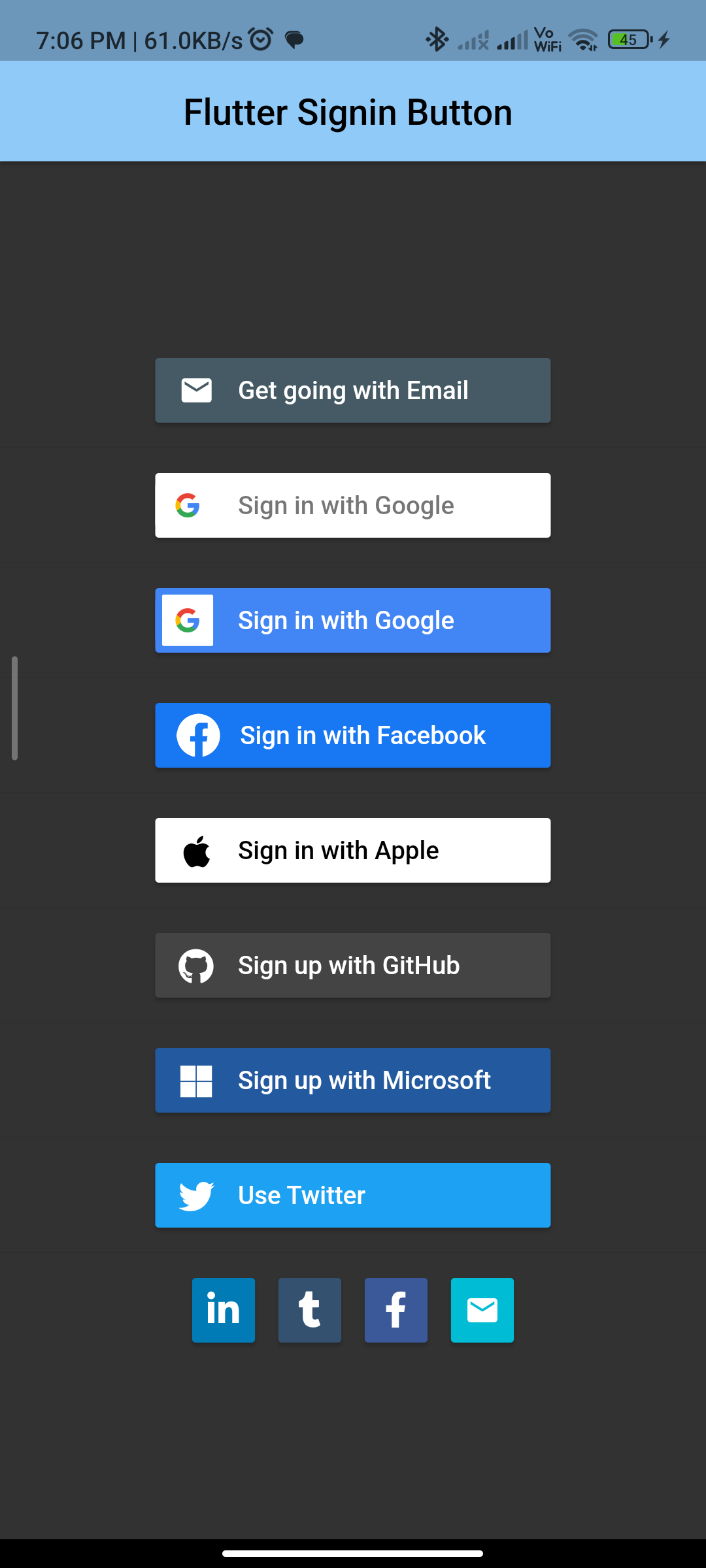
Introduction:
Implementing sign-in buttons in Flutter provides users with an easy way to authenticate and access your app’s features. The flutter_signin_button
package offers a variety of sign-in buttons for popular services like Google, Facebook, Twitter, and more. This tutorial will guide you through implementing sign-in buttons in Flutter using the flutter_signin_button
package.
Content:
Step 1: Add the dependency:
Add the flutter_signin_button
package to your pubspec.yaml
file:
dependencies:
flutter_signin_button: ^2.1.0
Save the file and run flutter pub get
to install the package.
Step 2: Import the package:
Import the flutter_signin_button
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:flutter_signin_button/flutter_signin_button.dart';
Step 3: Create sign-in buttons:
Use the SignInButton
widget to create sign-in buttons for different services. For example, to create a Google sign-in button:
SignInButton(
Buttons.Google,
onPressed: () {
// Handle Google sign-in
},
),
You can create buttons for other services like Facebook, Twitter, GitHub, etc., by replacing Buttons.Google
with the appropriate button type.
Step 4: Use the sign-in buttons in your UI:
Place the sign-in buttons in your app’s UI where you want them to appear:
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Sign In'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
SignInButton(
Buttons.Google,
onPressed: () {
// Handle Google sign-in
},
),
SignInButton(
Buttons.Facebook,
onPressed: () {
// Handle Facebook sign-in
},
),
SignInButton(
Buttons.Twitter,
onPressed: () {
// Handle Twitter sign-in
},
),
],
),
),
);
}
Step 5: Customize the buttons (optional):
You can customize the appearance of the sign-in buttons by using the mini
and text
parameters. For example, to create a mini Google sign-in button with text:
SignInButton(
Buttons.Google,
mini: true,
text: "Sign in with Google",
onPressed: () {
// Handle Google sign-in
},
),
Sample Code:
import 'package:flutter/material.dart';
import 'package:flutter_signin_button/flutter_signin_button.dart';
class flutterSignInButton extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData.light(),
home: Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Flutter Signin Button ",
style: TextStyle(color: Colors.black),
),
backgroundColor: Colors.blue[200],
),
backgroundColor: const Color.fromRGBO(50, 50, 50, 1.0),
body: SignInPage(),
),
debugShowCheckedModeBanner: false,
);
}
}
class SignInPage extends StatelessWidget {
/// Show a simple "___ Button Pressed" indicator
void _showButtonPressDialog(BuildContext context, String provider) {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text('$provider Button Pressed!'),
backgroundColor: Colors.black26,
duration: const Duration(milliseconds: 400),
));
}
/// Normally the signin buttons should be contained in the SignInPage
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SignInButtonBuilder(
text: 'Get going with Email',
icon: Icons.email,
onPressed: () {
_showButtonPressDialog(context, 'Email');
},
backgroundColor: Colors.blueGrey[700]!,
width: 220.0,
),
const Divider(),
SignInButton(
Buttons.Google,
onPressed: () {
_showButtonPressDialog(context, 'Google');
},
),
const Divider(),
SignInButton(
Buttons.GoogleDark,
onPressed: () {
_showButtonPressDialog(context, 'Google (dark)');
},
),
const Divider(),
SignInButton(
Buttons.FacebookNew,
onPressed: () {
_showButtonPressDialog(context, 'FacebookNew');
},
),
const Divider(),
SignInButton(
Buttons.Apple,
onPressed: () {
_showButtonPressDialog(context, 'Apple');
},
),
const Divider(),
SignInButton(
Buttons.GitHub,
text: 'Sign up with GitHub',
onPressed: () {
_showButtonPressDialog(context, 'Github');
},
),
const Divider(),
SignInButton(
Buttons.Microsoft,
text: 'Sign up with Microsoft ',
onPressed: () {
_showButtonPressDialog(context, 'Microsoft ');
},
),
const Divider(),
SignInButton(
Buttons.Twitter,
text: 'Use Twitter',
onPressed: () {
_showButtonPressDialog(context, 'Twitter');
},
),
const Divider(),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SignInButton(
Buttons.LinkedIn,
mini: true,
onPressed: () {
_showButtonPressDialog(context, 'LinkedIn (mini)');
},
),
SignInButton(
Buttons.Tumblr,
mini: true,
onPressed: () {
_showButtonPressDialog(context, 'Tumblr (mini)');
},
),
SignInButton(
Buttons.Facebook,
mini: true,
onPressed: () {
_showButtonPressDialog(context, 'Facebook (mini)');
},
),
SignInButtonBuilder(
icon: Icons.email,
text: 'Ignored for mini button',
mini: true,
onPressed: () {
_showButtonPressDialog(context, 'Email (mini)');
},
backgroundColor: Colors.cyan,
),
],
),
],
),
);
}
}
Output:
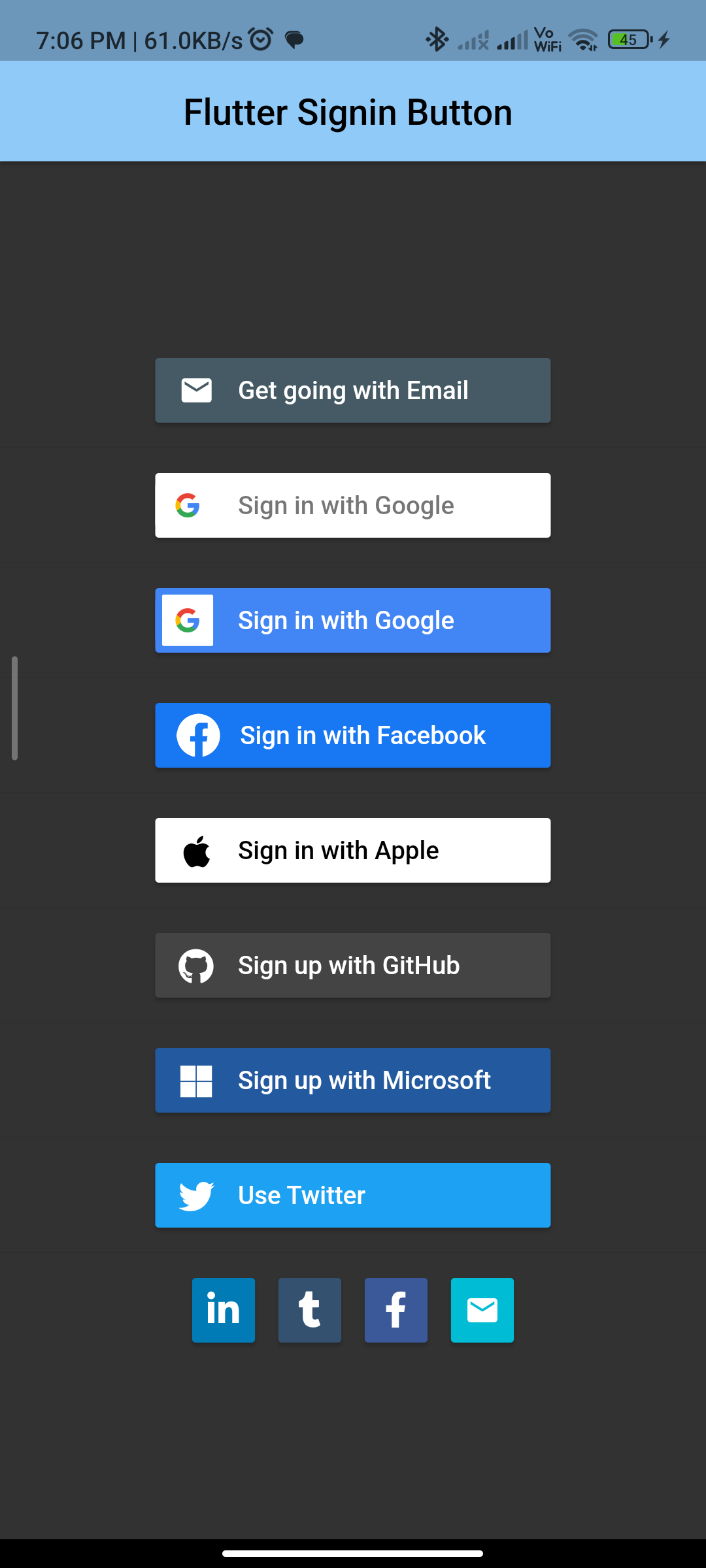