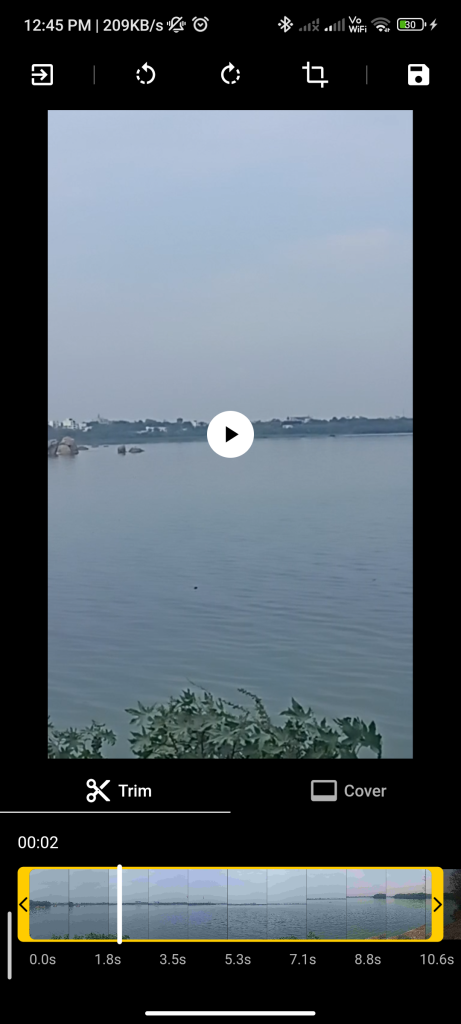
Introduction
The video_editor
package in Flutter allows you to perform various editing operations on videos, such as trimming, merging, adding filters, and more. This guide will show you how to use this package to implement a basic video editor in your Flutter app.
Content
1.Add the video_editor
dependency:
Open your pubspec.yaml
file and add the video_editor
dependency.
dependencies:
video_editor: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the video_editor
package in your Dart file.
import 'package:video_editor/video_editor.dart';
3.Initialize the VideoEditor
:
Create an instance of the VideoEditor
class to perform editing operations on videos.
VideoEditor videoEditor = VideoEditor();
4.Load a video:
Use the loadVideo
method to load a video file for editing.
await videoEditor.loadVideo('path_to_your_video.mp4');
5.Perform editing operations:
Use the various methods provided by the VideoEditor
class to perform editing operations on the loaded video. For example, you can trim the video using the trim
method.
await videoEditor.trim(start: Duration(seconds: 0), end: Duration(seconds: 10));
You can also merge multiple videos, add filters, adjust brightness, contrast, and more using the corresponding methods provided by the VideoEditor
class.
6.Export the edited video:
Once you have finished editing the video, use the export
method to export the edited video.
final VideoEditorController _controller = VideoEditorController.file(
File('/path/to/video.mp4'),
minDuration: const Duration(seconds: 1),
maxDuration: const Duration(seconds: 10),
);
@override
void initState() {
super.initState();
_controller.initialize().then((_) => setState(() {}));
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
/// Basic export video function
Future<void> exportVideo() async {
final config = VideoFFmpegVideoEditorConfig(_controller);
// Returns the generated command and the output path
final FFmpegVideoEditorExecute execute = await config.getExecuteConfig();
// ... handle the video exportation yourself, using ffmpeg_kit_flutter, your own video server, ...
}
/// Export the video as a GIF image
Future<void> exportGif() async {
final gifConfig = VideoFFmpegVideoEditorConfig(
_controller,
format: VideoExportFormat.gif,
);
// Returns the generated command and the output path
final FFmpegVideoEditorExecute gifExecute = await gifConfig.getExecuteConfig();
// ...
}
/// Export a video, with custom command (ultrafast preset + horizontal flip)
Future<void> exportMirroredVideo() async {
final mirrorConfig = VideoFFmpegVideoEditorConfig(
_controller,
name: 'mirror-video'
commandBuilder: (VideoFFmpegVideoEditorConfig config, String videoPath, String outputPath) {
final List<String> filters = config.getExportFilters();
filters.add('hflip'); // add horizontal flip
return '-i $videoPath ${config.filtersCmd(filters)} -preset ultrafast $outputPath';
},
);
// Returns the generated command and the output path
final FFmpegVideoEditorExecute mirrorExecute = await mirrorConfig.getExecuteConfig();
// ...
}
Sample Code
main.dart
import 'dart:io';
import 'dart:developer';
import 'package:ffmpeg_kit_flutter_min/ffmpeg_kit.dart';
import 'package:ffmpeg_kit_flutter_min/ffmpeg_kit_config.dart';
import 'package:ffmpeg_kit_flutter_min/ffmpeg_session.dart';
import 'package:ffmpeg_kit_flutter_min/return_code.dart';
import 'package:ffmpeg_kit_flutter_min/statistics.dart';
import 'package:flutter_one/views/export_result.dart';
import 'package:video_editor/video_editor.dart';
import 'package:flutter/material.dart';
import 'package:fraction/fraction.dart';
import 'package:video_editor/video_editor.dart';
// import 'package:video_editor_example/crop_page.dart';
// import 'package:video_editor_example/export_service.dart';
// import 'package:video_editor_example/widgets/export_result.dart';/
import 'package:flutter/material.dart';
import 'package:image_picker/image_picker.dart';
import 'package:video_editor/video_editor.dart';
void main() => runApp(
MaterialApp(
title: 'Flutter Video Editor Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.grey,
brightness: Brightness.dark,
tabBarTheme: const TabBarTheme(
indicator: UnderlineTabIndicator(
borderSide: BorderSide(color: Colors.white),
),
),
dividerColor: Colors.white,
),
home: const VideoEditorExample(),
),
);
class VideoEditorExample extends StatefulWidget {
const VideoEditorExample({super.key});
@override
State<VideoEditorExample> createState() => _VideoEditorExampleState();
}
class _VideoEditorExampleState extends State<VideoEditorExample> {
final ImagePicker _picker = ImagePicker();
void _pickVideo() async {
final XFile? file = await _picker.pickVideo(source: ImageSource.gallery);
if (mounted && file != null) {
Navigator.push(
context,
MaterialPageRoute<void>(
builder: (BuildContext context) => VideoEditor(file: File(file.path)),
),
);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text("Video Picker")),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text("Click on the button to select video"),
ElevatedButton(
onPressed: _pickVideo,
child: const Text("Pick Video From Gallery"),
),
],
),
),
);
}
}
//-------------------//
//VIDEO EDITOR SCREEN//
//-------------------//
class VideoEditor extends StatefulWidget {
const VideoEditor({super.key, required this.file});
final File file;
@override
State<VideoEditor> createState() => _VideoEditorState();
}
class _VideoEditorState extends State<VideoEditor> {
final _exportingProgress = ValueNotifier<double>(0.0);
final _isExporting = ValueNotifier<bool>(false);
final double height = 60;
late final VideoEditorController _controller = VideoEditorController.file(
widget.file,
minDuration: const Duration(seconds: 1),
maxDuration: const Duration(seconds: 10),
);
@override
void initState() {
super.initState();
_controller.initialize(aspectRatio: 9 / 16).then((_) => setState(() {})).catchError((error) {
// handle minumum duration bigger than video duration error
Navigator.pop(context);
}, test: (e) => e is VideoMinDurationError);
}
@override
void dispose() async {
_exportingProgress.dispose();
_isExporting.dispose();
_controller.dispose();
ExportService.dispose();
super.dispose();
}
void _showErrorSnackBar(String message) => ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text(message),
duration: const Duration(seconds: 1),
),
);
void _exportVideo() async {
_exportingProgress.value = 0;
_isExporting.value = true;
final config = VideoFFmpegVideoEditorConfig(
_controller,
// format: VideoExportFormat.gif,
// commandBuilder: (config, videoPath, outputPath) {
// final List<String> filters = config.getExportFilters();
// filters.add('hflip'); // add horizontal flip
// return '-i $videoPath ${config.filtersCmd(filters)} -preset ultrafast $outputPath';
// },
);
await ExportService.runFFmpegCommand(
await config.getExecuteConfig(),
onProgress: (stats) {
_exportingProgress.value = config.getFFmpegProgress(stats.getTime() as int);
},
onError: (e, s) => _showErrorSnackBar("Error on export video :("),
onCompleted: (file) {
_isExporting.value = false;
if (!mounted) return;
showDialog(
context: context,
builder: (_) => VideoResultPopup(video: file),
);
},
);
}
void _exportCover() async {
final config = CoverFFmpegVideoEditorConfig(_controller);
final execute = await config.getExecuteConfig();
if (execute == null) {
_showErrorSnackBar("Error on cover exportation initialization.");
return;
}
await ExportService.runFFmpegCommand(
execute,
onError: (e, s) => _showErrorSnackBar("Error on cover exportation :("),
onCompleted: (cover) {
if (!mounted) return;
showDialog(
context: context,
builder: (_) => CoverResultPopup(cover: cover),
);
},
);
}
@override
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: () async => false,
child: Scaffold(
backgroundColor: Colors.black,
body: _controller.initialized
? SafeArea(
child: Stack(
children: [
Column(
children: [
_topNavBar(),
Expanded(
child: DefaultTabController(
length: 2,
child: Column(
children: [
Expanded(
child: TabBarView(
physics: const NeverScrollableScrollPhysics(),
children: [
Stack(
alignment: Alignment.center,
children: [
CropGridViewer.preview(controller: _controller),
AnimatedBuilder(
animation: _controller.video,
builder: (_, __) => AnimatedOpacity(
opacity: _controller.isPlaying ? 0 : 1,
duration: kThemeAnimationDuration,
child: GestureDetector(
onTap: _controller.video.play,
child: Container(
width: 40,
height: 40,
decoration: const BoxDecoration(
color: Colors.white,
shape: BoxShape.circle,
),
child: const Icon(
Icons.play_arrow,
color: Colors.black,
),
),
),
),
),
],
),
CoverViewer(controller: _controller)
],
),
),
Container(
height: 200,
margin: const EdgeInsets.only(top: 10),
child: Column(
children: [
TabBar(
tabs: [
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: const [Padding(padding: EdgeInsets.all(5), child: Icon(Icons.content_cut)), Text('Trim')]),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: const [Padding(padding: EdgeInsets.all(5), child: Icon(Icons.video_label)), Text('Cover')],
),
],
),
Expanded(
child: TabBarView(
physics: const NeverScrollableScrollPhysics(),
children: [
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: _trimSlider(),
),
_coverSelection(),
],
),
),
],
),
),
ValueListenableBuilder(
valueListenable: _isExporting,
builder: (_, bool export, Widget? child) => AnimatedSize(
duration: kThemeAnimationDuration,
child: export ? child : null,
),
child: AlertDialog(
title: ValueListenableBuilder(
valueListenable: _exportingProgress,
builder: (_, double value, __) => Text(
"Exporting video ${(value * 100).ceil()}%",
style: const TextStyle(fontSize: 12),
),
),
),
)
],
),
),
)
],
)
],
),
)
: const Center(child: CircularProgressIndicator()),
),
);
}
Widget _topNavBar() {
return SafeArea(
child: SizedBox(
height: height,
child: Row(
children: [
Expanded(
child: IconButton(
onPressed: () => Navigator.of(context).pop(),
icon: const Icon(Icons.exit_to_app),
tooltip: 'Leave editor',
),
),
const VerticalDivider(endIndent: 22, indent: 22),
Expanded(
child: IconButton(
onPressed: () => _controller.rotate90Degrees(RotateDirection.left),
icon: const Icon(Icons.rotate_left),
tooltip: 'Rotate unclockwise',
),
),
Expanded(
child: IconButton(
onPressed: () => _controller.rotate90Degrees(RotateDirection.right),
icon: const Icon(Icons.rotate_right),
tooltip: 'Rotate clockwise',
),
),
Expanded(
child: IconButton(
onPressed: () => Navigator.push(
context,
MaterialPageRoute<void>(
builder: (context) => CropPage(controller: _controller),
),
),
icon: const Icon(Icons.crop),
tooltip: 'Open crop screen',
),
),
const VerticalDivider(endIndent: 22, indent: 22),
Expanded(
child: PopupMenuButton(
tooltip: 'Open export menu',
icon: const Icon(Icons.save),
itemBuilder: (context) => [
PopupMenuItem(
onTap: _exportCover,
child: const Text('Export cover'),
),
PopupMenuItem(
onTap: _exportVideo,
child: const Text('Export video'),
),
],
),
),
],
),
),
);
}
String formatter(Duration duration) =>
[duration.inMinutes.remainder(60).toString().padLeft(2, '0'), duration.inSeconds.remainder(60).toString().padLeft(2, '0')].join(":");
List<Widget> _trimSlider() {
return [
AnimatedBuilder(
animation: Listenable.merge([
_controller,
_controller.video,
]),
builder: (_, __) {
final int duration = _controller.videoDuration.inSeconds;
final double pos = _controller.trimPosition * duration;
return Padding(
padding: EdgeInsets.symmetric(horizontal: height / 4),
child: Row(children: [
Text(formatter(Duration(seconds: pos.toInt()))),
const Expanded(child: SizedBox()),
AnimatedOpacity(
opacity: _controller.isTrimming ? 1 : 0,
duration: kThemeAnimationDuration,
child: Row(mainAxisSize: MainAxisSize.min, children: [
Text(formatter(_controller.startTrim)),
const SizedBox(width: 10),
Text(formatter(_controller.endTrim)),
]),
),
]),
);
},
),
Container(
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.symmetric(vertical: height / 4),
child: TrimSlider(
controller: _controller,
height: height,
horizontalMargin: height / 4,
child: TrimTimeline(
controller: _controller,
padding: const EdgeInsets.only(top: 10),
),
),
)
];
}
Widget _coverSelection() {
return SingleChildScrollView(
child: Center(
child: Container(
margin: const EdgeInsets.all(15),
child: CoverSelection(
controller: _controller,
size: height + 10,
quantity: 8,
selectedCoverBuilder: (cover, size) {
return Stack(
alignment: Alignment.center,
children: [
cover,
Icon(
Icons.check_circle,
color: const CoverSelectionStyle().selectedBorderColor,
)
],
);
},
),
),
),
);
}
}
class ExportService {
static Future<void> dispose() async {
final executions = await FFmpegKit.listSessions();
if (executions.isNotEmpty) await FFmpegKit.cancel();
}
static Future<FFmpegSession> runFFmpegCommand(
FFmpegVideoEditorExecute execute, {
required void Function(File file) onCompleted,
void Function(Object, StackTrace)? onError,
void Function(Statistics)? onProgress,
}) {
log('FFmpeg start process with command = ${execute.command}');
return FFmpegKit.executeAsync(
execute.command,
(session) async {
final state = FFmpegKitConfig.sessionStateToString(await session.getState());
final code = await session.getReturnCode();
if (ReturnCode.isSuccess(code)) {
onCompleted(File(execute.outputPath));
} else {
if (onError != null) {
onError(
Exception('FFmpeg process exited with state $state and return code $code.\n${await session.getOutput()}'),
StackTrace.current,
);
}
return;
}
},
null,
onProgress,
);
}
}
class CropPage extends StatelessWidget {
const CropPage({super.key, required this.controller});
final VideoEditorController controller;
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black,
body: SafeArea(
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 30),
child: Column(children: [
Row(children: [
Expanded(
child: IconButton(
onPressed: () => controller.rotate90Degrees(RotateDirection.left),
icon: const Icon(Icons.rotate_left),
),
),
Expanded(
child: IconButton(
onPressed: () => controller.rotate90Degrees(RotateDirection.right),
icon: const Icon(Icons.rotate_right),
),
)
]),
const SizedBox(height: 15),
Expanded(
child: CropGridViewer.edit(
controller: controller,
rotateCropArea: false,
margin: const EdgeInsets.symmetric(horizontal: 20),
),
),
const SizedBox(height: 15),
Row(crossAxisAlignment: CrossAxisAlignment.end, children: [
Expanded(
flex: 2,
child: IconButton(
onPressed: () => Navigator.pop(context),
icon: const Center(
child: Text(
"cancel",
style: TextStyle(fontWeight: FontWeight.bold),
),
),
),
),
Expanded(
flex: 4,
child: AnimatedBuilder(
animation: controller,
builder: (_, __) => Column(
children: [
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
IconButton(
onPressed: () =>
controller.preferredCropAspectRatio = controller.preferredCropAspectRatio?.toFraction().inverse().toDouble(),
icon: controller.preferredCropAspectRatio != null && controller.preferredCropAspectRatio! < 1
? const Icon(Icons.panorama_vertical_select_rounded)
: const Icon(Icons.panorama_vertical_rounded),
),
IconButton(
onPressed: () =>
controller.preferredCropAspectRatio = controller.preferredCropAspectRatio?.toFraction().inverse().toDouble(),
icon: controller.preferredCropAspectRatio != null && controller.preferredCropAspectRatio! > 1
? const Icon(Icons.panorama_horizontal_select_rounded)
: const Icon(Icons.panorama_horizontal_rounded),
),
],
),
Row(
children: [
_buildCropButton(context, null),
_buildCropButton(context, 1.toFraction()),
_buildCropButton(context, Fraction.fromString("9/16")),
_buildCropButton(context, Fraction.fromString("3/4")),
],
)
],
),
),
),
Expanded(
flex: 2,
child: IconButton(
onPressed: () {
// WAY 1: validate crop parameters set in the crop view
controller.applyCacheCrop();
// WAY 2: update manually with Offset values
// controller.updateCrop(const Offset(0.2, 0.2), const Offset(0.8, 0.8));
Navigator.pop(context);
},
icon: Center(
child: Text(
"done",
style: TextStyle(
color: const CropGridStyle().selectedBoundariesColor,
fontWeight: FontWeight.bold,
),
),
),
),
),
]),
]),
),
),
);
}
Widget _buildCropButton(BuildContext context, Fraction? f) {
if (controller.preferredCropAspectRatio != null && controller.preferredCropAspectRatio! > 1) f = f?.inverse();
return Flexible(
child: TextButton(
style: ElevatedButton.styleFrom(
elevation: 0,
backgroundColor: controller.preferredCropAspectRatio == f?.toDouble() ? Colors.grey.shade800 : null,
foregroundColor: controller.preferredCropAspectRatio == f?.toDouble() ? Colors.white : null,
textStyle: Theme.of(context).textTheme.bodySmall,
),
onPressed: () => controller.preferredCropAspectRatio = f?.toDouble(),
child: Text(f == null ? 'free' : '${f.numerator}:${f.denominator}'),
),
);
}
}
export_result.dart
import 'dart:io';
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:fraction/fraction.dart';
import 'package:path/path.dart' as path;
import 'package:video_player/video_player.dart';
Future<void> _getImageDimension(File file, {required Function(Size) onResult}) async {
var decodedImage = await decodeImageFromList(file.readAsBytesSync());
onResult(Size(decodedImage.width.toDouble(), decodedImage.height.toDouble()));
}
String _fileMBSize(File file) => ' ${(file.lengthSync() / (1024 * 1024)).toStringAsFixed(1)} MB';
class VideoResultPopup extends StatefulWidget {
const VideoResultPopup({super.key, required this.video});
final File video;
@override
State<VideoResultPopup> createState() => _VideoResultPopupState();
}
class _VideoResultPopupState extends State<VideoResultPopup> {
VideoPlayerController? _controller;
FileImage? _fileImage;
Size _fileDimension = Size.zero;
late final bool _isGif = path.extension(widget.video.path).toLowerCase() == ".gif";
late String _fileMbSize;
@override
void initState() {
super.initState();
if (_isGif) {
_getImageDimension(
widget.video,
onResult: (d) => setState(() => _fileDimension = d),
);
} else {
_controller = VideoPlayerController.file(widget.video);
_controller?.initialize().then((_) {
_fileDimension = _controller?.value.size ?? Size.zero;
setState(() {});
_controller?.play();
_controller?.setLooping(true);
});
}
_fileMbSize = _fileMBSize(widget.video);
}
@override
void dispose() {
if (_isGif) {
_fileImage?.evict();
} else {
_controller?.pause();
_controller?.dispose();
}
super.dispose();
}
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(30),
child: Center(
child: Stack(
alignment: Alignment.bottomLeft,
children: [
AspectRatio(
aspectRatio: _fileDimension.aspectRatio == 0 ? 1 : _fileDimension.aspectRatio,
child: _isGif ? Image.file(widget.video) : VideoPlayer(_controller!),
),
Positioned(
bottom: 0,
child: FileDescription(
description: {
'Video path': widget.video.path,
if (!_isGif) 'Video duration': '${((_controller?.value.duration.inMilliseconds ?? 0) / 1000).toStringAsFixed(2)}s',
'Video ratio': Fraction.fromDouble(_fileDimension.aspectRatio).reduce().toString(),
'Video dimension': _fileDimension.toString(),
'Video size': _fileMbSize,
},
),
),
],
),
),
);
}
}
class CoverResultPopup extends StatefulWidget {
const CoverResultPopup({super.key, required this.cover});
final File cover;
@override
State<CoverResultPopup> createState() => _CoverResultPopupState();
}
class _CoverResultPopupState extends State<CoverResultPopup> {
late final Uint8List _imagebytes = widget.cover.readAsBytesSync();
Size? _fileDimension;
late String _fileMbSize;
@override
void initState() {
super.initState();
_getImageDimension(
widget.cover,
onResult: (d) => setState(() => _fileDimension = d),
);
_fileMbSize = _fileMBSize(widget.cover);
}
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(30),
child: Center(
child: Stack(
children: [
Image.memory(_imagebytes),
Positioned(
bottom: 0,
child: FileDescription(
description: {
'Cover path': widget.cover.path,
'Cover ratio': Fraction.fromDouble(_fileDimension?.aspectRatio ?? 0).reduce().toString(),
'Cover dimension': _fileDimension.toString(),
'Cover size': _fileMbSize,
},
),
),
],
),
),
);
}
}
class FileDescription extends StatelessWidget {
const FileDescription({super.key, required this.description});
final Map<String, String> description;
@override
Widget build(BuildContext context) {
return DefaultTextStyle(
style: const TextStyle(fontSize: 11),
child: Container(
width: MediaQuery.of(context).size.width - 60,
padding: const EdgeInsets.all(10),
color: Colors.black.withOpacity(0.5),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: description.entries
.map(
(entry) => Text.rich(
TextSpan(
children: [
TextSpan(
text: '${entry.key}: ',
style: const TextStyle(fontSize: 11),
),
TextSpan(
text: entry.value,
style: TextStyle(
fontSize: 10,
color: Colors.white.withOpacity(0.8),
),
),
],
),
),
)
.toList(),
),
),
);
}
}
Output
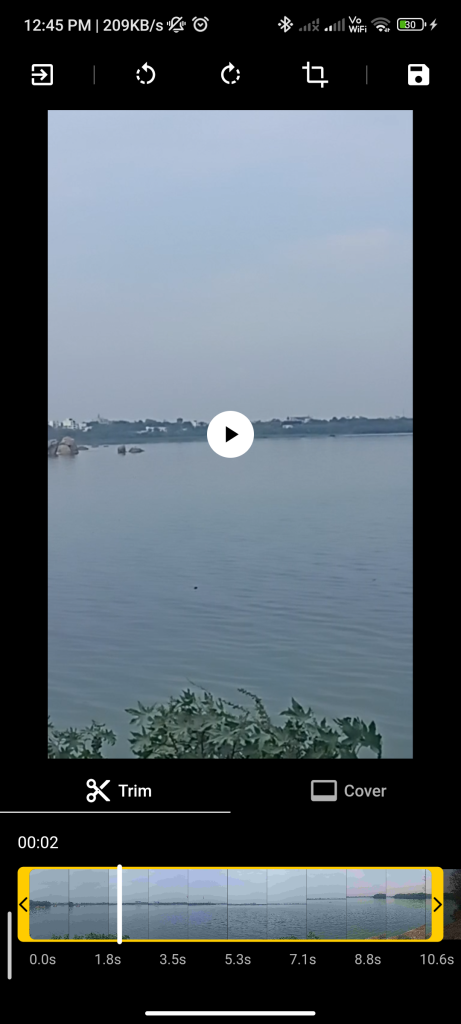
Conclusion
By following these steps, you can implement a basic video editor in your Flutter app using the video_editor
package. This allows you to perform various editing operations on videos to create custom video content in your app.