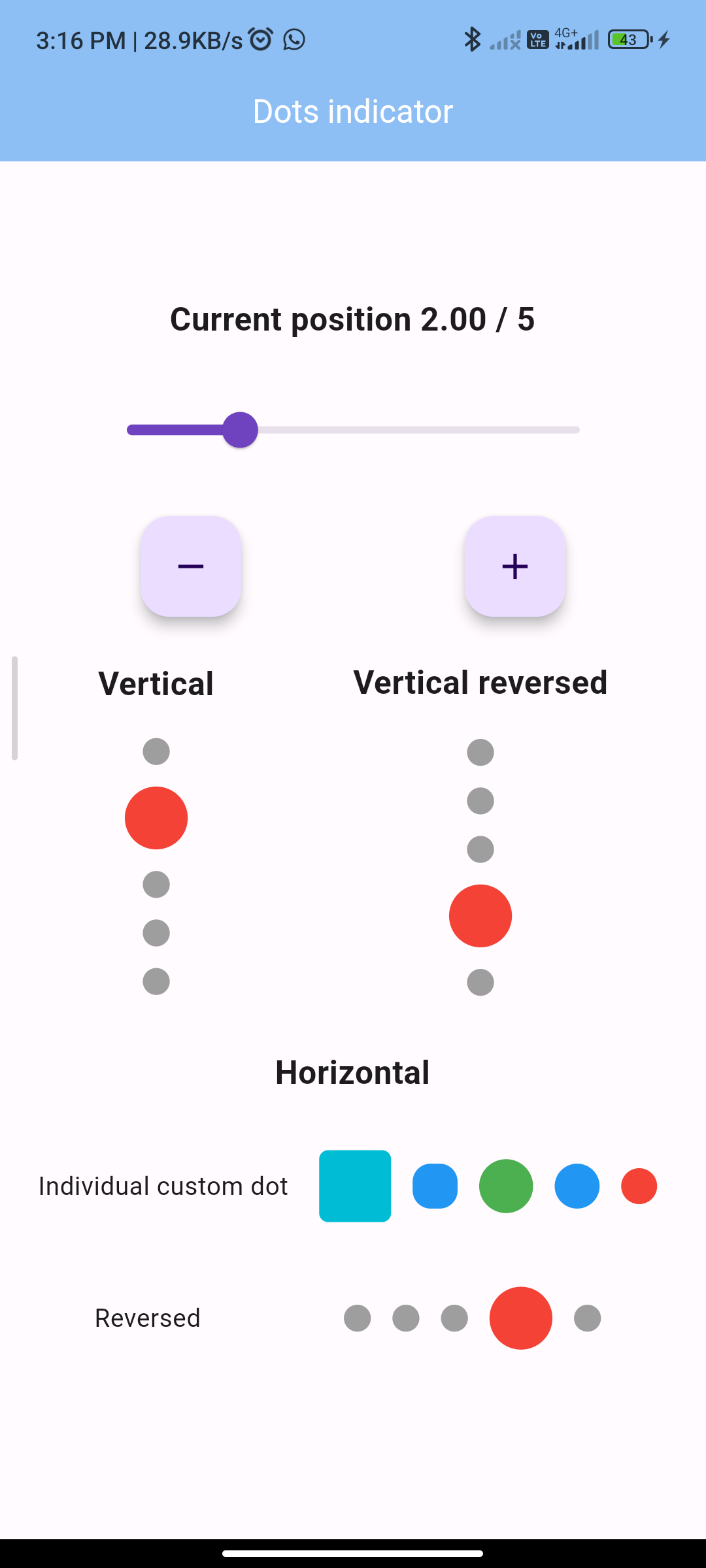
Introduction:
The `dots_indicator` package provides a customizable dots indicator widget for Flutter applications. It is commonly used to indicate the current position within a series of pages or slides. This guide will walk you through the process of implementing a dots indicator in your Flutter app.
Content:
Step 1: Add Dependency Ensure you have the `dots_indicator` package added to your `pubspec.yaml` file.
Run the following command in your terminal:
dots_indicator: ^3.0.0
Run flutter pub get
to fetch the package.
Step 2: Import Dependencies
In your Dart file, import the necessary packages:
import 'package:flutter/material.dart';
import 'package:dots_indicator/dots_indicator.dart';
Step 3: Create Dots Indicator Widget
Create a widget to display the dots indicator. For example:
class DotsIndicatorWidget extends StatefulWidget {
@override
_DotsIndicatorWidgetState createState() => _DotsIndicatorWidgetState();
}
class _DotsIndicatorWidgetState extends State<DotsIndicatorWidget> {
int _currentIndex = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Dots Indicator Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Page $_currentIndex',
style: TextStyle(fontSize: 24),
),
SizedBox(height: 20),
DotsIndicator(
dotsCount: 5, // Total number of dots
position: _currentIndex.toDouble(), // Current position
decorator: DotsDecorator(
size: Size(10, 10), // Size of each dot
color: Colors.grey, // Color of inactive dots
activeColor: Colors.blue, // Color of active dot
activeSize: Size(15, 15), // Size of active dot
spacing: EdgeInsets.all(5), // Spacing between dots
),
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_currentIndex = (_currentIndex + 1) % 5; // Update current index
});
},
child: Icon(Icons.navigate_next),
),
);
}
}
Step 4: Run the Application
Run your Flutter application and navigate to the screen containing the dots indicator. You should see the dots indicating the current position within the series of pages.
Sample Code:
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:dots_indicator/dots_indicator.dart';
class DotsIndicato extends StatefulWidget {
@override
_DotsIndicatoState createState() => _DotsIndicatoState();
}
class _DotsIndicatoState extends State<DotsIndicato> {
final _totalDots = 5;
int _currentPosition = 0;
int _validPosition(int position) {
if (position >= _totalDots) return 0;
if (position < 0) return _totalDots - 1;
return position;
}
void _updatePosition(int position) {
setState(() => _currentPosition = _validPosition(position));
}
Widget _buildRow(List<Widget> widgets) {
return Padding(
padding: const EdgeInsets.only(bottom: 24.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: widgets,
),
);
}
String getPrettyCurrPosition() {
return (_currentPosition + 1.0).toStringAsPrecision(3);
}
@override
Widget build(BuildContext context) {
final decorator = DotsDecorator(
activeColor: Colors.red,
size: Size.square(15.0),
activeSize: Size.square(35.0),
activeShape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(25.0),
),
);
const titleStyle = TextStyle(
fontWeight: FontWeight.w700,
fontSize: 18.0,
);
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 3,
title: Text(
'Dots indicator',
style: TextStyle(fontSize: 18, color: Colors.white),
),
backgroundColor: Colors.blue[200],
),
// appBar: AppBar(
// title: const Text('Dots indicator example'),
// ),
body: Center(
child: ListView(
shrinkWrap: true,
padding: const EdgeInsets.all(16.0),
children: [
_buildRow([
Text(
'Current position ${getPrettyCurrPosition()} / $_totalDots',
style: titleStyle,
),
]),
_buildRow([
SizedBox(
width: 300.0,
child: Slider(
value: _currentPosition.toDouble(),
max: (_totalDots - 1).toDouble(),
onChanged: (val) {
_updatePosition(val.round());
},
),
),
]),
_buildRow([
FloatingActionButton(
child: const Icon(Icons.remove),
onPressed: () {
_updatePosition(max(--_currentPosition, 0));
},
),
FloatingActionButton(
child: const Icon(Icons.add),
onPressed: () {
_updatePosition(min(
++_currentPosition,
_totalDots,
));
},
)
]),
_buildRow([
Column(
children: [
const Text('Vertical', style: titleStyle),
const SizedBox(height: 11.0),
DotsIndicator(
dotsCount: _totalDots,
position: _currentPosition,
axis: Axis.vertical,
decorator: decorator,
onTap: (pos) {
setState(() => _currentPosition = pos);
},
),
],
),
Column(
children: [
const Text('Vertical reversed', style: titleStyle),
const SizedBox(height: 12.0),
DotsIndicator(
dotsCount: _totalDots,
position: _currentPosition,
axis: Axis.vertical,
reversed: true,
decorator: decorator,
),
],
),
]),
_buildRow([
const Text('Horizontal', style: titleStyle),
]),
_buildRow([
const Text('Individual custom dot'),
DotsIndicator(
dotsCount: _totalDots,
position: _currentPosition,
decorator: DotsDecorator(
colors: [
Colors.red,
Colors.blue,
Colors.green,
Colors.yellow,
Colors.cyan,
].reversed.toList(),
activeColors: [
Colors.red,
Colors.blue,
Colors.green,
Colors.yellow,
Colors.cyan,
],
sizes: [
Size.square(40.0),
Size.square(35.0),
Size.square(30.0),
Size.square(25.0),
Size.square(20.0),
],
activeSizes: [
Size.square(20.0),
Size.square(25.0),
Size.square(30.0),
Size.square(35.0),
Size.square(10.0),
],
shapes: [
RoundedRectangleBorder(borderRadius: BorderRadius.circular(5.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(10.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(15.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(20.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(25.0)),
],
activeShapes: [
RoundedRectangleBorder(borderRadius: BorderRadius.circular(5.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(10.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(15.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(20.0)),
RoundedRectangleBorder(borderRadius: BorderRadius.circular(25.0)),
],
),
),
]),
_buildRow([
const Text('Reversed'),
DotsIndicator(
dotsCount: _totalDots,
position: _currentPosition,
reversed: true,
decorator: decorator,
),
]),
],
),
),
);
}
}
Output:
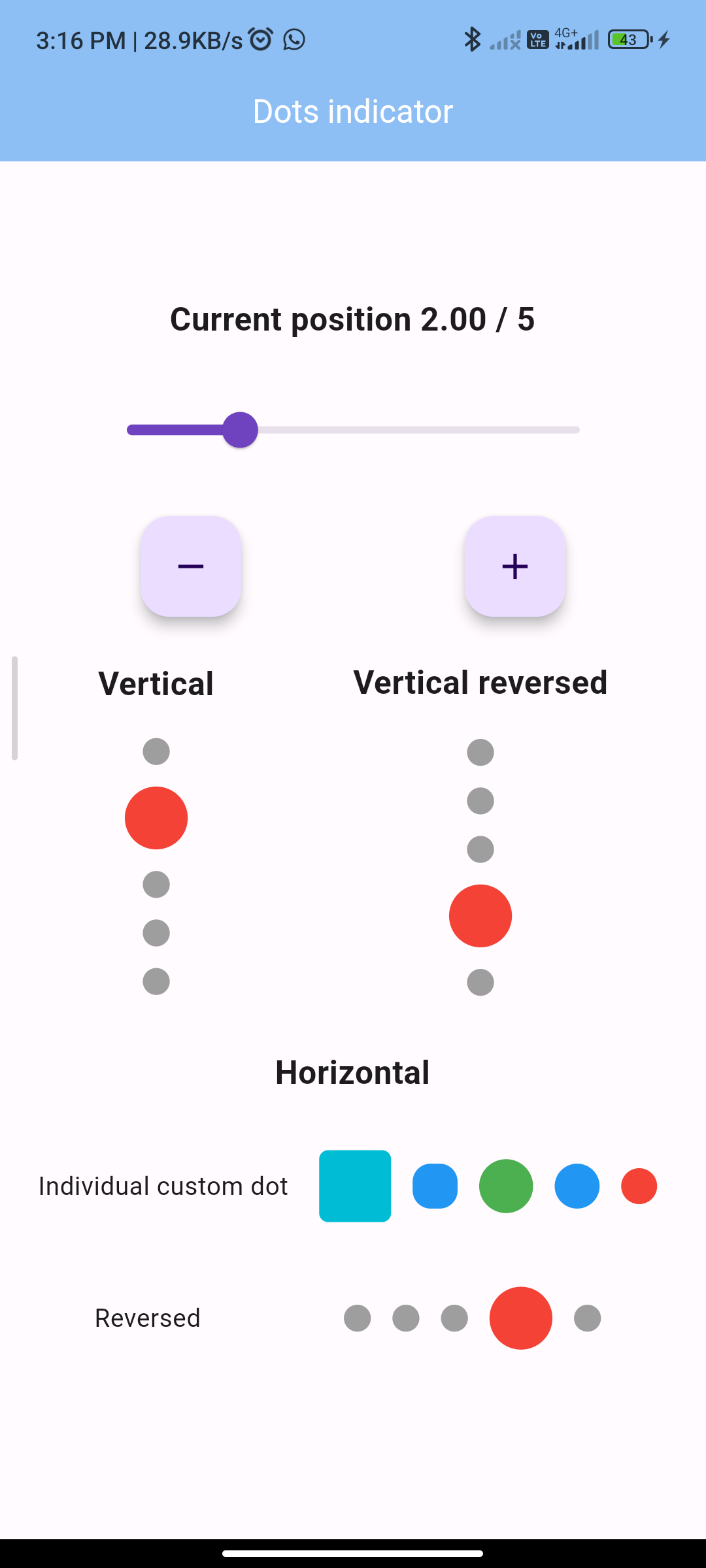
Conclusion:
Congratulations! You’ve successfully implemented a dots indicator in your Flutter app using the dots_indicator
package. You can further customize the dots indicator by adjusting its properties such as size, color, spacing, and more according to your app’s design requirements.