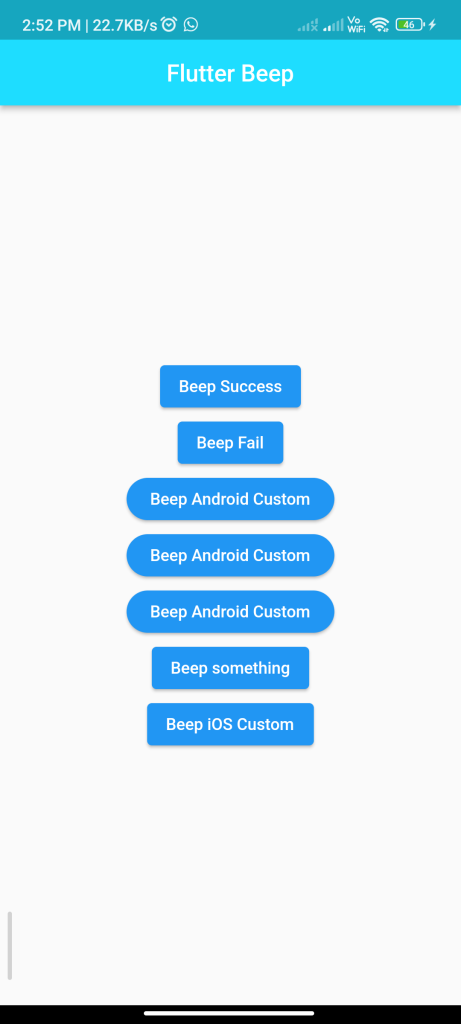
Introduction
The flutter_beep
package allows you to play system beeps in your Flutter app. This guide will show you how to integrate and use this package to play system beeps in your Flutter app.
Content
1.Add the flutter_beep
dependency:
Open your pubspec.yaml
file and add the flutter_beep
dependency.
dependencies:
flutter_beep: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the flutter_beep
package in your Dart file.
import 'package:flutter_beep/flutter_beep.dart';
3.Play a system beep:
Use the FlutterBeep.playSysSound
method to play a system beep.
FlutterBeep.playSysSound(0); // Play default system beep sound
You can pass different sound IDs to play different system beep sounds. Refer to the system sound IDs documentation for more details.
4.Run the app:
Run your Flutter app to hear the system beep sound. You should hear the default system beep sound or the specified system beep sound.
Sample Code
import 'package:flutter/material.dart';
import 'package:flutter_beep/flutter_beep.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
centerTitle: true,
backgroundColor: Color.fromARGB(255, 30, 221, 255),
title: const Text('Flutter Beep'),
),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
child: Text("Beep Success"),
onPressed: () => FlutterBeep.beep(),
),
ElevatedButton(
child: Text("Beep Fail"),
onPressed: () => FlutterBeep.beep(false),
),
ElevatedButton(
style: ElevatedButton.styleFrom(
primary: Colors.blue,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
padding: EdgeInsets.symmetric(horizontal: 20.0, vertical: 10.0),
),
child: Text("Beep Android Custom"),
onPressed: () => FlutterBeep.playSysSound(AndroidSoundIDs.TONE_CDMA_CALL_SIGNAL_ISDN_PING_RING),
),
ElevatedButton(
style: ElevatedButton.styleFrom(
primary: Colors.blue,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
padding: EdgeInsets.symmetric(horizontal: 20.0, vertical: 10.0),
),
child: Text("Beep Android Custom"),
onPressed: () => FlutterBeep.playSysSound(AndroidSoundIDs.TONE_CDMA_PRESSHOLDKEY_LITE),
),
ElevatedButton(
style: ElevatedButton.styleFrom(
primary: Colors.blue,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
padding: EdgeInsets.symmetric(horizontal: 20.0, vertical: 10.0),
),
child: Text("Beep Android Custom"),
onPressed: () => FlutterBeep.playSysSound(AndroidSoundIDs.TONE_CDMA_PRESSHOLDKEY_LITE),
//TONE_CDMA_PRESSHOLDKEY_LITE select this and hold ctrl double click mouse right button to see more option
),
ElevatedButton(
child: Text("Beep something"),
onPressed: () => FlutterBeep.playSysSound(41),
),
ElevatedButton(
child: Text("Beep iOS Custom"),
onPressed: () => FlutterBeep.playSysSound(iOSSoundIDs.AudioToneBusy),
),
],
),
),
),
);
}
}
Output
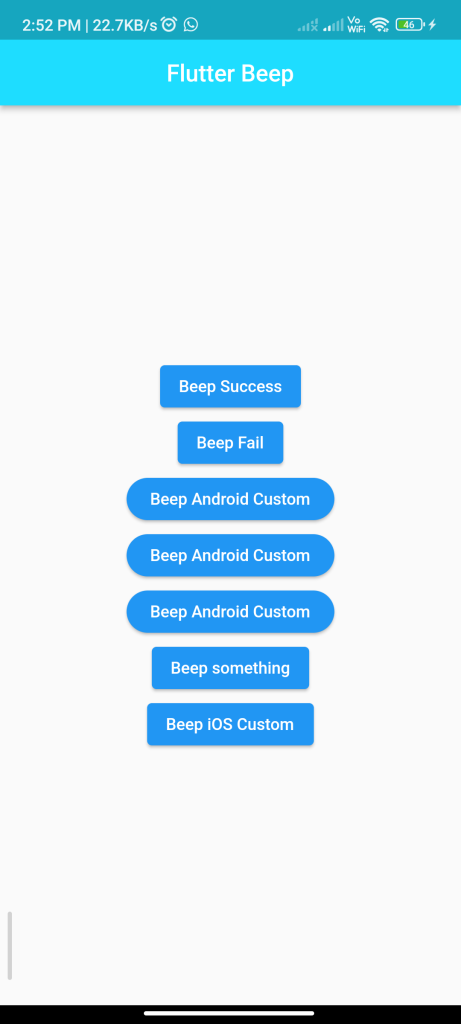
Conclusion
By following these steps, you can easily integrate the flutter_beep
package into your Flutter app and play system beep sounds. This can be useful for providing audio feedback to users or for signaling events in your app.