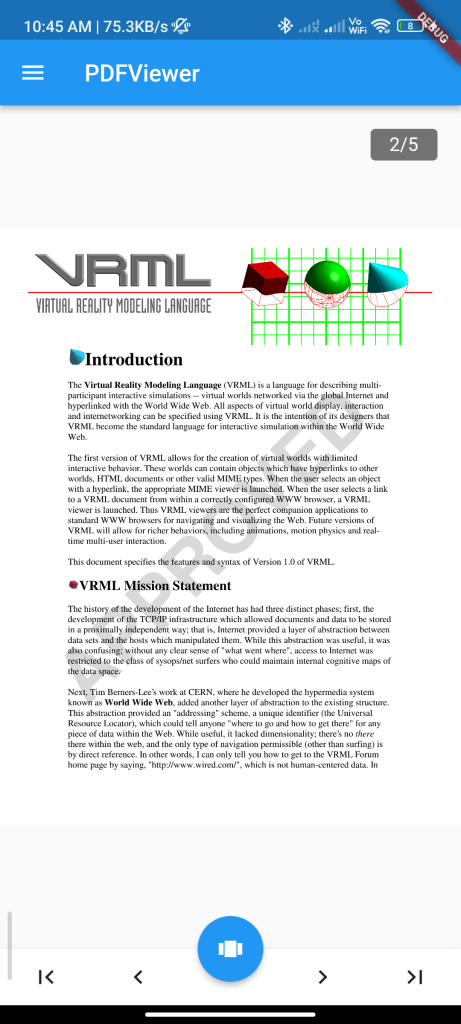
Introduction
The easy_pdf_viewer
package allows you to easily display PDF files in your Flutter app. This guide will show you how to integrate and use this package to display PDF files in your Flutter app.
Content
1.Add the easy_pdf_viewer
dependency:
Add the easy_pdf_viewer
dependency: Open your pubspec.yaml
file and add the easy_pdf_viewer
dependency.
dependencies:
easy_pdf_viewer: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the easy_pdf_viewer
package in your Dart file.
import 'package:easy_pdf_viewer/easy_pdf_viewer.dart';
3Load and display a PDF file:
Use the PDFViewer
widget to load and display a PDF file.
PDFViewer(
document: PDFDocument.openFile('path_to_your_pdf_file.pdf'),
)
Replace 'path_to_your_pdf_file.pdf'
with the actual path to your PDF file. You can also use PDFDocument.openAsset('asset_path_to_your_pdf_file.pdf')
to load a PDF file from your app’s assets.
4.Customize the PDFViewer:
You can customize the appearance of the PDFViewer widget using various parameters like scrollDirection
, swipeHorizontal
, autoSpacing
, etc.
PDFViewer(
document: PDFDocument.openFile('path_to_your_pdf_file.pdf'),
scrollDirection: Axis.vertical,
swipeHorizontal: false,
autoSpacing: true,
)
5.Run the app:
Run your Flutter app to see the PDFViewer in action. The PDF file will be displayed in the app according to the specified customization options.
5.add path in pubspec.yaml:
assets:
- assets/sample.pdf
- assets/sample2.pdf
5.Create an assets folder and add this Sample pdf:
sample pdf….
Sample Code
// import 'package:easy_pdf_viewer_example/with_progress.dart';
import 'package:flutter/material.dart';
import 'package:easy_pdf_viewer/easy_pdf_viewer.dart';
void main() => runApp(App());
class App extends StatelessWidget {
const App({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: MyApp(),
);
}
}
class MyApp extends StatefulWidget {
const MyApp({this.progressExample = false});
final bool progressExample;
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
bool _isLoading = true;
late PDFDocument document;
@override
void initState() {
super.initState();
loadDocument();
}
loadDocument() async {
document = await PDFDocument.fromAsset('assets/sample.pdf');
setState(() => _isLoading = false);
}
changePDF(value) async {
setState(() => _isLoading = true);
if (value == 1) {
document = await PDFDocument.fromAsset('assets/sample2.pdf');
} else if (value == 2) {
document = await PDFDocument.fromURL(
"https://www.africau.edu/images/default/sample.pdf",
/* cacheManager: CacheManager(
Config(
"customCacheKey",
stalePeriod: const Duration(days: 2),
maxNrOfCacheObjects: 10,
),
), */
);
} else {
document = await PDFDocument.fromAsset('assets/sample.pdf');
}
setState(() => _isLoading = false);
}
@override
Widget build(BuildContext context) {
return Scaffold(
drawer: Drawer(
child: Column(
children: <Widget>[
SizedBox(height: 36),
ListTile(
title: Text('Load from Assets'),
onTap: () {
changePDF(1);
},
),
ListTile(
title: Text('Load from URL'),
onTap: () {
changePDF(2);
},
),
ListTile(
title: Text('Restore default'),
onTap: () {
changePDF(3);
},
),
// ListTile(
// title: Text('With Progress'),
// onTap: () {
// Navigator.push(
// context,
// MaterialPageRoute(
// builder: (context) => WithProgress(),
// ),
// );
// },
// ),
],
),
),
appBar: AppBar(
title: const Text('PDFViewer'),
),
body: Center(
child: _isLoading
? Center(child: CircularProgressIndicator())
: PDFViewer(
document: document,
lazyLoad: false,
zoomSteps: 1,
numberPickerConfirmWidget: const Text(
"Confirm",
),
//uncomment below line to preload all pages
// lazyLoad: false,
// uncomment below line to scroll vertically
// scrollDirection: Axis.vertical,
//uncomment below code to replace bottom navigation with your own
/* navigationBuilder:
(context, page, totalPages, jumpToPage, animateToPage) {
return ButtonBar(
alignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
IconButton(
icon: Icon(Icons.first_page),
onPressed: () {
jumpToPage()(page: 0);
},
),
IconButton(
icon: Icon(Icons.arrow_back),
onPressed: () {
animateToPage(page: page - 2);
},
),
IconButton(
icon: Icon(Icons.arrow_forward),
onPressed: () {
animateToPage(page: page);
},
),
IconButton(
icon: Icon(Icons.last_page),
onPressed: () {
jumpToPage(page: totalPages - 1);
},
),
],
);
}, */
),
),
);
}
}
Output
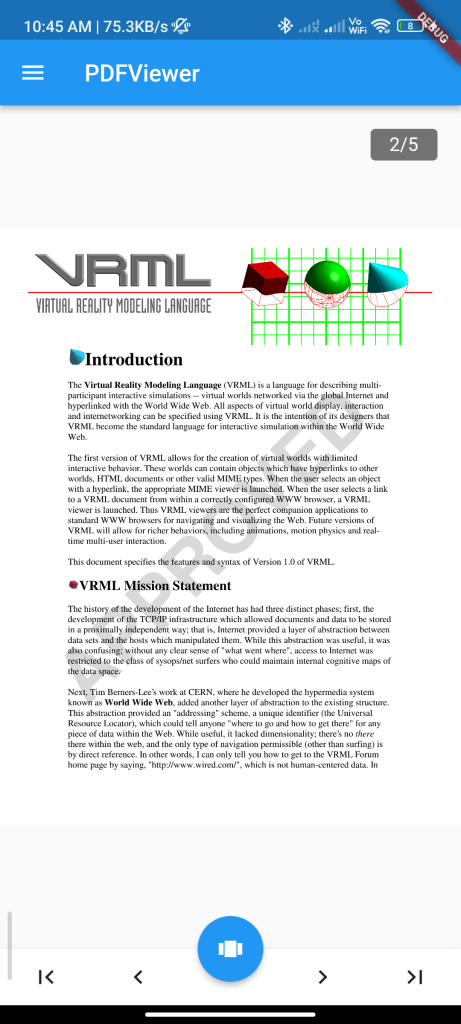
Conclusion
By following these steps, you can easily integrate the easy_pdf_viewer
package into your Flutter app and display PDF files with ease. This allows you to provide users with a convenient way to view PDF documents directly within your app.