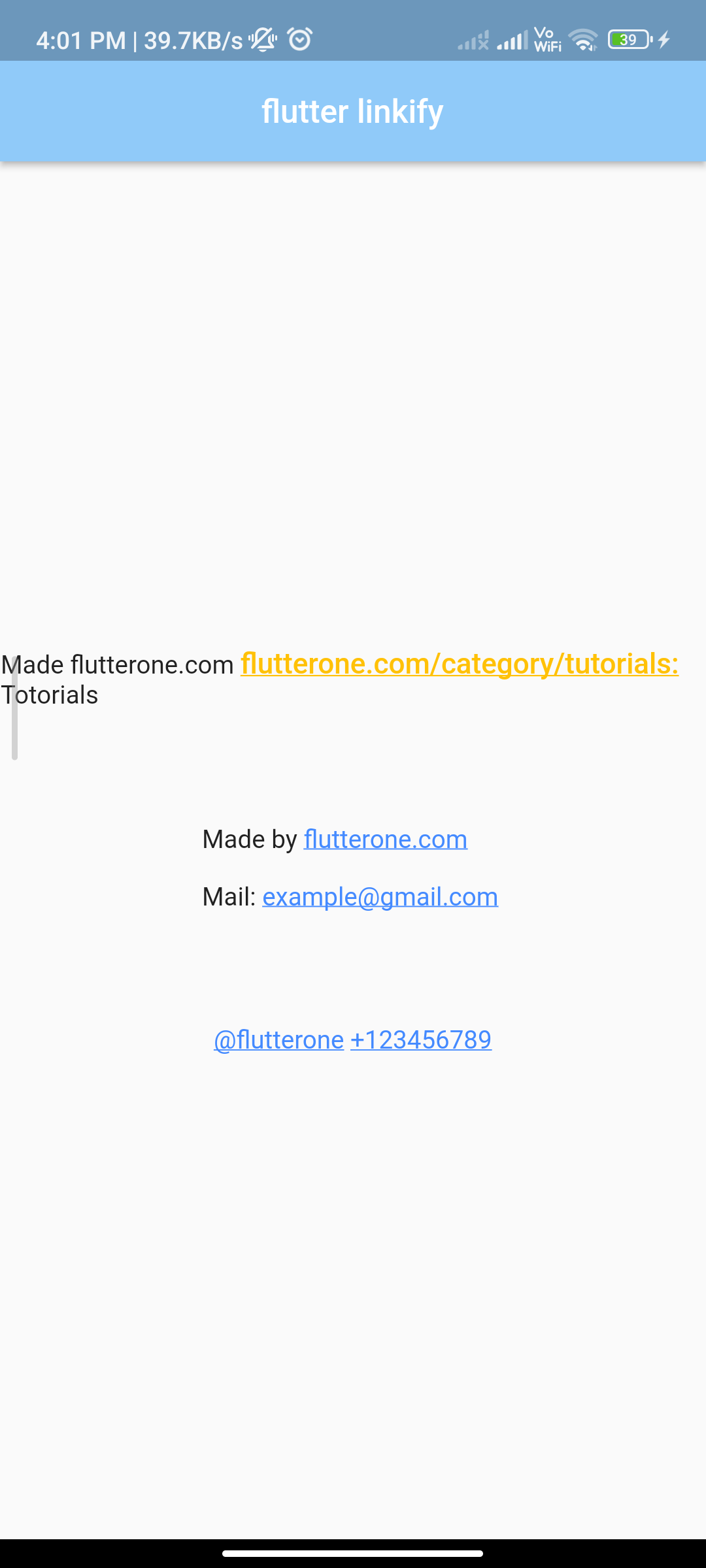
Introduction:
Hyperlinks are essential elements in text-based content, allowing users to navigate to external URLs or app screens directly from within the app. Flutter provides the flutter_linkify
package, which offers an easy way to detect and convert URLs and email addresses in text into clickable hyperlinks.
Content:
1. Add Dependency:
Begin by adding the flutter_linkify
package to your pubspec.yaml
file. This ensures that your Flutter project can access the functionalities provided by the package.
dependencies:
flutter:
sdk: flutter
flutter_linkify: ^5.0.0
Run the following command in your terminal to fetch the package:
flutter pub get
2. Import Package:
Import the flutter_linkify
package in the Dart file where you intend to implement hyperlink detection and conversion. This allows you to utilize the Linkify
widget provided by the package within your code.
import 'package:flutter/material.dart';
import 'package:flutter_linkify/flutter_linkify.dart';
3. Implement Linkify Widget:
Wrap the text that may contain URLs or email addresses with the Linkify
widget. This widget automatically detects and converts URLs and email addresses into clickable hyperlinks.
class LinkifyPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Linkify Example'),
),
body: Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: Linkify(
onOpen: (link) async {
// Handle the link opening (e.g., launch URL in browser)
},
text: 'Visit our website: https://www.example.com',
),
),
),
);
}
}
4. Customize Linkify Behavior (Optional):
Optionally, you can customize the behavior of the Linkify widget to suit your app’s design and functionality requirements. For example, you can specify text styles, link decoration, and handling of link interactions.
Linkify(
onOpen: (link) async {
// Handle the link opening (e.g., launch URL in browser)
},
text: 'Visit our website: https://www.example.com',
style: TextStyle(color: Colors.blue),
linkStyle: TextStyle(color: Colors.red, fontWeight: FontWeight.bold),
linkDecorations: LinkDecorations(
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
5. Handle Link Interactions:
Implement the onOpen
callback to define how links should be handled when tapped by the user. This callback is triggered whenever a link within the text is clicked.
6. Run the Application:
After implementing the Linkify widget, run your Flutter application on a device or emulator to observe the linkify functionality in action. This allows you to test its behavior and ensure that links are clickable and functional.
flutter run
SampleCode:
import 'package:flutter/material.dart';
import 'package:flutter_linkify/flutter_linkify.dart';
import 'package:linkify/linkify.dart';
import 'package:url_launcher/url_launcher.dart';
class LinkifyExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'flutter_linkify example',
home: Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 3,
title: Text(
'flutter linkify',
style: TextStyle(fontSize: 18, color: Colors.white),
),
backgroundColor: Colors.blue[200],
),
body: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Linkify(
linkStyle: TextStyle(color: Colors.amber, fontSize: 16, fontWeight: FontWeight.w500),
onOpen: _onOpen,
text: "Made flutterone.com https://flutterone.com/category/tutorials: Totorials",
),
SizedBox(height: 64),
SelectableLinkify(
onOpen: _onOpen,
text: "Made by https://flutterone.com\n\nMail: example@gmail.com",
),
SizedBox(height: 64),
Linkify(
onOpen: print,
text: "@flutterone +123456789",
linkifiers: [
const UserTagLinkifier(),
const PhoneNumberLinkifier(),
],
),
],
),
),
],
),
),
);
}
Future<void> _onOpen(LinkableElement link) async {
if (!await launchUrl(Uri.parse(link.url))) {
throw Exception('Could not launch ${link.url}');
}
}
}
Output:
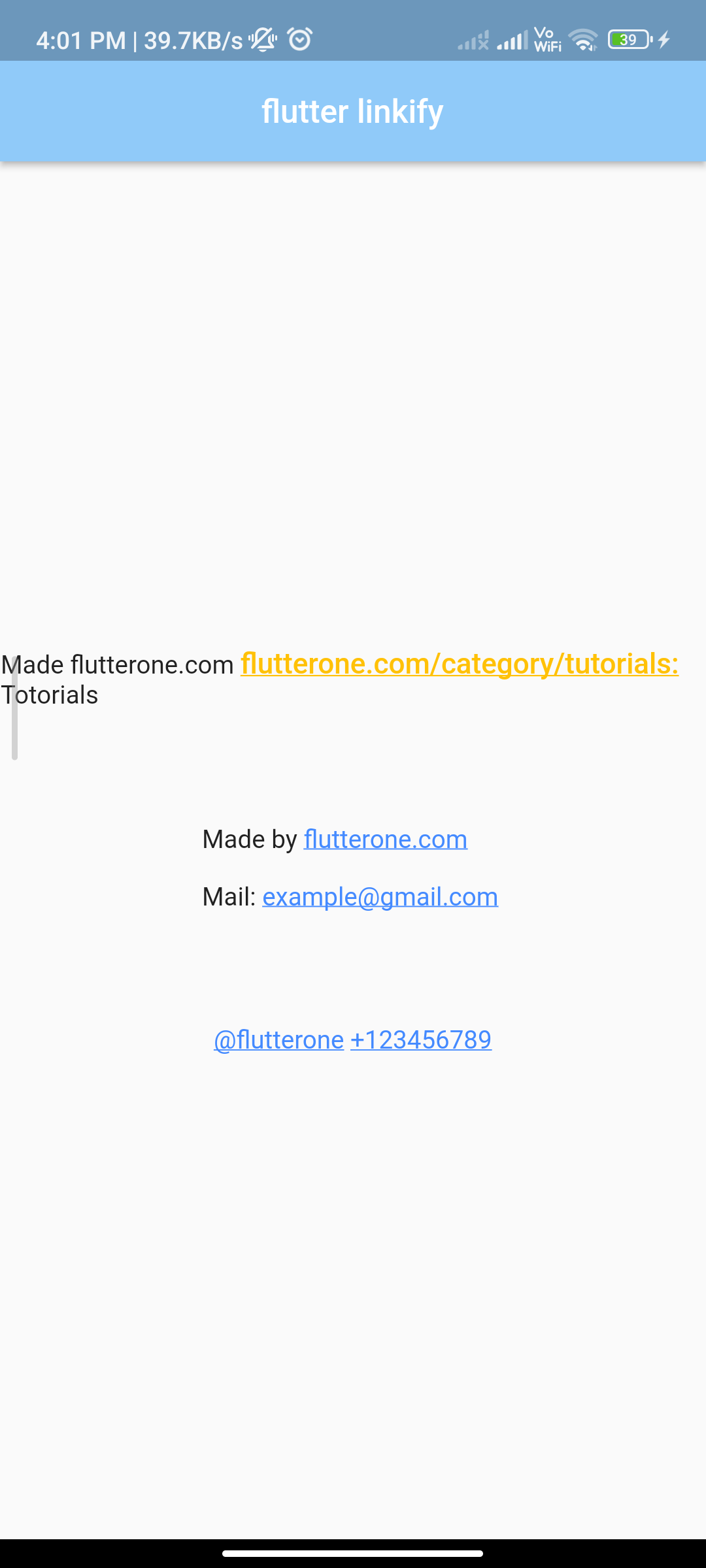
Conclusion:
Integrating Flutter Linkify into your app enables you to create clickable hyperlinks within text effortlessly. By following the steps outlined in this guide and customizing the Linkify widget as needed, you can enhance the user experience by providing easy access to relevant content or actions directly from the text. Experiment with different configurations and explore the package’s features to create a seamless linkify experience for your users.
This structured approach to implementing Flutter Linkify ensures that you can efficiently leverage its capabilities in your Flutter app, enhancing usability and interactivity.