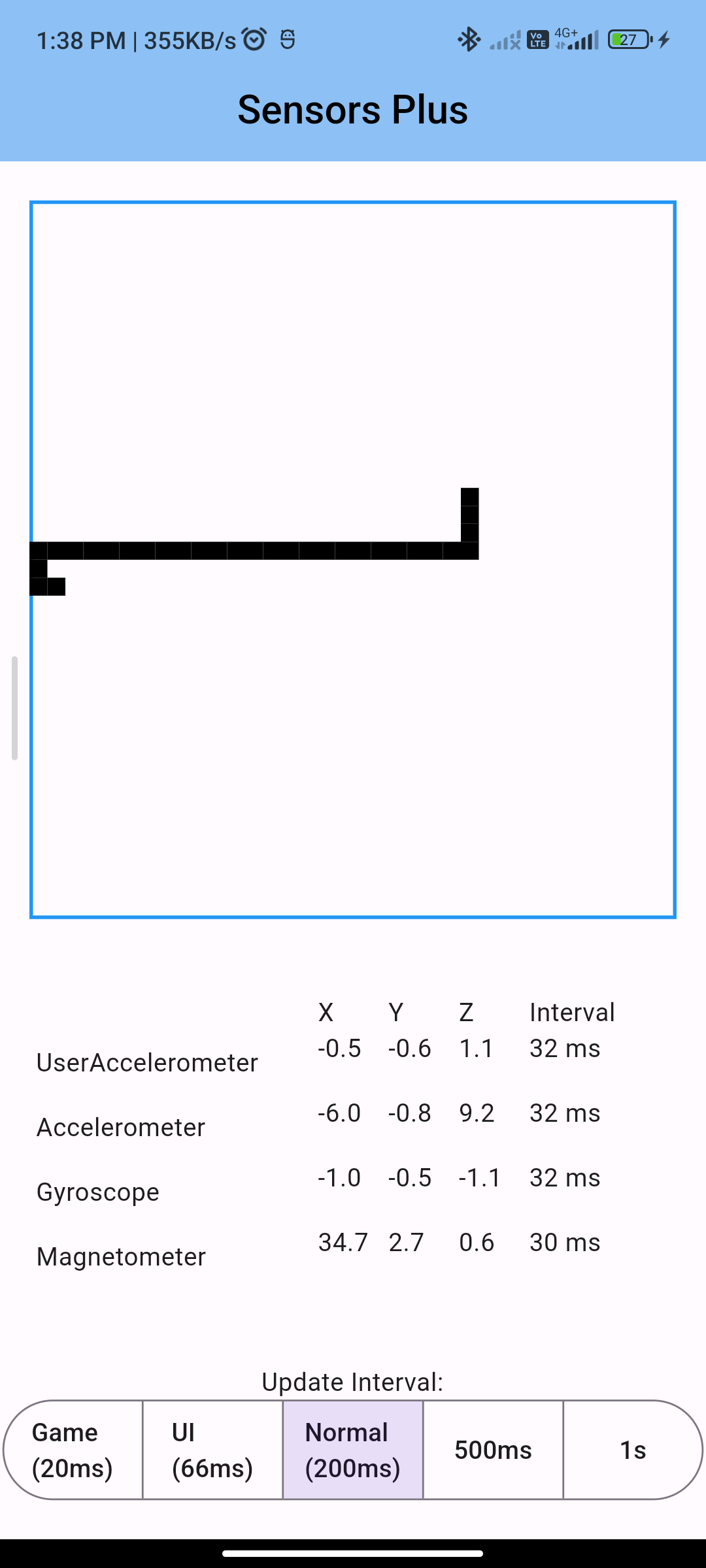
Introduction:
In Flutter, the gyroscope sensor can be used to detect the device’s orientation and rotation. The sensors_plus
package provides access to the gyroscope sensor and allows you to implement gyroscope functionality in your Flutter app. This tutorial will guide you through implementing a gyroscope sensor in Flutter using the sensors_plus
package.
Content:
Step 1. Add Dependencies:
Include the sensors_plus
package in your pubspec.yaml
file. Open the file and add the following dependency:
dependencies:
sensors_plus: ^0.6.1
Save the file and run flutter pub get
to fetch the package.
Step 2. Import Packages:
Import the required packages in your Dart file:
import 'package:flutter/material.dart';
import 'package:sensors_plus/sensors_plus.dart';
Step 3. Initialize Gyroscope Data:
Initialize gyroscope data by listening to gyroscope events. You can use a StreamSubscription
to listen to gyroscope data updates.
late StreamSubscription<GyroscopeEvent> _gyroscopeSubscription;
@override
void initState() {
super.initState();
_gyroscopeSubscription = gyroscopeEvents.listen((GyroscopeEvent event) {
// Handle gyroscope data (event.x, event.y, event.z)
});
}
@override
void dispose() {
super.dispose();
_gyroscopeSubscription.cancel();
}
Step 4. Display Gyroscope Data:
Display gyroscope data in your app’s UI. You can use a Text
widget to show the gyroscope data or update other widgets based on the gyroscope data changes.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Gyroscope Example',
home: Scaffold(
appBar: AppBar(
title: Text('Gyroscope Example'),
),
body: Center(
child: StreamBuilder<GyroscopeEvent>(
stream: gyroscopeEvents,
builder: (context, snapshot) {
if (snapshot.hasData) {
final GyroscopeEvent gyroscopeEvent = snapshot.data!;
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('X: ${gyroscopeEvent.x}'),
Text('Y: ${gyroscopeEvent.y}'),
Text('Z: ${gyroscopeEvent.z}'),
],
);
} else {
return CircularProgressIndicator();
}
},
),
),
),
);
}
Sample Code:
add this to your main file :
void main() {
WidgetsFlutterBinding.ensureInitialized();
SystemChrome.setPreferredOrientations(
[
DeviceOrientation.portraitUp,
DeviceOrientation.portraitDown,
],
);
runApp(const MyApp());
}
after that add this file homepage:
// Copyright 2017 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: public_member_api_docs
import 'dart:async';
import 'dart:math' as math;
import 'package:flutter/material.dart';
import 'package:sensors_plus/sensors_plus.dart';
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key, this.title}) : super(key: key);
final String? title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
static const Duration _ignoreDuration = Duration(milliseconds: 20);
static const int _snakeRows = 40;
static const int _snakeColumns = 36;
static const double _snakeCellSize = 10.0;
UserAccelerometerEvent? _userAccelerometerEvent;
AccelerometerEvent? _accelerometerEvent;
GyroscopeEvent? _gyroscopeEvent;
MagnetometerEvent? _magnetometerEvent;
DateTime? _userAccelerometerUpdateTime;
DateTime? _accelerometerUpdateTime;
DateTime? _gyroscopeUpdateTime;
DateTime? _magnetometerUpdateTime;
int? _userAccelerometerLastInterval;
int? _accelerometerLastInterval;
int? _gyroscopeLastInterval;
int? _magnetometerLastInterval;
final _streamSubscriptions = <StreamSubscription<dynamic>>[];
Duration sensorInterval = SensorInterval.normalInterval;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Sensors Plus",
style: TextStyle(fontSize: 22, color: Colors.black, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
body: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Center(
child: DecoratedBox(
decoration: BoxDecoration(
border: Border.all(width: 2.0, color: Colors.blue),
),
child: SizedBox(
height: _snakeRows * _snakeCellSize,
width: _snakeColumns * _snakeCellSize,
child: Snake(
rows: _snakeRows,
columns: _snakeColumns,
cellSize: _snakeCellSize,
),
),
),
),
Padding(
padding: const EdgeInsets.all(20.0),
child: Table(
columnWidths: const {
0: FlexColumnWidth(4),
4: FlexColumnWidth(2),
},
children: [
const TableRow(
children: [
SizedBox.shrink(),
Text('X'),
Text('Y'),
Text('Z'),
Text('Interval'),
],
),
TableRow(
children: [
const Padding(
padding: EdgeInsets.symmetric(vertical: 8.0),
child: Text('UserAccelerometer'),
),
Text(_userAccelerometerEvent?.x.toStringAsFixed(1) ?? '?'),
Text(_userAccelerometerEvent?.y.toStringAsFixed(1) ?? '?'),
Text(_userAccelerometerEvent?.z.toStringAsFixed(1) ?? '?'),
Text('${_userAccelerometerLastInterval?.toString() ?? '?'} ms'),
],
),
TableRow(
children: [
const Padding(
padding: EdgeInsets.symmetric(vertical: 8.0),
child: Text('Accelerometer'),
),
Text(_accelerometerEvent?.x.toStringAsFixed(1) ?? '?'),
Text(_accelerometerEvent?.y.toStringAsFixed(1) ?? '?'),
Text(_accelerometerEvent?.z.toStringAsFixed(1) ?? '?'),
Text('${_accelerometerLastInterval?.toString() ?? '?'} ms'),
],
),
TableRow(
children: [
const Padding(
padding: EdgeInsets.symmetric(vertical: 8.0),
child: Text('Gyroscope'),
),
Text(_gyroscopeEvent?.x.toStringAsFixed(1) ?? '?'),
Text(_gyroscopeEvent?.y.toStringAsFixed(1) ?? '?'),
Text(_gyroscopeEvent?.z.toStringAsFixed(1) ?? '?'),
Text('${_gyroscopeLastInterval?.toString() ?? '?'} ms'),
],
),
TableRow(
children: [
const Padding(
padding: EdgeInsets.symmetric(vertical: 8.0),
child: Text('Magnetometer'),
),
Text(_magnetometerEvent?.x.toStringAsFixed(1) ?? '?'),
Text(_magnetometerEvent?.y.toStringAsFixed(1) ?? '?'),
Text(_magnetometerEvent?.z.toStringAsFixed(1) ?? '?'),
Text('${_magnetometerLastInterval?.toString() ?? '?'} ms'),
],
),
],
),
),
Column(
mainAxisSize: MainAxisSize.min,
children: [
const Text('Update Interval:'),
SegmentedButton(
segments: [
ButtonSegment(
value: SensorInterval.gameInterval,
label: Text('Game\n'
'(${SensorInterval.gameInterval.inMilliseconds}ms)'),
),
ButtonSegment(
value: SensorInterval.uiInterval,
label: Text('UI\n'
'(${SensorInterval.uiInterval.inMilliseconds}ms)'),
),
ButtonSegment(
value: SensorInterval.normalInterval,
label: Text('Normal\n'
'(${SensorInterval.normalInterval.inMilliseconds}ms)'),
),
const ButtonSegment(
value: Duration(milliseconds: 500),
label: Text('500ms'),
),
const ButtonSegment(
value: Duration(seconds: 1),
label: Text('1s'),
),
],
selected: {sensorInterval},
showSelectedIcon: false,
onSelectionChanged: (value) {
setState(() {
sensorInterval = value.first;
userAccelerometerEventStream(samplingPeriod: sensorInterval);
accelerometerEventStream(samplingPeriod: sensorInterval);
gyroscopeEventStream(samplingPeriod: sensorInterval);
magnetometerEventStream(samplingPeriod: sensorInterval);
});
},
),
],
),
],
),
);
}
@override
void dispose() {
super.dispose();
for (final subscription in _streamSubscriptions) {
subscription.cancel();
}
}
@override
void initState() {
super.initState();
_streamSubscriptions.add(
userAccelerometerEventStream(samplingPeriod: sensorInterval).listen(
(UserAccelerometerEvent event) {
final now = DateTime.now();
setState(() {
_userAccelerometerEvent = event;
if (_userAccelerometerUpdateTime != null) {
final interval = now.difference(_userAccelerometerUpdateTime!);
if (interval > _ignoreDuration) {
_userAccelerometerLastInterval = interval.inMilliseconds;
}
}
});
_userAccelerometerUpdateTime = now;
},
onError: (e) {
showDialog(
context: context,
builder: (context) {
return const AlertDialog(
title: Text("Sensor Not Found"),
content: Text("It seems that your device doesn't support User Accelerometer Sensor"),
);
});
},
cancelOnError: true,
),
);
_streamSubscriptions.add(
accelerometerEventStream(samplingPeriod: sensorInterval).listen(
(AccelerometerEvent event) {
final now = DateTime.now();
setState(() {
_accelerometerEvent = event;
if (_accelerometerUpdateTime != null) {
final interval = now.difference(_accelerometerUpdateTime!);
if (interval > _ignoreDuration) {
_accelerometerLastInterval = interval.inMilliseconds;
}
}
});
_accelerometerUpdateTime = now;
},
onError: (e) {
showDialog(
context: context,
builder: (context) {
return const AlertDialog(
title: Text("Sensor Not Found"),
content: Text("It seems that your device doesn't support Accelerometer Sensor"),
);
});
},
cancelOnError: true,
),
);
_streamSubscriptions.add(
gyroscopeEventStream(samplingPeriod: sensorInterval).listen(
(GyroscopeEvent event) {
final now = DateTime.now();
setState(() {
_gyroscopeEvent = event;
if (_gyroscopeUpdateTime != null) {
final interval = now.difference(_gyroscopeUpdateTime!);
if (interval > _ignoreDuration) {
_gyroscopeLastInterval = interval.inMilliseconds;
}
}
});
_gyroscopeUpdateTime = now;
},
onError: (e) {
showDialog(
context: context,
builder: (context) {
return const AlertDialog(
title: Text("Sensor Not Found"),
content: Text("It seems that your device doesn't support Gyroscope Sensor"),
);
});
},
cancelOnError: true,
),
);
_streamSubscriptions.add(
magnetometerEventStream(samplingPeriod: sensorInterval).listen(
(MagnetometerEvent event) {
final now = DateTime.now();
setState(() {
_magnetometerEvent = event;
if (_magnetometerUpdateTime != null) {
final interval = now.difference(_magnetometerUpdateTime!);
if (interval > _ignoreDuration) {
_magnetometerLastInterval = interval.inMilliseconds;
}
}
});
_magnetometerUpdateTime = now;
},
onError: (e) {
showDialog(
context: context,
builder: (context) {
return const AlertDialog(
title: Text("Sensor Not Found"),
content: Text("It seems that your device doesn't support Magnetometer Sensor"),
);
});
},
cancelOnError: true,
),
);
}
}
// Copyright 2017 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: public_member_api_docs
class Snake extends StatefulWidget {
Snake({Key? key, this.rows = 20, this.columns = 20, this.cellSize = 10.0}) : super(key: key) {
assert(10 <= rows);
assert(10 <= columns);
assert(5.0 <= cellSize);
}
final int rows;
final int columns;
final double cellSize;
@override
// ignore: no_logic_in_create_state
State<StatefulWidget> createState() => SnakeState(rows, columns, cellSize);
}
class SnakeBoardPainter extends CustomPainter {
SnakeBoardPainter(this.state, this.cellSize);
GameState? state;
double cellSize;
@override
void paint(Canvas canvas, Size size) {
final blackLine = Paint()..color = Colors.red;
final blackFilled = Paint()
..color = Colors.black
..style = PaintingStyle.fill;
canvas.drawRect(
Rect.fromPoints(Offset.zero, size.bottomLeft(Offset.zero)),
blackLine,
);
for (final p in state!.body) {
final a = Offset(cellSize * p.x, cellSize * p.y);
final b = Offset(cellSize * (p.x + 1), cellSize * (p.y + 1));
canvas.drawRect(Rect.fromPoints(a, b), blackFilled);
}
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return true;
}
}
class SnakeState extends State<Snake> {
SnakeState(int rows, int columns, this.cellSize) {
state = GameState(rows, columns);
}
double cellSize;
GameState? state;
AccelerometerEvent? acceleration;
late StreamSubscription<AccelerometerEvent> _streamSubscription;
late Timer _timer;
@override
Widget build(BuildContext context) {
return CustomPaint(painter: SnakeBoardPainter(state, cellSize));
}
@override
void dispose() {
super.dispose();
_streamSubscription.cancel();
_timer.cancel();
}
@override
void initState() {
super.initState();
_streamSubscription = accelerometerEventStream().listen((AccelerometerEvent event) {
setState(() {
acceleration = event;
});
});
_timer = Timer.periodic(const Duration(milliseconds: 200), (_) {
setState(() {
_step();
});
});
}
void _step() {
final newDirection = acceleration == null
? null
: acceleration!.x.abs() < 1.0 && acceleration!.y.abs() < 1.0
? null
: (acceleration!.x.abs() < acceleration!.y.abs())
? math.Point<int>(0, acceleration!.y.sign.toInt())
: math.Point<int>(-acceleration!.x.sign.toInt(), 0);
state!.step(newDirection);
}
}
// class GameState {
// GameState(this.rows, this.columns) {
// snakeLength = math.min(rows, columns) - 5;
// }
// int rows;
// int columns;
// late int snakeLength;
// List<math.Point<int>> body = <math.Point<int>>[const math.Point<int>(0, 0)];
// math.Point<int> direction = const math.Point<int>(1, 0);
// void step(math.Point<int>? newDirection) {
// var next = body.last + direction;
// next = math.Point<int>(next.x % columns, next.y % rows);
// body.add(next);
// if (body.length > snakeLength) body.removeAt(0);
// direction = newDirection ?? direction;
// }
// }
class GameState {
GameState(this.rows, this.columns) {
snakeLength = math.min(rows, columns) - 5;
_generateFood(); // Generate initial food position
}
int rows;
int columns;
late int snakeLength;
late math.Point<int> food; // Position of the food
List<math.Point<int>> body = <math.Point<int>>[const math.Point<int>(0, 0)];
math.Point<int> direction = const math.Point<int>(1, 0);
void step(math.Point<int>? newDirection) {
var next = body.last + direction;
next = math.Point<int>(next.x % columns, next.y % rows);
// Check if the snake eats the food
if (next == food) {
snakeLength++; // Increase the snake's length
_generateFood(); // Generate a new food position
}
body.add(next);
if (body.length > snakeLength) body.removeAt(0);
direction = newDirection ?? direction;
}
void _generateFood() {
final random = math.Random();
int x = random.nextInt(columns);
int y = random.nextInt(rows);
food = math.Point<int>(x, y);
}
}
Output:
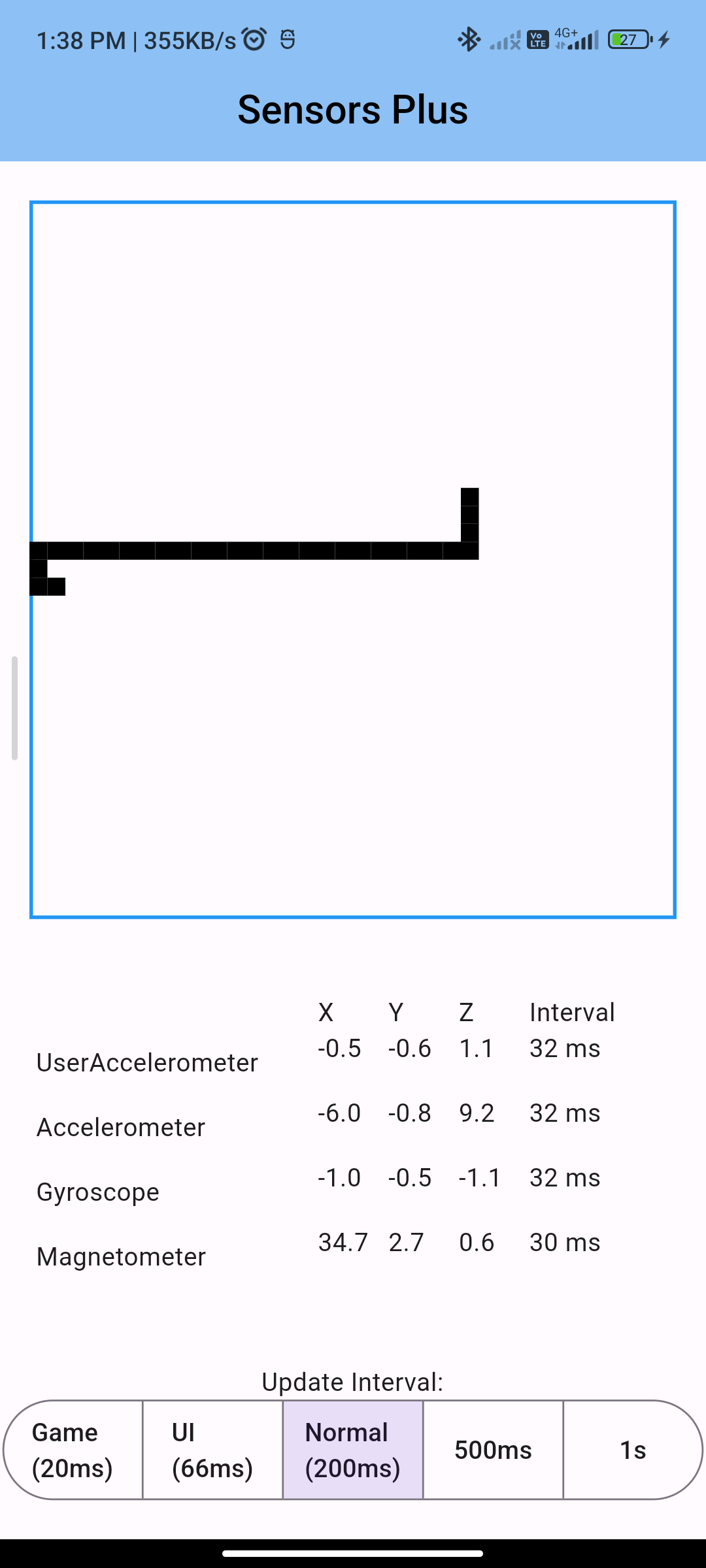
Conclusion:
Conclusion:
By following these steps, you can implement a gyroscope sensor in Flutter using the sensors_plus
package. Use the gyroscope sensor to detect the device’s orientation and rotation in your Flutter apps.
By following these steps, you can implement a gyroscope sensor in Flutter using the sensors_plus
package. Use the gyroscope sensor to detect the device’s orientation and rotation in your Flutter apps.