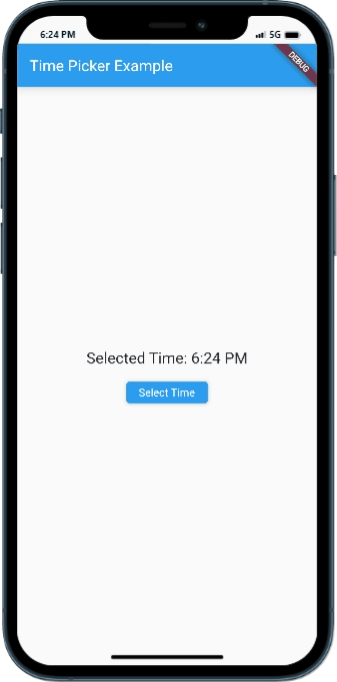
Introduction
A time picker is a useful component in mobile apps for allowing users to select a specific time. In Flutter, you can easily implement a time picker in your app using the flutter_datetime_picker
package. This tutorial will guide you through the process of integrating the time picker widget and retrieving the selected time values.
Content
To implement a time picker in Flutter, follow these steps:
Step 1.Import the flutter_datetime_picker
Package:
- Open your Flutter project in your preferred code editor.
- Open the
pubspec.yaml
file. - In the
dependencies
section, add the following line:flutter_datetime_picker: ^2.0.0
. - Save the
pubspec.yaml
file. The code editor should automatically detect the changes and prompt you to runflutter pub get
. Run this command to fetch the package.
Step 2.Import and Use the Time Picker Widget:
- Open the Dart file where you want to implement the time picker.
- Import the necessary packages:
import 'package:flutter/material.dart';
import 'package:flutter_datetime_picker/flutter_datetime_picker.dart';
- Use the
showTimePicker
method provided by theflutter_datetime_picker
package to display the time picker widget. For example:
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Time Picker App',
home: Scaffold(
appBar: AppBar(
title: Text('Time Picker App'),
),
body: Center(
child: RaisedButton(
child: Text('Select Time'),
onPressed: () {
DatePicker.showTimePicker(
context,
showSecondsColumn: false,
onChanged: (time) {
print('Selected time: $time');
},
);
},
),
),
),
);
}
}
In this code snippet, we import the flutter_datetime_picker
package and use the DatePicker.showTimePicker
method to display the time picker widget when the button is pressed. The onChanged
callback allows you to handle the selected time. In this example, we simply print the selected time to the console.
Step 3.Run the App:
Save the changes made to your Dart file. Execute the following command in your terminal to run the app:
flutter run
Sample Code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Time Picker Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
TimeOfDay? _selectedTime;
@override
void initState() {
super.initState();
_selectedTime = TimeOfDay.now();
}
Future<void> _showTimePicker() async {
final TimeOfDay? pickedTime = await showTimePicker(
context: context,
initialTime: _selectedTime!,
);
if (pickedTime != null && pickedTime != _selectedTime) {
setState(() {
_selectedTime = pickedTime;
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Time Picker Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Selected Time: ${_selectedTime?.format(context) ?? ''}',
style: TextStyle(fontSize: 20),
),
SizedBox(height: 20),
ElevatedButton(
child: Text('Select Time'),
onPressed: _showTimePicker,
),
],
),
),
);
}
}
Output:
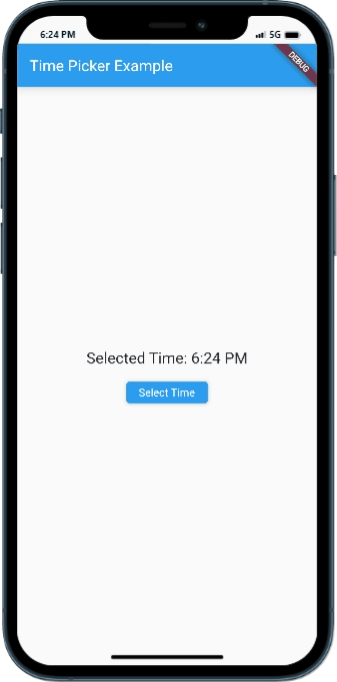
Conclusion:
Congratulations! You have successfully implemented a time picker in your Flutter app using the flutter_datetime_picker
package. By following this tutorial, you learned how to import the package, use the showTimePicker
method to display the time picker widget, and handle the selected time. Feel free to customize the time picker’s appearance and integrate it into your app’s specific use case.