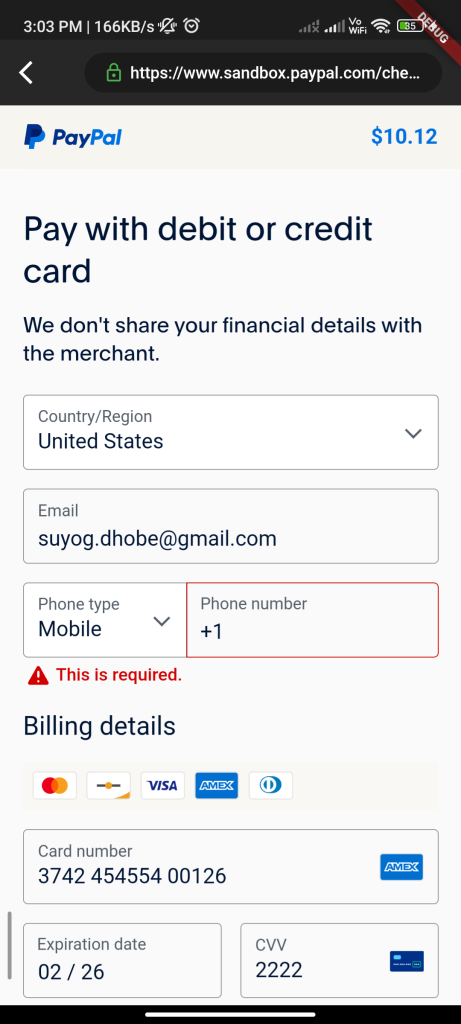
Introduction
The flutter_paypal
package provides an easy way to integrate PayPal payment functionality into your Flutter app. This guide will show you how to use this package to implement PayPal payments in your Flutter app.
Content
1.Add the flutter_paypal
dependency:
Open your pubspec.yaml
file and add the flutter_paypal
dependency.
dependencies:
flutter_paypal: ^latest_version
Run flutter pub get
to install the package
2.Import the package:
Import the flutter_paypal
package in your Dart file.
import 'package:flutter_paypal/flutter_paypal.dart';
3.Set up PayPal:
Initialize PayPal with your client ID and secret. You can obtain these credentials by creating a PayPal developer account and registering your app.
void initPayPal() {
PayPal.init(
clientId: 'YOUR_CLIENT_ID',
secret: 'YOUR_SECRET',
environment: PayPalEnvironment.sandbox, // Or PayPalEnvironment.production for production environment
);
}
4.Make a payment:
Use the PayPal.requestPayment
method to initiate a PayPal payment. Provide the amount, currency, and description for the payment.
void makePayment() async {
PayPalPaymentDetails paymentDetails = PayPalPaymentDetails(
amount: '10.00',
currency: 'USD',
description: 'Payment for your order',
);
PayPalPaymentResponse paymentResponse = await PayPal.requestPayment(
paymentDetails: paymentDetails,
);
if (paymentResponse.status == PayPalStatus.success) {
// Payment successful
} else {
// Payment failed
}
}
5.Handle payment response:
Check the status of the payment response to determine if the payment was successful or failed.
6.Handle errors:
Implement error handling to manage any issues that may occur during the payment process
Sample Code
import 'package:flutter/material.dart';
import 'package:flutter_paypal/flutter_paypal.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Paypal',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(title: 'Flutter Paypal Example'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: TextButton(
onPressed: () => {
Navigator.of(context).push(
MaterialPageRoute(
builder: (BuildContext context) => UsePaypal(
sandboxMode: true,
clientId: "AW1TdvpSGbIM5iP4HJNI5TyTmwpY9Gv9dYw8_8yW5lYIbCqf326vrkrp0ce9TAqjEGMHiV3OqJM_aRT0",
secretKey: "EHHtTDjnmTZATYBPiGzZC_AZUfMpMAzj2VZUeqlFUrRJA_C0pQNCxDccB5qoRQSEdcOnnKQhycuOWdP9",
returnURL: "https://samplesite.com/return",
cancelURL: "https://samplesite.com/cancel",
transactions: const [
{
"amount": {
"total": '10.12',
"currency": "USD",
"details": {"subtotal": '10.12', "shipping": '0', "shipping_discount": 0}
},
"description": "The payment transaction description.",
// "payment_options": {
// "allowed_payment_method":
// "INSTANT_FUNDING_SOURCE"
// },
"item_list": {
"items": [
{"name": "A demo product", "quantity": 1, "price": '10.12', "currency": "USD"}
],
// shipping address is not required though
"shipping_address": {
"recipient_name": "Jane Foster",
"line1": "Travis County",
"line2": "",
"city": "Austin",
"country_code": "US",
"postal_code": "73301",
"phone": "+00000000",
"state": "Texas"
},
}
}
],
note: "Contact us for any questions on your order.",
onSuccess: (Map params) async {
print("onSuccess: $params");
},
onError: (error) {
print("onError: $error");
},
onCancel: (params) {
print('cancelled: $params');
}),
),
)
},
child: const Text("Make Payment")),
));
}
}
Output
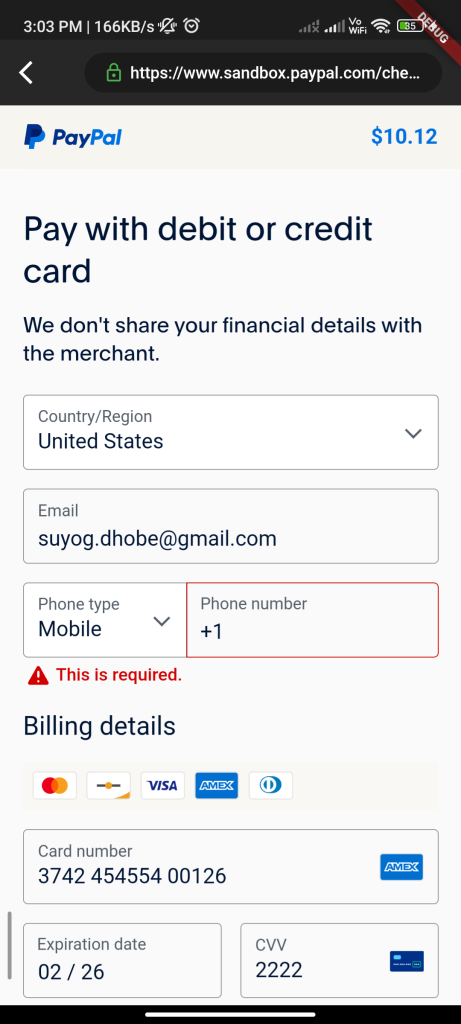
Conclusion
By following these steps, you can easily implement PayPal payment functionality in your Flutter app using the flutter_paypal
package. This allows you to accept payments from users using PayPal, providing a convenient and secure payment method for your app.