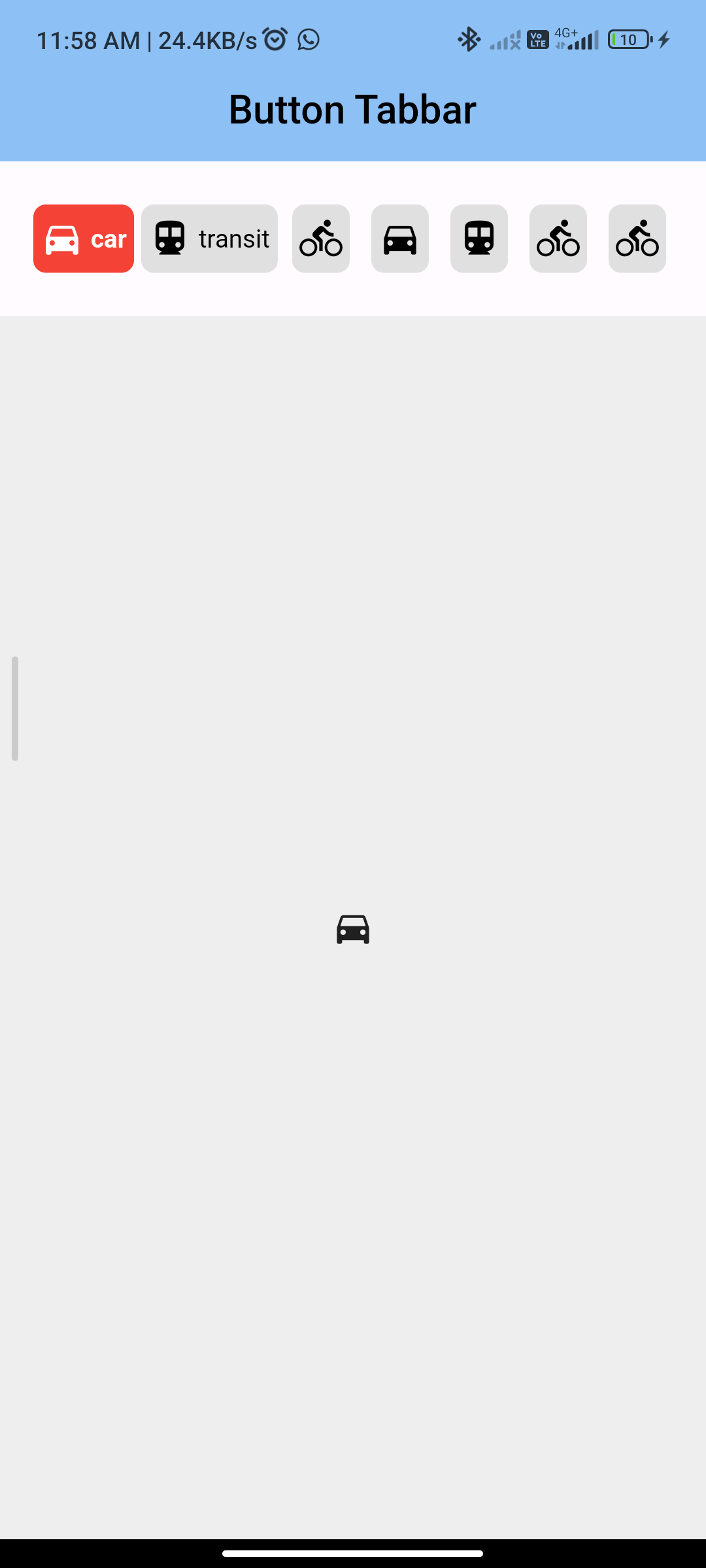
Introduction:
Implementing a buttons_tabbar
in Flutter allows you to create a tab bar with customizable buttons for navigation. The buttons_tabbar
package provides a simple way to implement this feature. This tutorial will guide you through implementing a buttons_tabbar
in Flutter using the buttons_tabbar
package.
Content:
Step 1: Add the dependency:
Add the buttons_tabbar
package to your pubspec.yaml
file:
dependencies:
buttons_tabbar: ^1.0.0
Save the file and run flutter pub get
to install the package.
Step 2: Import the package:
Import the buttons_tabbar
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:buttons_tabbar/buttons_tabbar.dart';
Step 3: Create a ButtonsTabBar widget:
Create a ButtonsTabBar
widget and define the tabs using Tab
widgets. Each Tab
widget represents a tab in the tab bar:
Scaffold(
appBar: AppBar(
title: Text('Buttons TabBar Example'),
),
body: DefaultTabController(
length: 3,
child: Column(
children: <Widget>[
ButtonsTabBar(
backgroundColor: Colors.blue,
unselectedBackgroundColor: Colors.grey[300],
unselectedLabelStyle: TextStyle(color: Colors.black),
labelStyle: TextStyle(color: Colors.white),
tabs: [
Tab(text: 'Tab 1'),
Tab(text: 'Tab 2'),
Tab(text: 'Tab 3'),
],
),
Expanded(
child: TabBarView(
children: [
// Tab 1 content
Center(child: Text('Tab 1 content')),
// Tab 2 content
Center(child: Text('Tab 2 content')),
// Tab 3 content
Center(child: Text('Tab 3 content')),
],
),
),
],
),
),
);
n this example, the ButtonsTabBar
widget is created with three tabs. The backgroundColor
, unselectedBackgroundColor
, unselectedLabelStyle
, and labelStyle
properties are used to customize the appearance of the tab bar.
Step 4: Run the app:
Run your Flutter app to see the buttons_tabbar
in action. You should see a tab bar with three tabs at the top of the screen.
Sample Code:
// ignore_for_file: prefer_const_literals_to_create_immutables, prefer_const_constructors
import 'package:buttons_tabbar/buttons_tabbar.dart';
import 'package:flutter/material.dart';
class ButtonTabbar extends StatefulWidget {
ButtonTabbar({Key? key}) : super(key: key);
@override
_ButtonTabbarState createState() => _ButtonTabbarState();
}
class _ButtonTabbarState extends State<ButtonTabbar> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
elevation: 2,
title: Text(
"Button Tabbar",
style: TextStyle(fontSize: 22, color: Colors.black, fontWeight: FontWeight.w500),
),
backgroundColor: Colors.blue[200],
),
body: SafeArea(
child: DefaultTabController(
length: 7,
child: Container(
// color: Colors.green,
child: Column(
children: <Widget>[
SizedBox(
height: 20,
),
ButtonsTabBar(
borderColor: Colors.black,
backgroundColor: Colors.red,
unselectedBackgroundColor: Colors.grey[300],
unselectedLabelStyle: TextStyle(color: Colors.black),
labelStyle: TextStyle(color: Colors.white, fontWeight: FontWeight.bold),
tabs: [
Tab(
icon: Icon(Icons.directions_car),
text: "car",
),
Tab(
icon: Icon(Icons.directions_transit),
text: "transit",
),
Tab(icon: Icon(Icons.directions_bike)),
Tab(icon: Icon(Icons.directions_car)),
Tab(icon: Icon(Icons.directions_transit)),
Tab(icon: Icon(Icons.directions_bike)),
Tab(icon: Icon(Icons.directions_bike)),
],
),
SizedBox(
height: 20,
),
Expanded(
child: TabBarView(
children: <Widget>[
Container(
color: Colors.grey[200],
child: Center(
child: Icon(Icons.directions_car),
),
),
Center(
child: Icon(Icons.directions_transit),
),
Center(
child: Icon(Icons.directions_bike),
),
Center(
child: Icon(Icons.directions_car),
),
Center(
child: Icon(Icons.directions_transit),
),
Center(
child: Icon(Icons.directions_bike),
),
Center(
child: Icon(Icons.directions_bike),
),
],
),
),
],
),
),
),
),
);
}
}
Output:
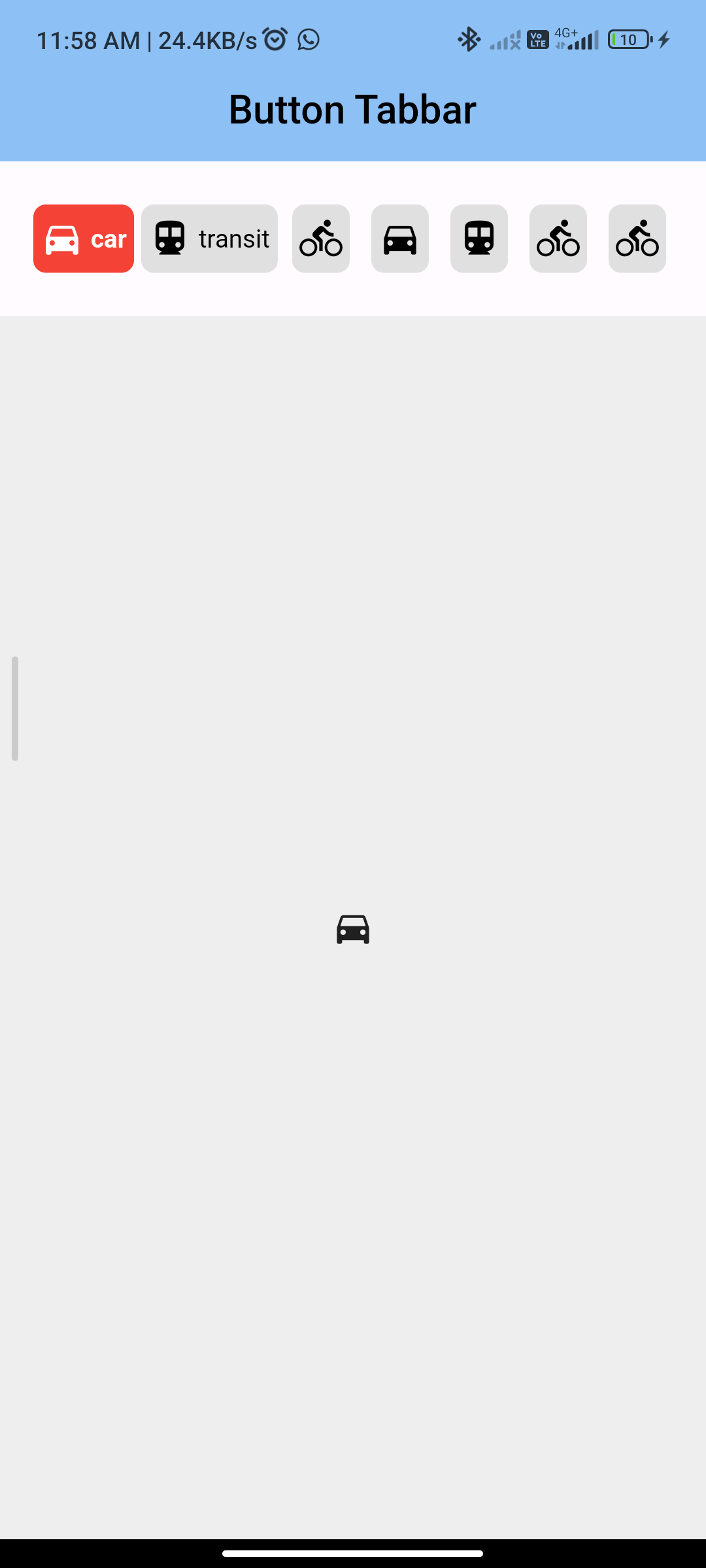
Conclusion:
By following these steps, you can easily implement a buttons_tabbar
in Flutter using the buttons_tabbar
package. This allows you to create a tab bar with customizable buttons for navigation in your app.