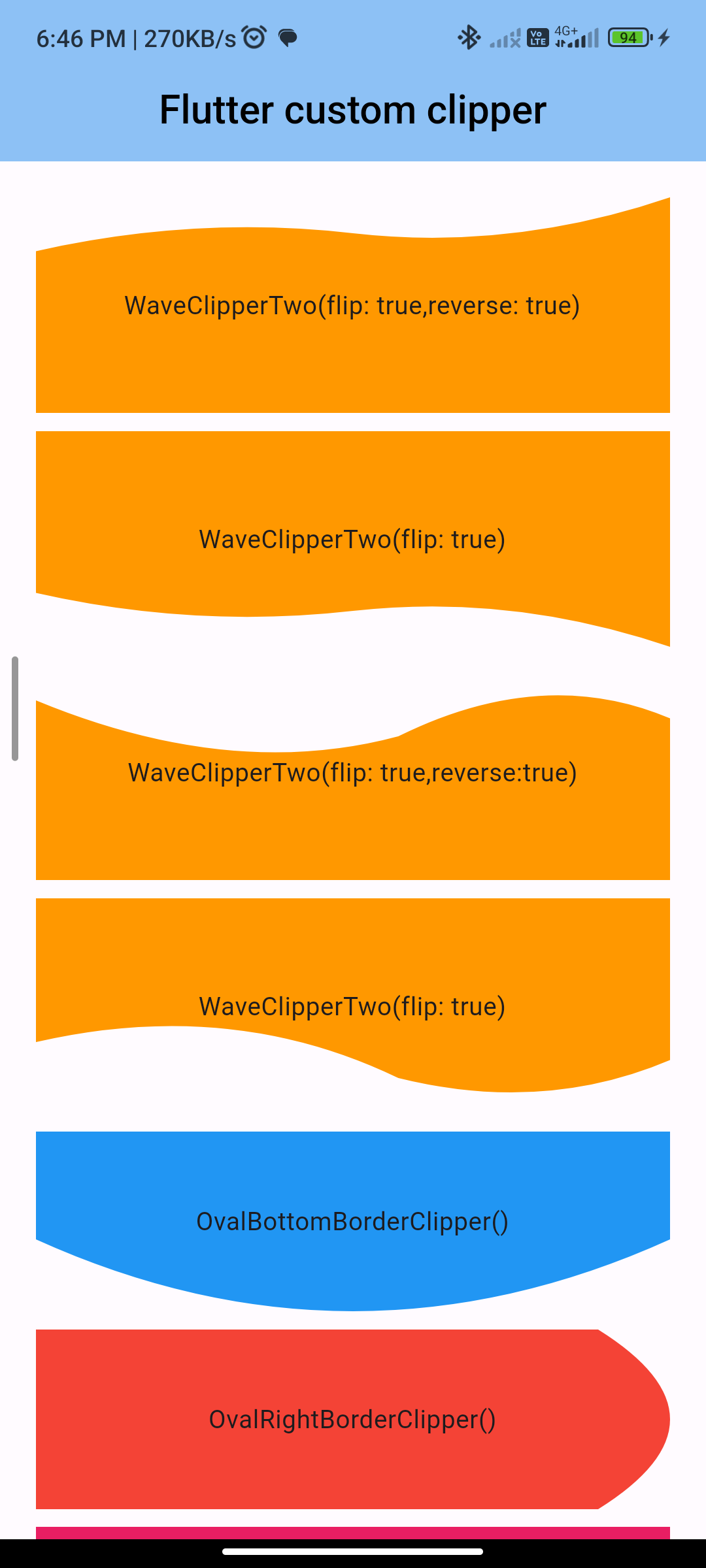
Introduction:
Implementing a custom clipper in Flutter allows you to create unique shapes and paths for clipping widgets. The flutter_custom_clippers
package provides a variety of pre-defined custom clippers that you can use in your Flutter app. This tutorial will guide you through implementing a custom clipper using the flutter_custom_clippers
package.
Content:
Step 1: Add the dependency:
Add the flutter_custom_clippers
package to your pubspec.yaml
file:
dependencies:
flutter_custom_clippers: ^2.1.0
Save the file and run flutter pub get
to install the package.
Step 2: Import the package:
Import the flutter_custom_clippers
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:flutter_custom_clippers/flutter_custom_clippers.dart';
Step 3: Use a custom clipper:
Use one of the pre-defined custom clippers provided by the flutter_custom_clippers
package. For example, to use the DiagonalPathClipperOne
clipper:
ClipPath(
clipper: DiagonalPathClipperOne(),
child: Container(
height: 200,
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.blue, Colors.green],
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
),
),
),
),
In this example, the ClipPath
widget is used to clip a Container
widget with a diagonal clipping path created by the DiagonalPathClipperOne
.
Step 4: Run the app:
Run your Flutter app to see the custom clipper in action. The Container
widget should be clipped with the specified shape.
Sample Code:
import 'package:flutter/material.dart';
import 'package:flutter_custom_clippers/flutter_custom_clippers.dart';
class CustomeCliper extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Flutter custom clipper",
style: TextStyle(fontSize: 22, color: Colors.black, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
body: ListView(
padding: EdgeInsets.all(20.0),
children: <Widget>[
ClipPath(
clipper: WaveClipperOne(flip: true, reverse: true),
child: Container(
height: 120,
color: Colors.orange,
child: Center(child: Text("WaveClipperTwo(flip: true,reverse: true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperOne(flip: true),
child: Container(
height: 120,
color: Colors.orange,
child: Center(child: Text("WaveClipperTwo(flip: true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperTwo(flip: true, reverse: true),
child: Container(
height: 120,
color: Colors.orange,
child: Center(child: Text("WaveClipperTwo(flip: true,reverse:true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperTwo(flip: true),
child: Container(
height: 120,
color: Colors.orange,
child: Center(child: Text("WaveClipperTwo(flip: true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: OvalBottomBorderClipper(),
child: Container(
height: 100,
color: Colors.blue,
child: Center(child: Text("OvalBottomBorderClipper()")),
),
),
const SizedBox(height: 10.0),
ClipPath(
clipper: OvalRightBorderClipper(),
child: Container(
height: 100,
color: Colors.red,
child: Center(child: Text("OvalRightBorderClipper()")),
),
),
const SizedBox(height: 10.0),
ClipPath(
clipper: SideCutClipper(),
child: Container(
height: 200,
color: Colors.pink,
//play with scals to get more clear versions
child: Center(child: Text("SideCutClipper()")),
),
),
const SizedBox(height: 10.0),
ClipPath(
clipper: OvalLeftBorderClipper(),
child: Container(
height: 100,
color: Colors.green,
child: Center(child: Text("OvalLeftBorderClipper()")),
),
),
const SizedBox(height: 10.0),
ClipPath(
clipper: ArcClipper(),
child: Container(
height: 100,
color: Colors.pink,
child: Center(child: Text("ArcClipper()")),
),
),
ClipPath(
clipper: PointsClipper(),
child: Container(
height: 100,
color: Colors.indigo,
child: Center(child: Text("PointsClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: TriangleClipper(),
child: Container(
height: 100,
color: Colors.deepOrange,
child: Center(child: Text("TriangleClipper()")),
),
),
ClipPath(
clipper: MovieTicketClipper(),
child: Container(
height: 100,
color: Colors.deepPurple,
child: Center(child: Text("MovieTicketClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: MovieTicketBothSidesClipper(),
child: Container(
height: 100,
color: Colors.green,
child: Center(child: Text("MovieTicketBothSidesClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: MultipleRoundedCurveClipper(),
child: Container(
height: 100,
color: Colors.pink,
child: Center(child: Text("MultipleRoundedCurveClipper()")),
),
),
SizedBox(height: 20.0),
ClipPath(
clipper: MultiplePointedEdgeClipper(),
child: Container(
height: 100,
color: Colors.green,
child: Center(child: Text("MultiplePointedEdgeClipper()")),
),
),
SizedBox(height: 20.0),
ClipPath(
clipper: OctagonalClipper(),
child: Container(
height: 220,
color: Colors.red,
child: Center(child: Text("OctagonalClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: HexagonalClipper(),
child: Container(
height: 220,
color: Colors.blueAccent,
child: Center(child: Text("HexagonalClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: HexagonalClipper(reverse: true),
child: Container(
height: 220,
color: Colors.orangeAccent,
child: Center(child: Text("HexagonalClipper(reverse: true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: ParallelogramClipper(),
child: Container(
height: 220,
color: Colors.pinkAccent,
child: Center(child: Text("ParallelogramClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: DiagonalPathClipperOne(),
child: Container(
height: 120,
color: Colors.red,
child: Center(child: Text("DiagonalPathClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: DiagonalPathClipperTwo(),
child: Container(
height: 120,
color: Colors.pink,
child: Center(child: Text("DiagonalPathClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperOne(),
child: Container(
height: 120,
color: Colors.deepPurple,
child: Center(child: Text("WaveClipperOne()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperOne(reverse: true),
child: Container(
height: 120,
color: Colors.deepPurple,
child: Center(child: Text("WaveClipperOne(reverse: true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperTwo(),
child: Container(
height: 120,
color: Colors.orange,
child: Center(child: Text("WaveClipperTwo()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: WaveClipperTwo(reverse: true),
child: Container(
height: 120,
color: Colors.orange,
child: Center(child: Text("WaveClipperTwo(reverse: true)")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: RoundedDiagonalPathClipper(),
child: Container(
height: 320,
decoration: BoxDecoration(
borderRadius: BorderRadius.all(Radius.circular(50.0)),
color: Colors.orange,
),
child: Center(child: Text("RoundedDiagonalPathClipper()")),
),
),
SizedBox(height: 10.0),
ClipPath(
clipper: OvalTopBorderClipper(),
child: Container(
height: 120,
color: Colors.yellow,
child: Center(child: Text("OvalTopBorderClipper()")),
),
),
SizedBox(height: 10),
ClipPath(
clipper: ArrowClipper(70, 80, Edge.LEFT),
child: Container(
height: 120,
color: Colors.pink,
child: Center(child: Text("ArrowClipper()")),
),
),
SizedBox(height: 10),
ClipPath(
clipper: ArrowClipper(70, 80, Edge.RIGHT),
child: Container(
height: 120,
color: Colors.red,
child: Center(child: Text("ArrowClipper()")),
),
),
SizedBox(height: 10),
ClipPath(
clipper: ArrowClipper(20, 300, Edge.TOP),
child: Container(
height: 70,
width: 50,
color: Colors.blue,
child: Center(child: Text("ArrowClipper()")),
),
),
SizedBox(height: 10),
ClipPath(
clipper: ArrowClipper(70, 80, Edge.BOTTOM),
child: Container(
height: 120,
color: Colors.green,
child: Center(child: Text("ArrowClipper()")),
),
),
SizedBox(height: 10),
ClipPath(
clipper: StarClipper(8),
child: Container(
height: 450,
color: Colors.indigo,
child: Center(child: Text("StarClipper()")),
),
),
ClipPath(
clipper: MessageClipper(borderRadius: 16),
child: Container(
height: 200,
decoration: BoxDecoration(
borderRadius: BorderRadius.all(Radius.circular(16.0)),
color: Colors.red,
),
child: Center(child: Text("MessageClipper()")),
),
),
SizedBox(height: 20),
ClipPath(
clipper: WavyCircleClipper(32),
child: Container(
width: 400,
height: 400,
color: Colors.purple,
child: const Center(child: Text("WavyCircleClipper()")),
),
),
],
),
);
}
}
Output:
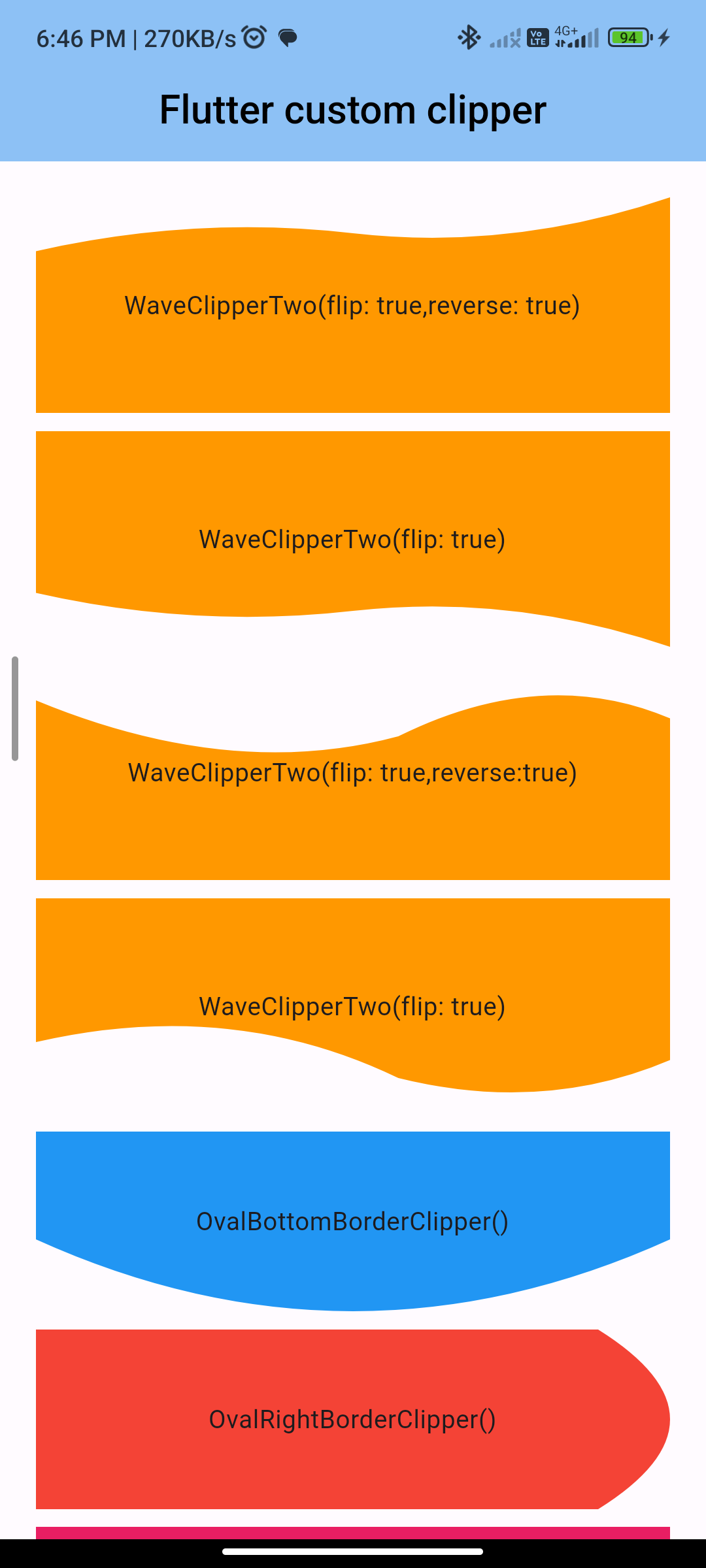
Conclusion:
By following these steps, you can easily implement custom clippers in Flutter using the flutter_custom_clippers
package. This allows you to create unique and visually appealing shapes and paths for clipping widgets in your Flutter app.