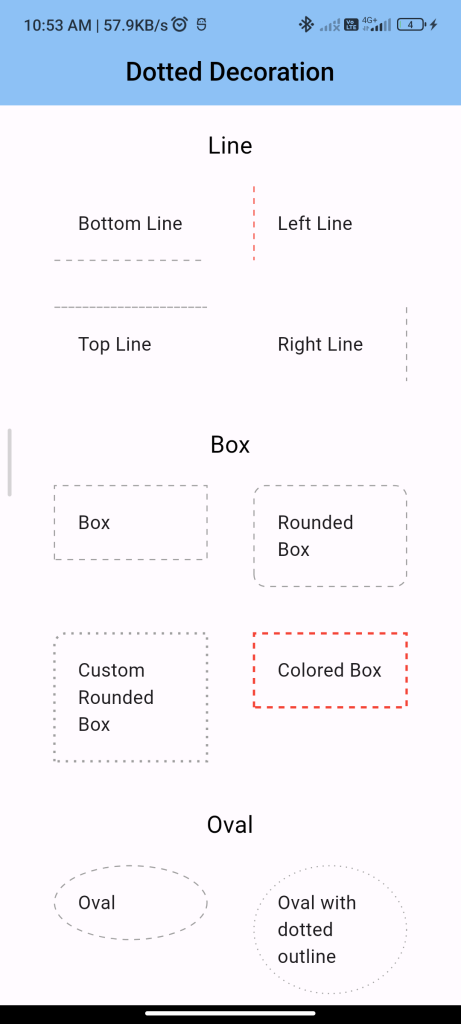
Introduction:
Implementing a dotted line in Flutter can be useful for indicating boundaries or dividing sections in your app. The dotted_decoration
package provides a simple way to implement a dotted line in Flutter. This tutorial will guide you through implementing a dotted line using the dotted_decoration
package.
Content:
Step 1: Add the dependency:
Add the dotted_decoration
package to your pubspec.yaml
file:
dependencies:
dotted_decoration: ^1.0.0
Save the file and run flutter pub get
to install the package.
Step 2: Import the package: Import the dotted_decoration
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:dotted_decoration/dotted_decoration.dart';
Step 3: Create a DottedLine widget:
Create a Container
widget with a BoxDecoration
that uses the DottedDecoration
class to create a dotted line:
class DottedLineScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Dotted Line Example'),
),
body: Center(
child: Container(
height: 1,
width: 200,
decoration: DottedDecoration(
shape: Shape.line,
linePosition: LinePosition.bottom,
lineThickness: 1.0,
dash: <int>[3, 2],
color: Colors.grey,
),
),
),
);
}
}
In this example, the DottedDecoration
class is used to create a horizontal dotted line at the bottom of a Container
widget with a thickness of 1.0 and a dash pattern of [3, 2]
.
Step 4: Run the app:
Run your Flutter app to see the dotted line in action. You should see a horizontal dotted line at the bottom of the screen.
Sample Code:
import 'package:dotted_decoration/dotted_decoration.dart';
import 'package:flutter/material.dart';
class DottedLine extends StatefulWidget {
DottedLine({Key? key}) : super(key: key);
@override
_DottedLineState createState() => _DottedLineState();
}
class _DottedLineState extends State<DottedLine> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Dotted Decoration",
style: TextStyle(fontSize: 22, color: Colors.black, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
body: SingleChildScrollView(
child: Container(
padding: EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
_getDecorationWidget('Line', <Decoration>[
DottedDecoration(shape: Shape.line, linePosition: LinePosition.bottom),
DottedDecoration(shape: Shape.line, linePosition: LinePosition.left, color: Colors.red),
DottedDecoration(shape: Shape.line, linePosition: LinePosition.top, dash: <int>[5, 1]),
DottedDecoration(shape: Shape.line, linePosition: LinePosition.right)
], <String>[
'Bottom Line',
'Left Line',
'Top Line',
'Right Line'
]),
_getDecorationWidget('Box', <Decoration>[
DottedDecoration(shape: Shape.box),
DottedDecoration(
shape: Shape.box,
borderRadius: BorderRadius.circular(10),
),
DottedDecoration(
shape: Shape.box, borderRadius: BorderRadius.only(topLeft: Radius.circular(10)), dash: <int>[2, 5], strokeWidth: 2),
DottedDecoration(shape: Shape.box, color: Colors.red, strokeWidth: 2),
], <String>[
'Box',
'Rounded Box',
'Custom Rounded Box',
'Colored Box'
]),
_getDecorationWidget('Oval', <Decoration>[
DottedDecoration(shape: Shape.circle),
DottedDecoration(shape: Shape.circle, dash: <int>[1, 4]),
], <String>[
'Oval',
'Oval with dotted outline'
])
],
)),
),
);
}
_getDecorationWidget(String title, List<Decoration> decorations, List<String> decorationStrings) {
return Container(
padding: EdgeInsets.symmetric(vertical: 10),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(
title,
style: TextStyle(color: Colors.black, fontSize: 20),
),
Wrap(
children: List.generate(
decorations.length,
(index) => Container(
width: 130,
margin: EdgeInsets.all(20),
padding: EdgeInsets.all(20),
decoration: decorations[index],
child: Text(
decorationStrings[index],
style: TextStyle(
fontSize: 16,
),
),
)),
)
],
),
);
}
}
Output:
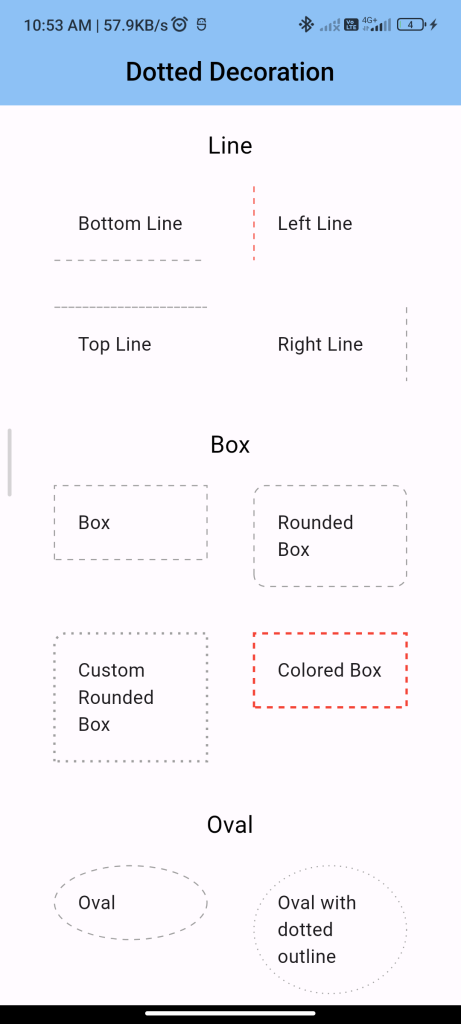
Conclusion:
By following these steps, you can easily implement a dotted line in Flutter using the dotted_decoration
package. This allows you to add visually appealing dotted lines to your app’s UI.