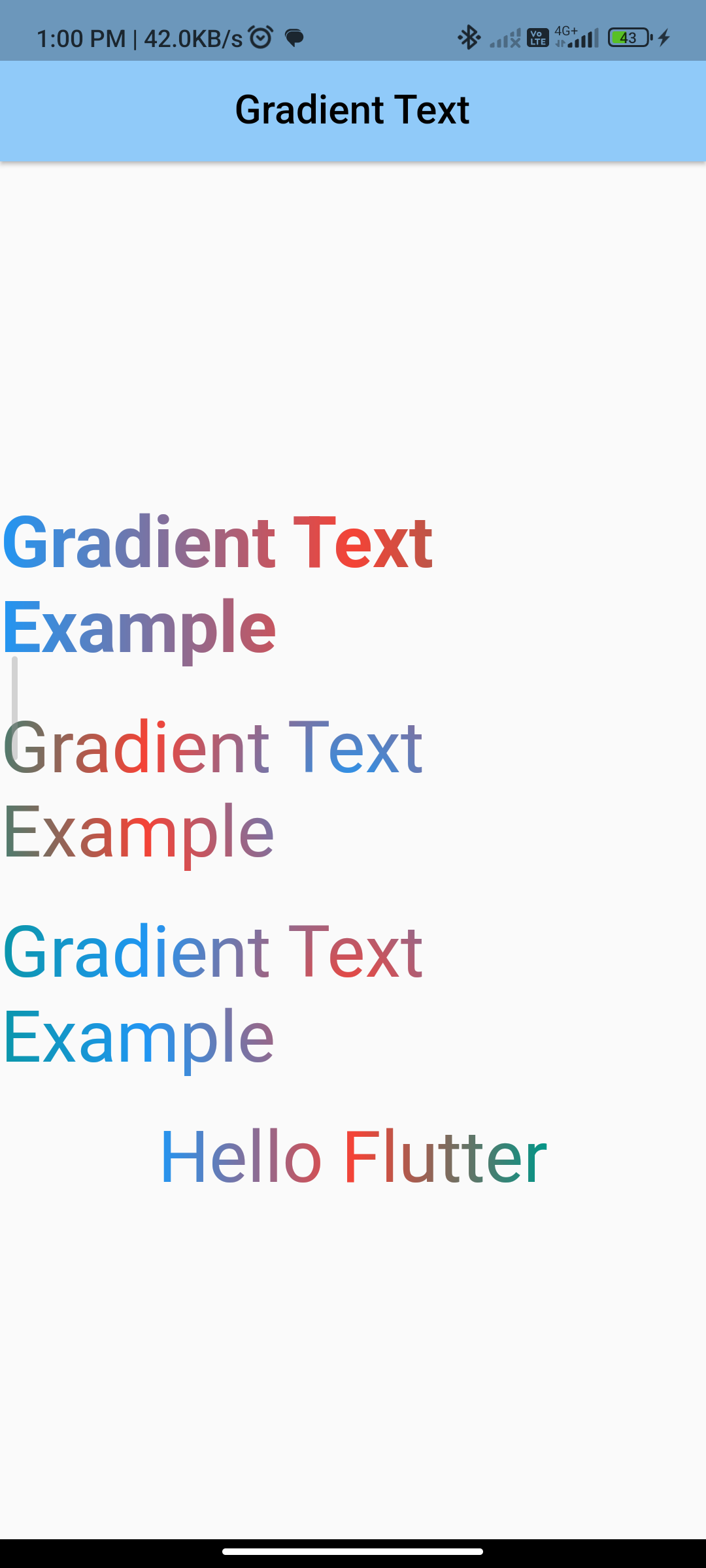
Introduction:
Implementing gradient text in Flutter allows you to add colorful and visually appealing text to your app. The simple_gradient_text
package provides a simple way to create gradient text in Flutter. This tutorial will guide you through implementing gradient text using the simple_gradient_text
package.
Content:
Step 1: Add the dependency:
Add the simple_gradient_text
package to your pubspec.yaml
file:
dependencies:
simple_gradient_text: ^1.0.0
Save the file and run flutter pub get
to install the package.
Step 2: Import the package:
Import the simple_gradient_text
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:simple_gradient_text/simple_gradient_text.dart';
Step 3: Use the GradientText
widget:
Use the GradientText
widget to create gradient text. Specify the text, gradient colors, and other properties:
GradientText(
'Hello, Gradient!',
gradientDirection: GradientDirection.diagonal,
colors: [Colors.blue, Colors.green],
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold),
),
In this example, the GradientText
widget is used to display the text “Hello, Gradient!” with a diagonal gradient from blue to green.
Step 4: Run the app:
Run your Flutter app to see the gradient text in action.
Sample Code:
import 'package:flutter/material.dart';
import 'package:simple_gradient_text/simple_gradient_text.dart';
class GradiantText extends StatelessWidget {
const GradiantText({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Gradient Text',
home: Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Gradient Text",
style: TextStyle(fontSize: 22, color: Colors.black, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
GradientText(
'Gradient Text Example',
style: const TextStyle(fontSize: 40.0, fontWeight: FontWeight.bold),
colors: const [
Colors.blue,
Colors.red,
Colors.teal,
],
),
SizedBox(
height: 20,
),
// This is a example for RadialGradient
// Use Radius when use radial gradient text
GradientText(
'Gradient Text Example',
style: const TextStyle(
fontSize: 40.0,
),
gradientType: GradientType.radial,
radius: 2.5,
colors: const [
Colors.blue,
Colors.red,
Colors.teal,
],
),
SizedBox(
height: 20,
),
// This is a example for RadialGradient
// Use Radius when use radial gradient text
GradientText(
'Gradient Text Example',
style: const TextStyle(
fontSize: 40.0,
),
gradientType: GradientType.radial,
radius: 2.5,
colors: const [
Colors.red,
Colors.blue,
Colors.teal,
],
),
SizedBox(
height: 20,
),
GradientText(
'Hello Flutter',
style: const TextStyle(fontSize: 40),
colors: const [
Colors.blue,
Colors.red,
Colors.teal,
],
),
],
),
),
),
);
}
}
Output:
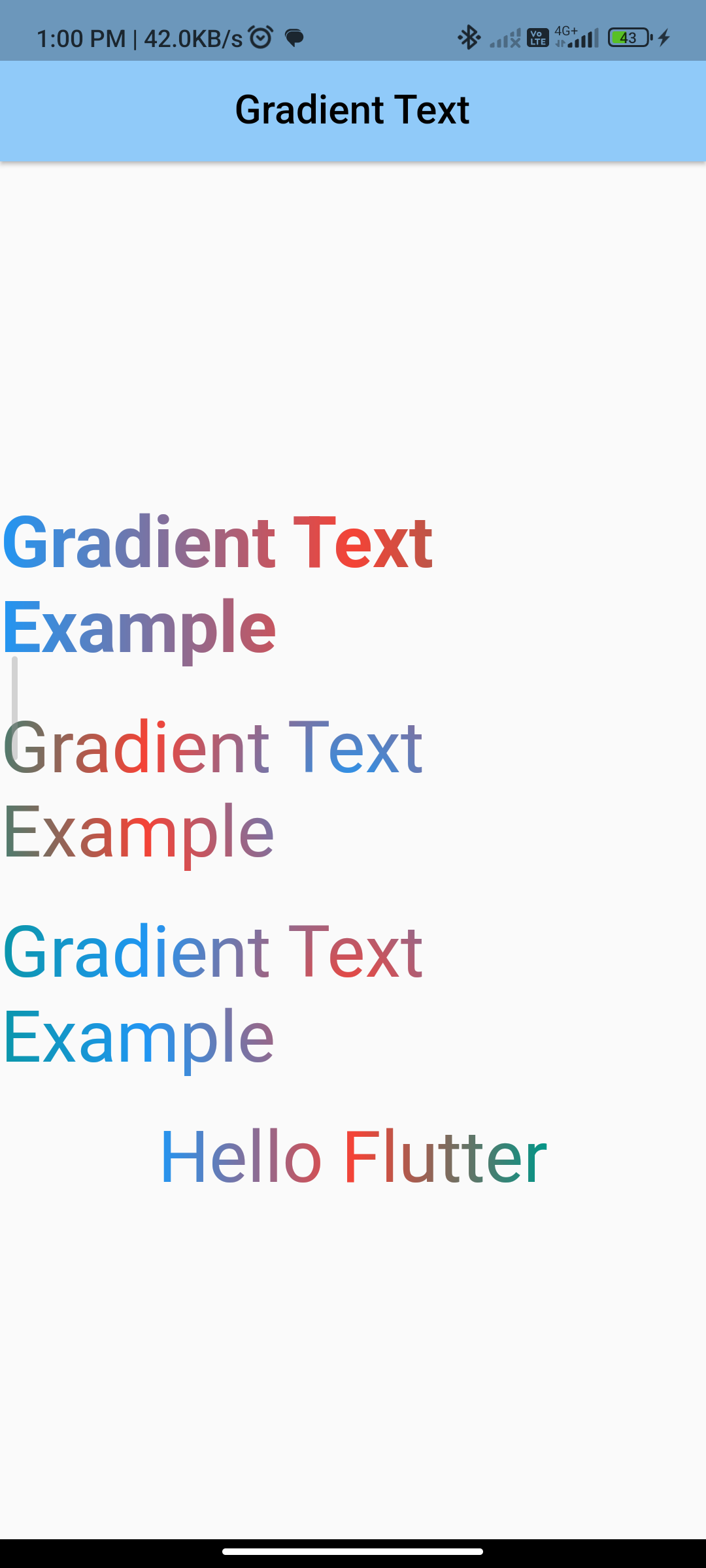
Conclusion:
By following these steps, you can easily implement gradient text in Flutter using the simple_gradient_text
package. This allows you to add colorful and visually appealing text to your app’s UI.