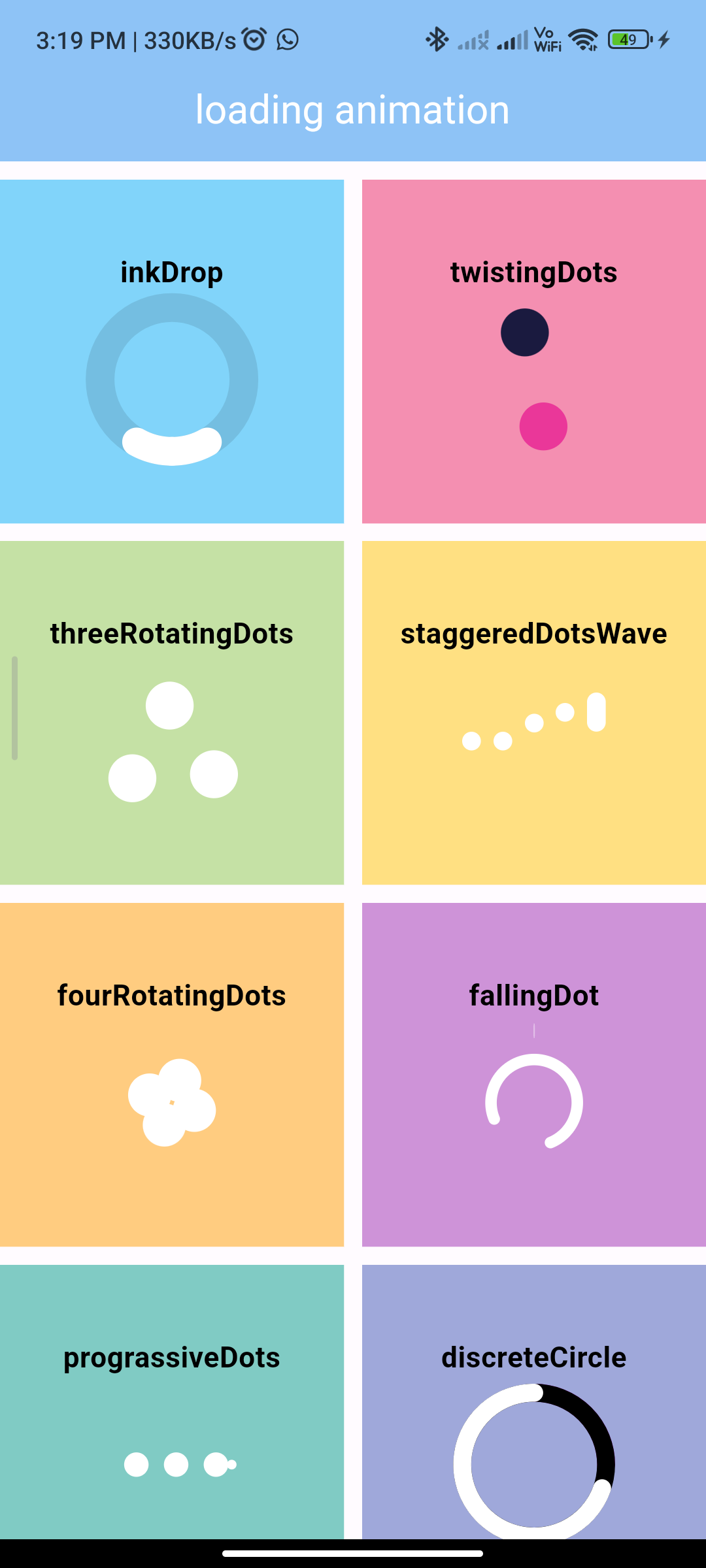
Introduction:
Loading animations are essential UI elements in mobile apps to indicate to users that a process is in progress. In Flutter, you can easily implement loading animations using various packages available. In this guide, we’ll walk you through the process of integrating a loading animation into your Flutter app using the loading_animation
package.
Content:
Step 1: Install the loading_animation
Package
Add the loading_animation
package to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
loading_animation: ^1.0.0
Then, run the following command in your terminal to fetch the package:
flutter pub get
Step 2: Import the Required Libraries
In the Dart file where you want to implement the loading animation, import the necessary libraries:
import 'package:flutter/material.dart';
import 'package:loading_animation/loading_animation.dart';
Step 3: Implement the Loading Animation Widget
Inside the build method of your widget, create a LoadingAnimation widget:
LoadingAnimation(
size: 50, // Adjust the size of the loading animation as needed
),
Step 4: Customize the Loading Animation
Customize the loading animation by adjusting parameters such as size, color, duration, etc., according to your app’s design requirements.
Step 5: Integrate the Loading Animation into Your App
Place the LoadingAnimation widget in the desired location within your app’s UI hierarchy.
Step 6: Test the Loading Animation
Save your changes and run the app on a device or emulator. Verify that the loading animation is displayed correctly.
Step 7: Run the Application
Run your Flutter application on a device or emulator using the following command in your terminal:
flutter run
Once the application is launched, you should see the loading animation displayed on the screen, indicating that some process is in progress.
Sample Code:
// ignore_for_file: prefer_const_constructors, camel_case_types
import 'package:flutter/material.dart';
import 'package:loading_animation_widget/loading_animation_widget.dart';
const Color _kAppColor = Color(0xFFFDDE6F);
const double _kSize = 70;
class loadingAnimation extends StatefulWidget {
const loadingAnimation({Key? key}) : super(key: key);
@override
_loadingAnimationState createState() => _loadingAnimationState();
}
class _loadingAnimationState extends State<loadingAnimation> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
elevation: 1,
backgroundColor: Colors.blue[200],
centerTitle: true,
title: Text(
"loading animation",
style: TextStyle(color: Colors.white),
),
),
body: MyGridView(),
);
}
}
class AppBody {
final String title;
final Widget widget;
AppBody(
this.title,
this.widget,
);
}
final listOfAnimations = <AppBody>[
AppBody(
'inkDrop',
LoadingAnimationWidget.inkDrop(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'twistingDots',
LoadingAnimationWidget.twistingDots(
leftDotColor: const Color(0xFF1A1A3F),
rightDotColor: const Color(0xFFEA3799),
size: _kSize,
),
),
AppBody(
'threeRotatingDots',
LoadingAnimationWidget.threeRotatingDots(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'staggeredDotsWave',
LoadingAnimationWidget.staggeredDotsWave(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'fourRotatingDots',
LoadingAnimationWidget.fourRotatingDots(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'fallingDot',
LoadingAnimationWidget.fallingDot(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'prograssiveDots',
LoadingAnimationWidget.prograssiveDots(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'discreteCircle',
LoadingAnimationWidget.discreteCircle(color: Colors.white, size: _kSize, secondRingColor: Colors.black, thirdRingColor: Colors.purple),
),
AppBody(
'threeArchedCircle',
LoadingAnimationWidget.threeArchedCircle(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'bouncingBall',
LoadingAnimationWidget.bouncingBall(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'flickr',
LoadingAnimationWidget.flickr(
leftDotColor: const Color(0xFF0063DC),
rightDotColor: const Color(0xFFFF0084),
size: _kSize,
),
),
AppBody(
'hexagonDots',
LoadingAnimationWidget.hexagonDots(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'beat',
LoadingAnimationWidget.beat(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'twoRotatingArc',
LoadingAnimationWidget.twoRotatingArc(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'horizontalRotatingDots',
LoadingAnimationWidget.horizontalRotatingDots(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'newtonCradle',
LoadingAnimationWidget.newtonCradle(
color: Colors.white,
size: 2 * _kSize,
),
),
AppBody(
'stretchedDots',
LoadingAnimationWidget.stretchedDots(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'halfTriangleDot',
LoadingAnimationWidget.halfTriangleDot(
color: Colors.white,
size: _kSize,
),
),
AppBody(
'dotsTriangle',
LoadingAnimationWidget.dotsTriangle(
color: Colors.white,
size: _kSize,
),
),
];
final List<Color?> colorList = [
Colors.lightBlue[200],
Colors.pink[200],
Colors.lightGreen[200],
Colors.amber[200],
Colors.orange[200],
Colors.purple[200],
Colors.teal[200],
Colors.indigo[200],
Colors.deepOrange[200],
Colors.pink[200],
Colors.lightBlue[200],
Colors.pink[200],
Colors.lightGreen[200],
Colors.amber[200],
Colors.orange[200],
Colors.purple[200],
Colors.teal[200],
Colors.indigo[200],
Colors.deepOrange[200],
Colors.pink[200],
Colors.lightBlue[200],
Colors.pink[200],
Colors.lightGreen[200],
Colors.amber[200],
Colors.orange[200],
Colors.purple[200],
Colors.teal[200],
Colors.indigo[200],
Colors.deepOrange[200],
Colors.pink[200],
];
class MyGridView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GridView.builder(
itemCount: listOfAnimations.length,
padding: EdgeInsets.symmetric(vertical: 10),
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 2,
crossAxisSpacing: 10.0,
mainAxisSpacing: 10.0,
),
itemBuilder: (BuildContext context, int index) {
final animation = listOfAnimations[index];
return GridTile(
child: Container(
color: colorList[index],
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
animation.title,
textAlign: TextAlign.center,
style: TextStyle(fontSize: 16, color: Colors.black, fontWeight: FontWeight.bold),
),
SizedBox(
height: 8,
),
Container(
child: animation.widget,
)
],
),
),
),
);
},
);
}
}
Output:
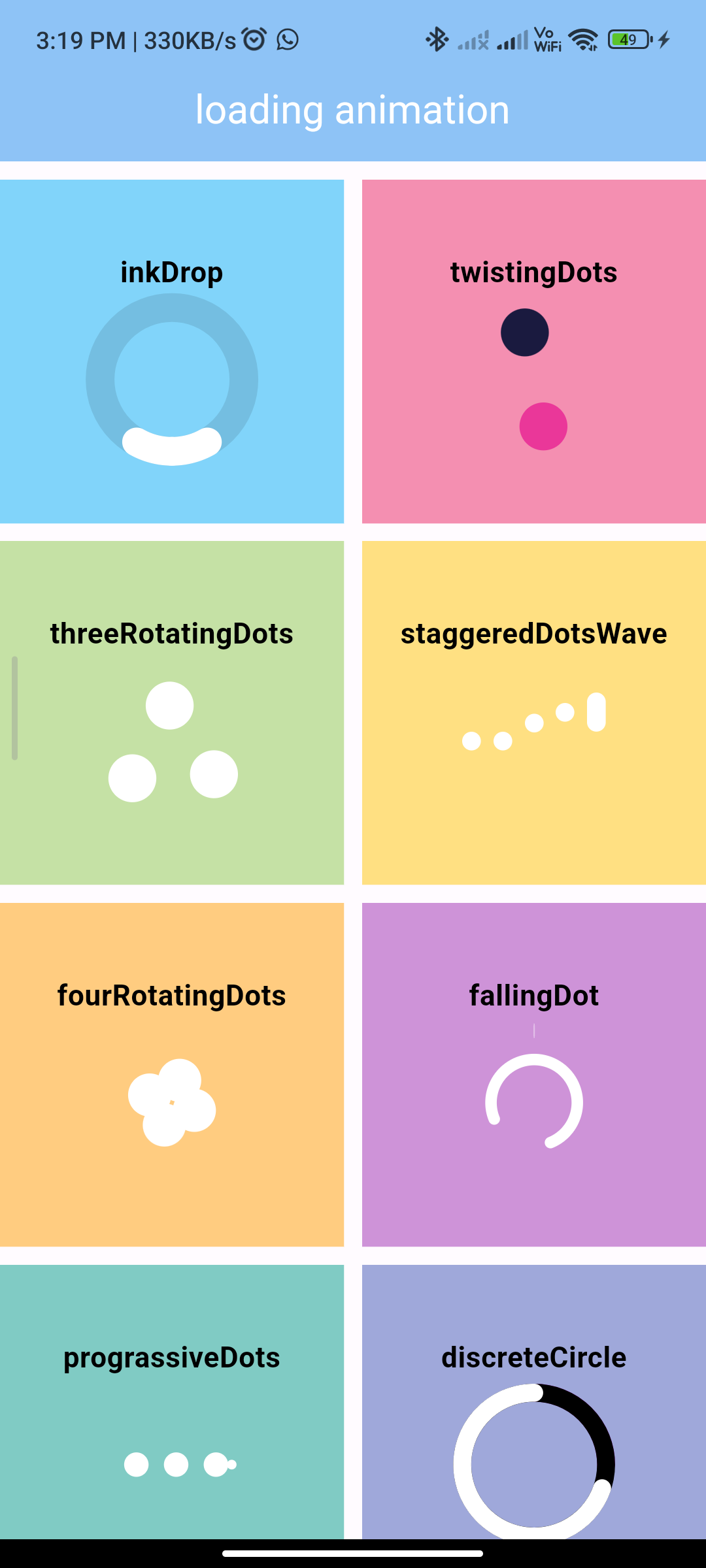
Conclusion:
Congratulations! You have successfully implemented a loading animation in your Flutter app using the loading_animation
package. Loading animations are crucial for providing feedback to users during asynchronous operations, enhancing the overall user experience of your app.