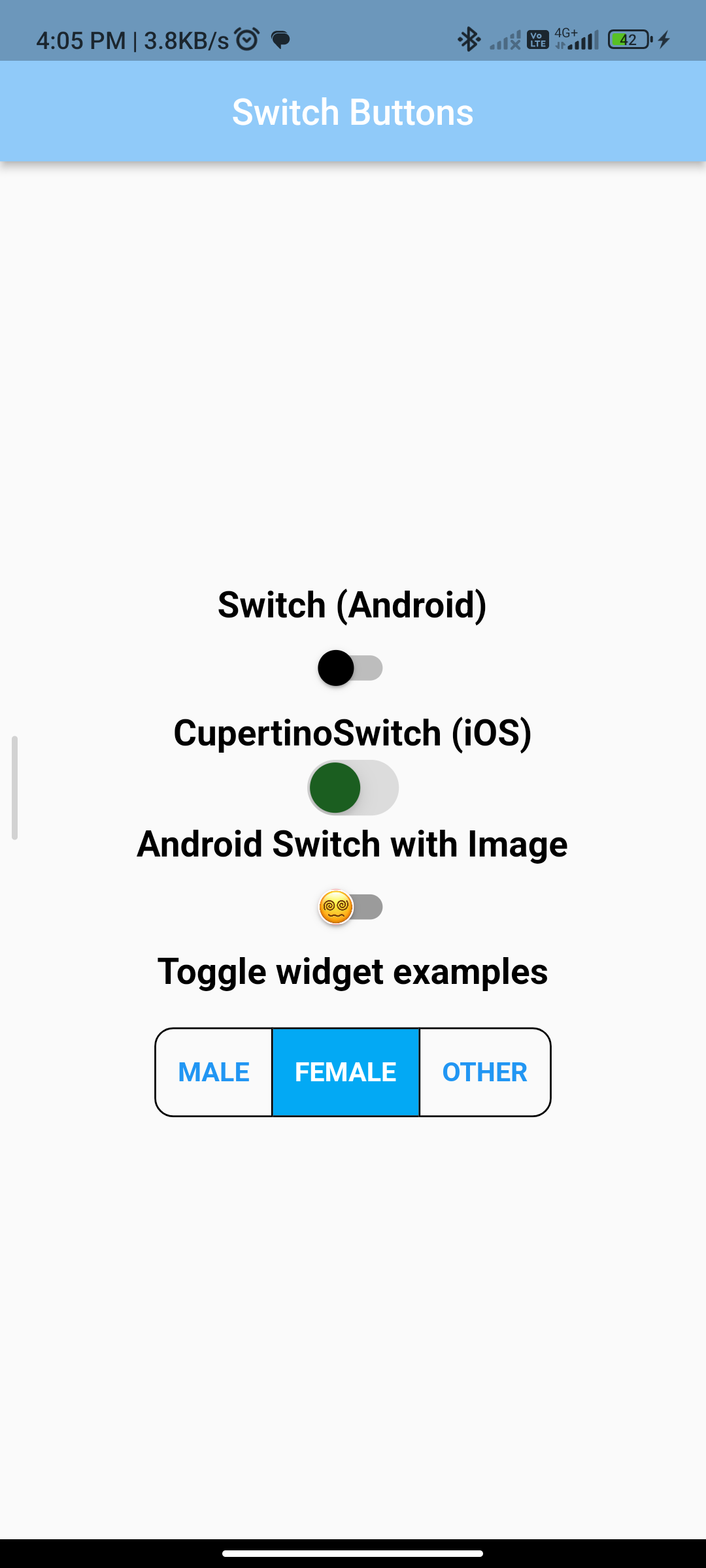
Introduction:
The Switch
widget in Flutter provides a simple and intuitive way to implement a toggle switch, allowing users to switch between two states – ON and OFF. In this step-by-step guide, we will create a Flutter app demonstrating the implementation of a Switch widget. This example will illustrate how to dynamically update the state and display the current switch status.
Content:
1. Create a New Flutter Project
Ensure that you have Flutter installed and set up on your machine. Create a new Flutter project using the following command in your terminal:
flutter create switch_example
Step 2: Add Dependencies (Optional)
In this simple example, we won’t need any additional dependencies.
Step 3: Implement the Switch Widget
Open the lib/main.dart
file and replace its content with the following code:
import 'package:flutter/material.dart';
void main() {
runApp(SwitchApp());
}
class SwitchApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Switch Example"),
),
body: Center(
child: SwitchWidget(),
),
),
);
}
}
class SwitchWidget extends StatefulWidget {
@override
_SwitchWidgetState createState() => _SwitchWidgetState();
}
class _SwitchWidgetState extends State<SwitchWidget> {
bool switchValue = false;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
switchValue ? 'Switch is ON' : 'Switch is OFF',
style: TextStyle(fontSize: 20),
),
SizedBox(height: 20),
Switch(
value: switchValue,
onChanged: (value) {
setState(() {
switchValue = value;
});
},
),
],
);
}
}
Step 4: Run the App
Save your changes and run the app using the following command in your terminal:
flutter run
Sample code:
// ignore_for_file: prefer_const_constructors
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:sizer/sizer.dart';
class Switchtoggle extends StatefulWidget {
const Switchtoggle({super.key});
@override
State<Switchtoggle> createState() => _SwitchtoggleState();
}
class _SwitchtoggleState extends State<Switchtoggle> {
bool forAndroid = false;
bool forIos = false;
bool forImage = false;
List<bool> isSelected = [true, false, false];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.blue[200],
centerTitle: true,
title: Text(
"Switch Buttons",
style: TextStyle(color: Colors.white),
),
),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Switch (Android)",
style: TextStyle(color: Colors.black, fontSize: 20, fontWeight: FontWeight.bold),
),
Switch(
activeColor: Colors.amber,
activeTrackColor: Colors.cyan,
inactiveThumbColor: Colors.black,
inactiveTrackColor: Colors.grey.shade400,
splashRadius: 50.0,
value: forAndroid,
onChanged: (value) => setState(() => forAndroid = value),
),
Text(
"CupertinoSwitch (iOS)",
style: TextStyle(color: Colors.black, fontSize: 20, fontWeight: FontWeight.bold),
),
CupertinoSwitch(
activeColor: Colors.pink.shade200,
thumbColor: Colors.green.shade900,
trackColor: Colors.black12,
value: forIos,
onChanged: (value) => setState(() => forIos = value),
),
Text(
"Android Switch with Image",
style: TextStyle(color: Colors.black, fontSize: 20, fontWeight: FontWeight.bold),
),
Switch(
trackColor: MaterialStateProperty.all(Colors.black38),
activeColor: Colors.green.withOpacity(0.4),
inactiveThumbColor: Colors.red.withOpacity(0.4),
activeThumbImage:
NetworkImage('https://upload.wikimedia.org/wikipedia/commons/thumb/9/90/Twemoji_1f600.svg/220px-Twemoji_1f600.svg.png'),
inactiveThumbImage: NetworkImage(
'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcR5yuUaYWuLYHKrhee05r_JUHlXRTbbZtACmPurbBhSxDm00y2bZNp50596dQ&s'),
value: forImage,
onChanged: (value) => setState(() => forImage = value),
),
Text(
"Toggle widget examples",
style: TextStyle(color: Colors.black, fontSize: 20, fontWeight: FontWeight.bold),
),
SizedBox(
height: 20,
),
ToggleButtons(
// list of booleans
isSelected: isSelected,
// text color of selected toggle
selectedColor: Colors.white,
// text color of not selected toggle
color: Colors.blue,
// fill color of selected toggle
fillColor: Colors.lightBlue,
// when pressed, splash color is seen
// splashColor: Colors.red,
// long press to identify highlight color
highlightColor: Colors.blue,
// if consistency is needed for all text style
textStyle: const TextStyle(fontWeight: FontWeight.bold),
// border properties for each toggle
renderBorder: true,
borderColor: Colors.black,
borderWidth: 1,
borderRadius: BorderRadius.circular(10),
selectedBorderColor: Colors.black,
// add widgets for which the users need to toggle
children: const [
Padding(
padding: EdgeInsets.symmetric(horizontal: 12),
child: Text('MALE', style: TextStyle(fontSize: 15)),
),
Padding(
padding: EdgeInsets.symmetric(horizontal: 12),
child: Text('FEMALE', style: TextStyle(fontSize: 15)),
),
Padding(
padding: EdgeInsets.symmetric(horizontal: 12),
child: Text('OTHER', style: TextStyle(fontSize: 15)),
),
],
// to select or deselect when pressed
onPressed: (int newIndex) {
setState(() {
// looping through the list of booleans values
for (int index = 0; index < isSelected.length; index++) {
// checking for the index value
if (index == newIndex) {
// one button is always set to true
isSelected[index] = true;
} else {
// other two will be set to false and not selected
isSelected[index] = false;
}
}
});
}),
],
),
),
);
}
}
Output:
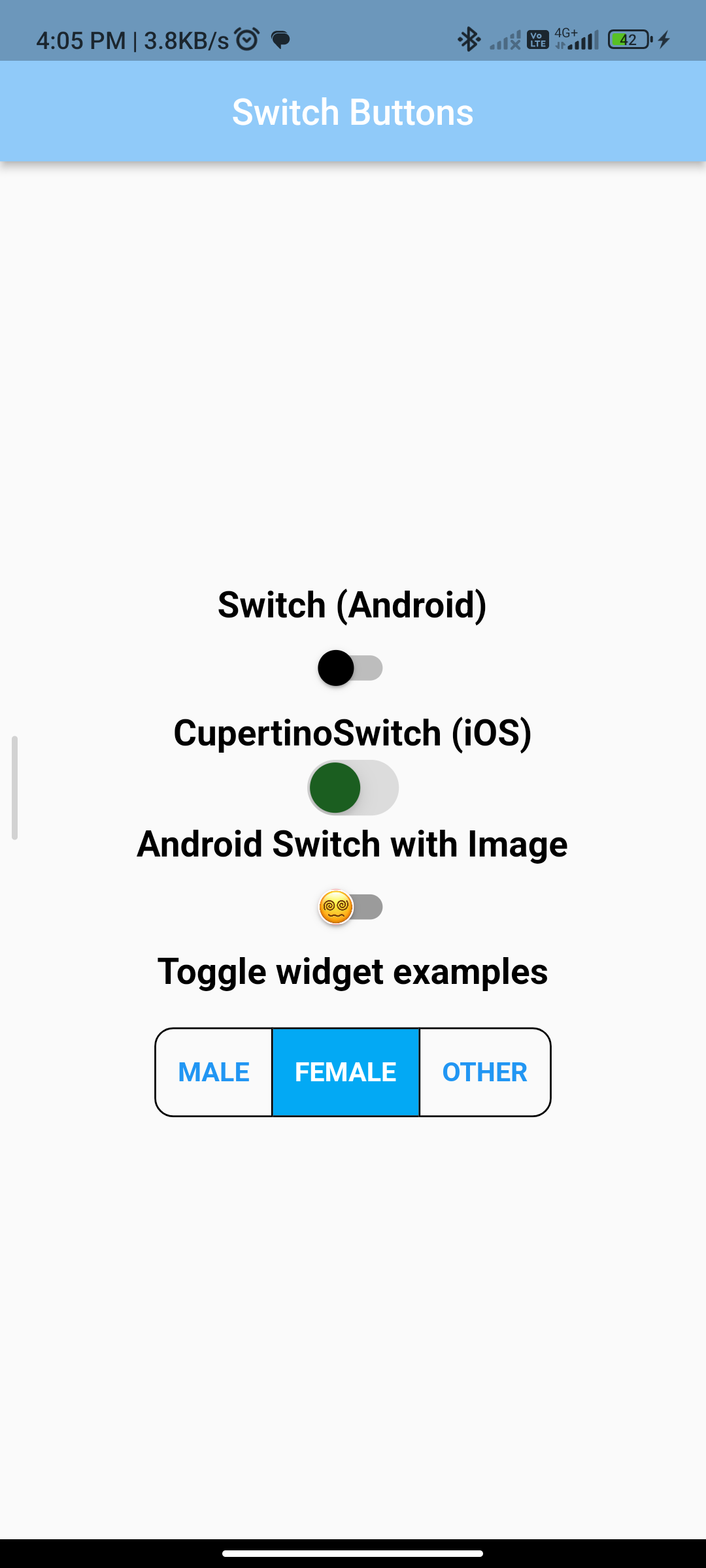
Conclusion:
In this example, we’ve demonstrated how to create a Flutter app with a Switch widget. The SwitchWidget
class, being a stateful widget, maintains the state of the switch (switchValue
). The Switch
widget itself is used to display the on/off toggle button. By tapping the switch, the state is updated, and the displayed text dynamically reflects whether the switch is ON or OFF.