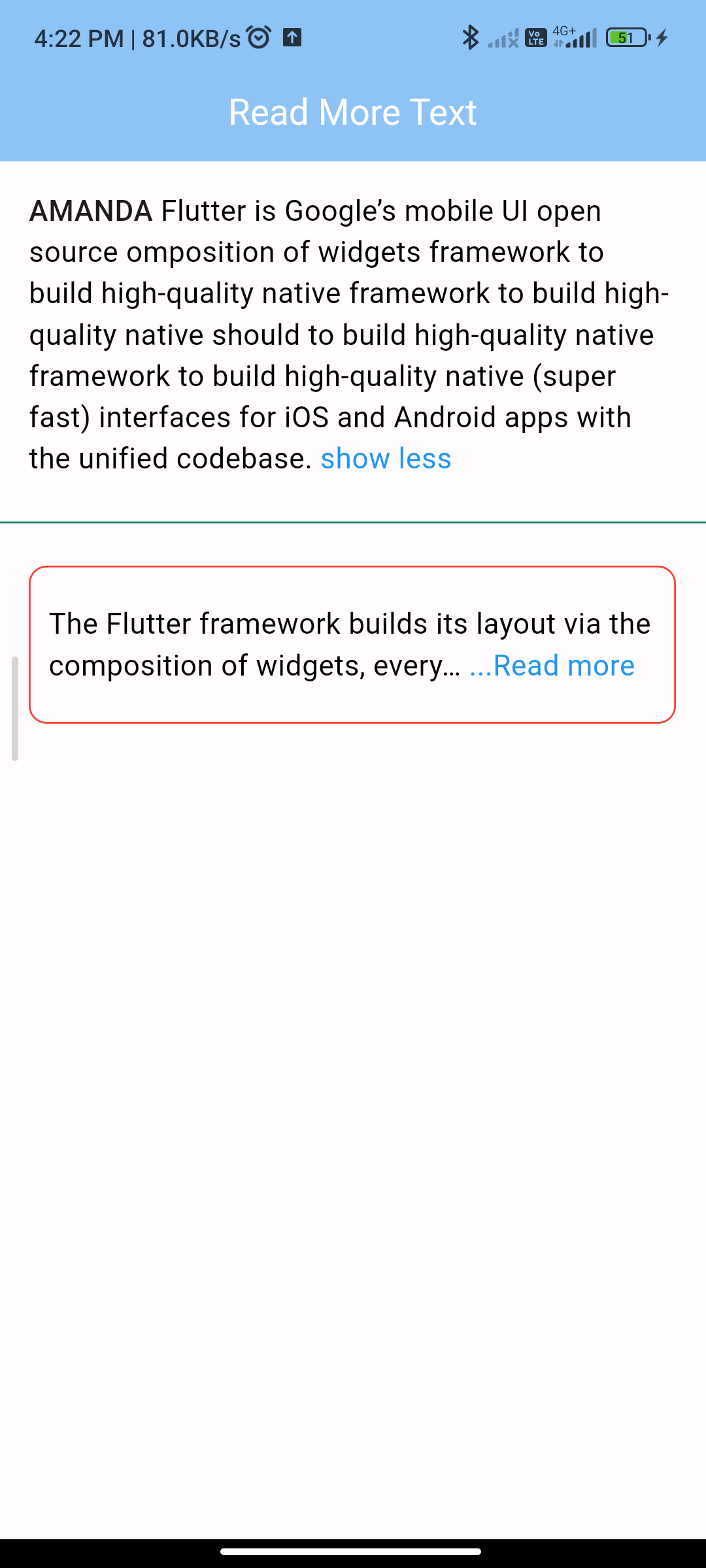
Introduction:
The `readmore` package provides a widget that allows you to collapse and expand text content with a “Read More” button. This functionality is useful when you have lengthy text content that you want to display in a condensed form initially. In this guide, you’ll learn how to integrate the `readmore` package into your Flutter app.
Content:
Step 1: Add Dependency Ensure you have the `readmore` package added to your `pubspec.yaml` file.
Run the following command in your terminal:
readmore: ^2.2.0
Run flutter pub get
to fetch the package.
Step 2: Import Dependencies
In your Dart file, import the necessary packages:
import 'package:flutter/material.dart';
import 'package:readmore/readmore.dart';
Step 3: Implement Read More Widget
Create a widget to display the text content with the “Read More” functionality. For example:
class ReadMoreWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Read More Example'),
),
body: Center(
child: Padding(
padding: EdgeInsets.all(16.0),
child: ReadMoreText(
'Lorem ipsum dolor sit amet, consectetur adipiscing elit. '
'Pellentesque sed est ac arcu aliquam vestibulum. '
'Suspendisse potenti. Vivamus sollicitudin, mauris vel aliquet '
'elementum, mauris velit efficitur odio, nec sollicitudin justo '
'ante ut nulla. Donec convallis tellus sit amet nulla porta, '
'vitae vestibulum nunc efficitur. Nullam in mi vel dui viverra '
'id convallis purus fermentum. '
'Duis dignissim quam vel neque rutrum, '
'quis tincidunt mauris cursus. '
'Nullam a augue ac orci congue pharetra. '
'Nulla et nisl vitae tellus sollicitudin sodales. '
'Nulla efficitur felis vel sapien laoreet tincidunt. '
'Praesent lobortis magna non convallis vehicula. '
'Integer lobortis metus vitae bibendum aliquet. '
'Donec ac mauris vel libero varius egestas. '
'Fusce semper orci nec nisl vestibulum, vel sollicitudin turpis '
'blandit. Mauris fringilla ipsum a tincidunt malesuada.'
'Donec ac mauris vel libero varius egestas. '
'Fusce semper orci nec nisl vestibulum, vel sollicitudin turpis '
'blandit. Mauris fringilla ipsum a tincidunt malesuada.'
'Donec ac mauris vel libero varius egestas. '
'Fusce semper orci nec nisl vestibulum, vel sollicitudin turpis '
'blandit. Mauris fringilla ipsum a tincidunt malesuada.',
trimLines: 3,
colorClickableText: Colors.blue,
trimMode: TrimMode.Line,
trimCollapsedText: '...Read more',
trimExpandedText: ' Show less',
style: TextStyle(fontSize: 16),
moreStyle: TextStyle(fontWeight: FontWeight.bold),
lessStyle: TextStyle(fontWeight: FontWeight.bold),
),
),
),
);
}
}
Step 4: Run the Application
Run your Flutter application, and navigate to the screen containing the ReadMore
widget. You should see the text content with the “Read More” functionality. Clicking on “Read More” will expand the text to display the full content.
Sample Code:
\
// ignore_for_file: prefer_const_constructors, prefer_const_literals_to_create_immutables
import 'package:flutter/material.dart';
import 'package:readmore/readmore.dart';
class ReadMore extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 1,
title: Text(
'Read More Text',
style: TextStyle(fontSize: 20, color: Colors.white),
),
backgroundColor: Colors.blue[200],
),
body: DefaultTextStyle.merge(
style: const TextStyle(
fontSize: 16.0,
//fontFamily: 'monospace',
),
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Padding(
key: const Key('showMore'),
padding: const EdgeInsets.all(16.0),
child: ReadMoreText(
'Flutter is Google’s mobile UI open source omposition of widgets framework to build high-quality native framework to build high-quality native should to build high-quality native framework to build high-quality native (super fast) interfaces for iOS and Android apps with the unified codebase.',
trimLines: 4,
preDataText: "AMANDA",
preDataTextStyle: TextStyle(fontWeight: FontWeight.w500),
style: TextStyle(color: Colors.black),
colorClickableText: Colors.blue,
trimMode: TrimMode.Line,
trimCollapsedText: '...Show more',
trimExpandedText: ' show less',
),
),
Divider(
color: const Color(0xFF167F67),
),
Padding(
padding: const EdgeInsets.all(16.0),
child: Container(
padding: EdgeInsets.symmetric(vertical: 20, horizontal: 10),
decoration: BoxDecoration(
border: Border.all(
color: Colors.red,
),
borderRadius: BorderRadius.circular(10)),
child: ReadMoreText(
'The Flutter framework builds its layout via the composition of widgets, everything that you construct programmatically is a widget and these are compiled together to create the user interface. ',
trimLines: 2,
style: TextStyle(color: Colors.black),
colorClickableText: Colors.blue,
trimMode: TrimMode.Line,
trimCollapsedText: '...Read more',
trimExpandedText: ' Less',
),
),
),
],
),
),
),
);
}
}
Output:
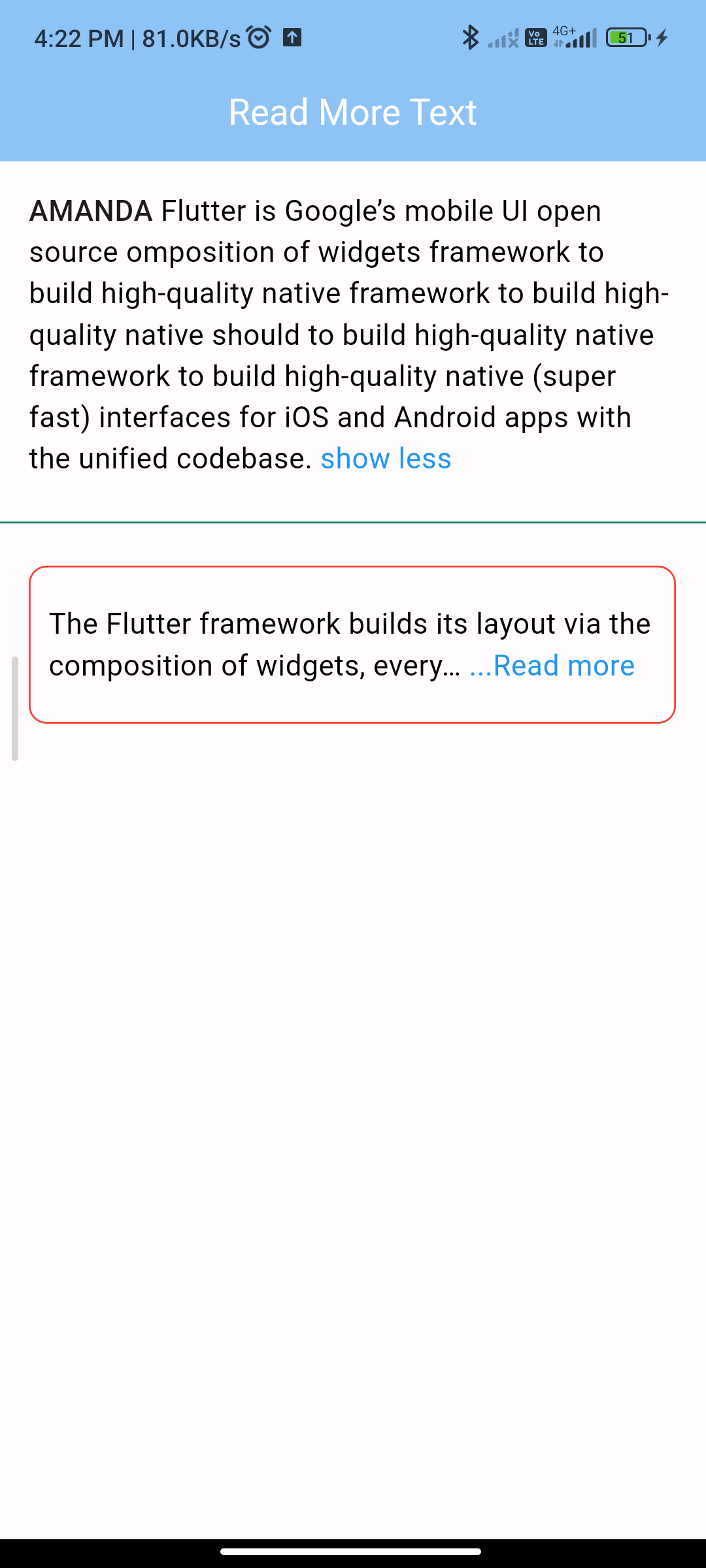
Conclusion:
Congratulations! You’ve successfully implemented the “Read More” functionality in your Flutter app using the readmore
package. Users can now easily expand and collapse lengthy text content as needed.