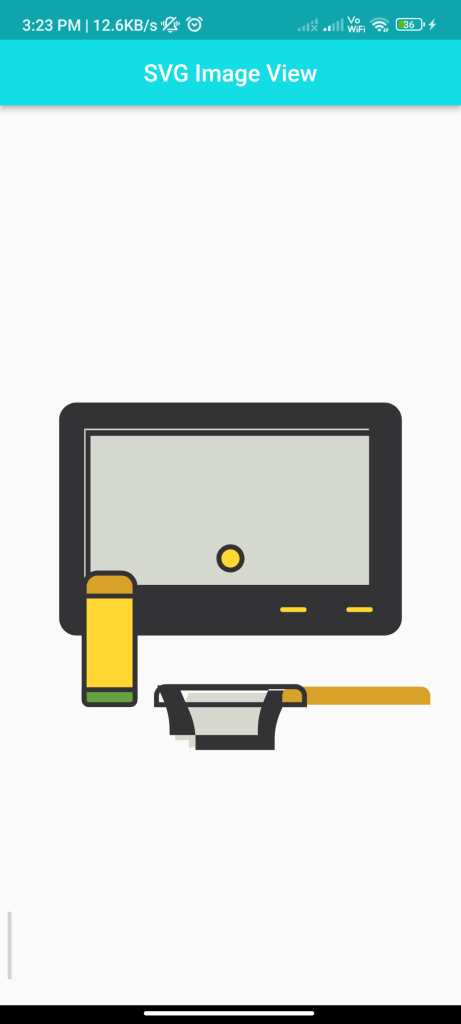
Introduction
SVG (Scalable Vector Graphics) images can be displayed in Flutter using the flutter_svg
package. This guide demonstrates how to add and display an SVG image in your Flutter app.
Content
1.Add flutter_svg
dependency:
Open your pubspec.yaml
file and add the flutter_svg
dependency.
dependencies:
flutter_svg: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the flutter_svg
package in your Dart file.
import 'package:flutter_svg/flutter_svg.dart';
3.Display the SVG image:
Use the SvgPicture.asset
widget to display the SVG image.
SvgPicture.asset(
'assets/image.svg',
semanticsLabel: 'A shark',
)
Replace 'assets/image.svg'
with the path to your SVG file. The semanticsLabel
parameter is optional and provides a description for the image for accessibility purposes.
4.Ensure the SVG file is in the assets folder:
Place your SVG file in the assets folder of your Flutter project.
5.Update pubspec.yaml
:
Include the SVG file in the pubspec.yaml
under the flutter
section.
flutter:
assets:
- assets/image.svg
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
class SvgImageWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('SVG Image Example'),
),
body: Center(
child: SvgPicture.asset(
'assets/image.svg',
semanticsLabel: 'A shark',
),
),
);
}
}
6.Run your app:
Verify that the SVG image is displayed correctly in your Flutter app.
Sample Code
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
void main() {
runApp(MyApp());
}
const String upsideSvgString = '''
<svg xmlns="http://www.w3.org/2000/svg" width="800px" height="800px" viewBox="0 0 1024 1024" class="icon" version="1.1">
<path d="M419.5 922.7v16.3h185v-16.3c0-22.4 4.6-44.6 13.6-65.2l17.3-39.7H419.6l-17.3 39.7c-8.9 20.6-13.6 42.8-13.6 65.2z" fill="#D5D9CF" />
<path d="M380.3 812.3h263.4l-20.6 47.4c-8.7 20-13.1 41.2-13.1 63v21.8H434.4v-21.8c0-21.8-4.4-43-13.1-63l-20.6-47.4zM627 801.3H348.9l13.9 32c9.3 21.4 14 44.1 14 67.4v10.8h196v-10.8c0-23.3 4.7-46 14-67.4l13.9-32z" fill="#333336" />
<path d="M169.9 684.7h684.2c18.2 0 32.9-14.8 32.9-33V211c0-18.2-14.7-33-32.9-33H169.9c-18.2 0-33 14.8-33 33v440.6c0 18.3 14.7 33.1 32.9 33.1z" fill="#68A240" />
<path d="M169.9 690.2h684.2c21.2 0 38.5-17.3 38.5-38.5V211c0-21.2-17.3-38.5-38.5-38.5H169.9c-21.2 0-38.5 17.3-38.5 38.5v440.6c0 21.3 17.3 38.6 38.5 38.6zM169.9 183.5c-15.2 0-27.5 12.3-27.5 27.5v440.6c0 15.2 12.3 27.5 27.5 27.5H854c15.2 0 27.5-12.3 27.5-27.5V211c0-15.2-12.3-27.5-27.5-27.5H169.9z" fill="#333336" />
<path d="M187 577.7h632.8V230.5H187z" fill="#D5D9CF" />
<path d="M833.9 593.2H190.1V234h643.8v359.2zM559.3 582.1H868V246.5H201.1v335.6z" fill="#333336" />
<path d="M512 521m25.7 0a25.7 25.7 0 1 1-51.4 0 25.7 25.7 0 1 1 51.4 0Z" fill="#FFD632" />
<path d="M512 550.1c17.2 0 31.2-14 31.2-31.2s-14-31.2-31.2-31.2-31.2 14-31.2 31.2 14 31.2 31.2 31.2z m0-51.3c11.1 0 20.2 9.1 20.2 20.2s-9.1 20.2-20.2 20.2-20.2-9.1-20.2-20.2 9.1-20.2 20.2-20.2z" fill="#333336" />
<path d="M676.4 643.5h-49.4c-5.5 0-9.9-4.5-9.9-9.9v-1.7c0-5.5 4.5-9.9 9.9-9.9h49.4c5.5 0 9.9 4.5 9.9 9.9v1.7c0 5.5-4.4 9.9-9.9 9.9z" fill="#FFD632" />
<path d="M676.4 649h-49.4c-8.5 0-15.4-6.9-15.4-15.4v-1.7c0-8.5 6.9-15.4 15.4-15.4h49.4c8.5 0 15.4 6.9 15.4 15.4v1.7c0 8.5-6.9 15.4-15.4 15.4zM627 627.5c-2.4 0-4.4 2-4.4 4.4v1.7c0 2.4 2 4.4 4.4 4.4h49.4c2.4 0 4.4-2 4.4-4.4v-1.7c0-2.4-2-4.4-4.4-4.4h-49.4z" fill="#333336" />
<path d="M823.4 643.5H774c-5.5 0-9.9-4.5-9.9-9.9v-1.7c0-5.5 4.5-9.9 9.9-9.9h49.4c5.5 0 9.9 4.5 9.9 9.9v1.7c0 5.5-4.5 9.9-9.9 9.9z" fill="#FFD632" />
<path d="M823.4 649H774c-8.5 0-15.4-6.9-15.4-15.4v-1.7c0-8.5 6.9-15.4 15.4-15.4h49.4c8.5 0 15.4 6.9 15.4 15.4v1.7c0 8.5-6.9 15.4-15.4 15.4zM774 627.5c-2.4 0-4.4 2-4.4 4.4v1.7c0 2.4 2 4.4 4.4 4.4h49.4c2.4 0 4.4-2 4.4-4.4v-1.7c0-2.4-2-4.4-4.4-4.4H774z" fill="#333336" />
<path d="M676.4 844h-49.4v-19.6c0-11.3 9.2-20.5 20.5-20.5h287.8c11.3 0 20.5 9.2 20.5 20.5V844z" fill="#D8A128" />
<path d="M681.9 849.5H342.1v-25.1c0-14.3 11.7-26 26-26h287.8c14.3 0 26 11.7 26 26v25.1z m-328.8-11h317.8v-14.1c0-8.3-6.7-15-15-15H368.1c-8.3 0-15 6.7-15 15v14.1z" fill="#333336" />
<path d="M300 827.2H187V589.1c0-10.6 8.6-19.2 19.2-19.2h72.7c11.7 0 21.2 9.5 21.2 21.2v236.1z" fill="#FFD632" />
<path d="M305.5 832.7h-124V589.1c0-13.6 11.1-24.7 24.7-24.7h72.7c14.7 0 26.7 12 26.7 26.7v241.6z m-113-11h102V591.1c0-8.6-7-15.7-15.7-15.7h-72.7c-7.5 0-13.7 6.1-13.7 13.7v232.6z" fill="#333336" />
<path d="M300 602.6H187v-20.9c0-16.7 13.5-30.2 30.2-30.2h52.6c16.7 0 30.2 13.5 30.2 30.2v20.9z" fill="#D8A128" />
<path d="M305.5 608.1h-124v-26.4c0-19.7 16-35.7 35.7-35.7h52.6c19.7 0 35.7 16 35.7 35.7v26.4z m-113-11h102v-15.4c0-13.6-11.1-24.7-24.7-24.7h-52.6c-13.6 0-24.7 11.1-24.7 24.7v15.4z" fill="#333336" />
<path d="M289.5 843.9h-92.1c-5.8 0-10.5-4.7-10.5-10.5v-22.8h113v22.8c0.1 5.8-4.6 10.5-10.4 10.5z" fill="#68A240" />
<path d="M289.5 849.4h-92.1c-8.8 0-16-7.2-16-16v-28.3h124v28.3c0.1 8.9-7.1 16-15.9 16z m-97-33.2v17.3c0 2.7 2.2 5 5 5h92.1c2.7 0 5-2.2 5-5v-17.3H192.5z" fill="#333336" />
</svg>
''';
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
centerTitle: true,
backgroundColor: Color.fromARGB(255, 19, 222, 230),
title: Text(
"SVG Image View",
),
),
body: Center(
child: SvgPicture.string(
upsideSvgString,
width: 500,
height: 500,
),
),
),
);
}
}
Output
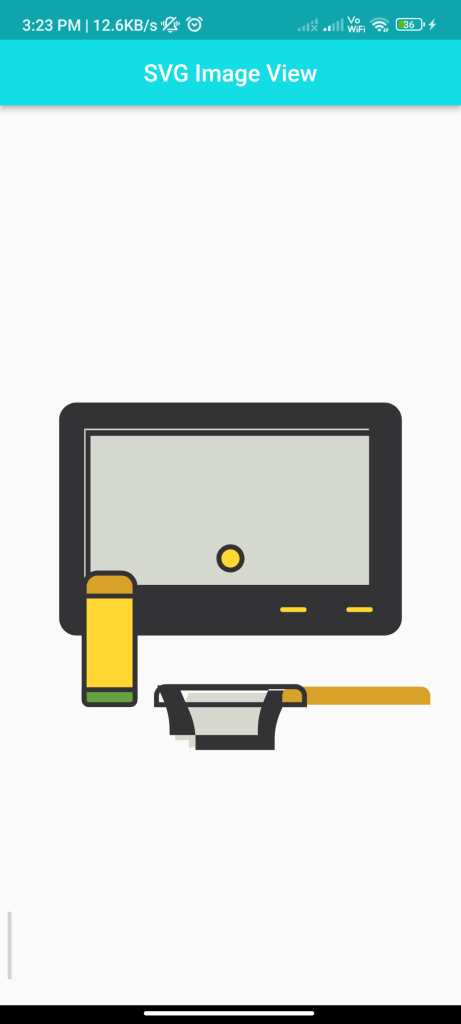
Conclusion
Using the flutter_svg
package, you can easily add and display SVG images in your Flutter app. SVG images are scalable and maintain their quality on different screen sizes, making them a great choice for high-quality graphics in Flutter applications.