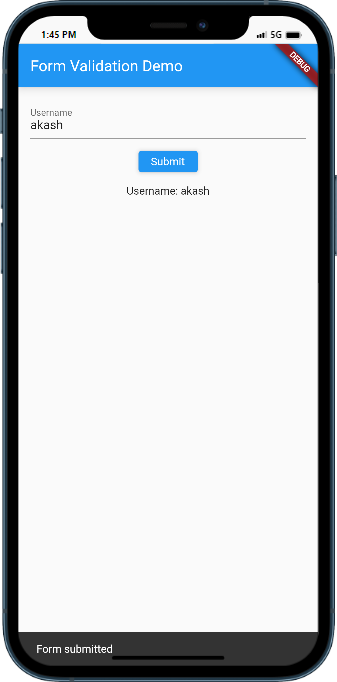
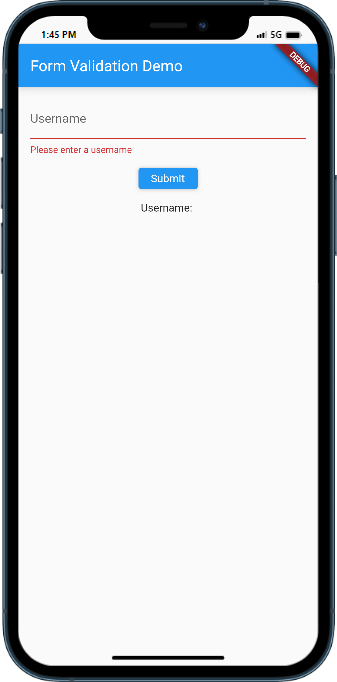
Introduction
Form validation is an essential part of building robust and user-friendly Flutter applications. It ensures that the user provides valid and complete input before submitting the form. One common validation requirement is to display an error message when a form field is empty.
In this tutorial, we will explore how to validate form fields and display error messages when they are empty. We will use Flutter’s built-in form validation features to achieve this functionality. By the end of this tutorial, you will be able to enhance your app’s user experience by providing meaningful feedback to users when they forget to fill in required fields.
Let’s get started with the step-by-step implementation!
Content:
Step 1: Create a Form Widget
To implement form field validation, we need to encapsulate our form fields within a Form
widget. The Form
widget provides built-in validation capabilities and manages the form’s state.
- Open your Flutter project in your preferred IDE or text editor.
- Locate the file where you want to implement the form field validation (e.g.,
my_form_screen.dart
). - Import the necessary Flutter packages:
import 'package:flutter/material.dart';
- Create a new stateful widget that represents your form screen:
class MyFormScreen extends StatefulWidget { @override _MyFormScreenState createState() => _MyFormScreenState(); } class _MyFormScreenState extends State<MyFormScreen> { // Form key for form validation final _formKey = GlobalKey<FormState>(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('My Form'), ), body: Form( key: _formKey, child: SingleChildScrollView( child: Column( children: [ // Add form fields here ], ), ), ), ); } }
Step 2: Add Form Fields
Now that we have our form widget set up, let’s add form fields to it. For demonstration purposes, we will add a single text form field.
- Inside the
Column
widget, add aTextFormField
widget:Column( children: [ TextFormField( validator: (value) { if (value.isEmpty) { return 'Please enter a value'; } return null; }, decoration: InputDecoration( labelText: 'Name', ), ), // Add more form fields here if needed ], ),
In the above code snippet, we define a
TextFormField
widget with a validator function. The validator function checks if the form field value is empty and returns an error message if it is.
Step 3: Display the Error Message
To display the error message when a form field is empty, we can utilize the Text
widget and conditionally show/hide it based on the form field’s validation state.
- Modify the form field code as follows:
TextFormField( validator: (value) { if (value.isEmpty) { return 'Please enter a value'; } return null; }, decoration: InputDecoration( labelText: 'Name', errorText: _formKey.currentState?.validate() == false ? 'Please enter a value' : null, ), ),
In the
InputDecoration
of theTextFormField
, we set theerrorText
property to display the error message. We use the_formKey.currentState?.validate()
expression to check if the form is currently invalid (i.e., if any form field validation failed).
That’s it! Now, when the form is submitted and a form field is empty, the corresponding error message will be displayed.
4. Run the app:
Save the main.dart
file and run the app using the following command in your terminal:
flutter run
Sample Code:
import 'package:flutter/material.dart';
class myappcreation extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Form Validation Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text('Form Validation Demo'),
),
body: MyForm(),
),
);
}
}
class MyForm extends StatefulWidget {
@override
_MyFormState createState() => _MyFormState();
}
class _MyFormState extends State<MyForm> {
final _formKey = GlobalKey<FormState>();
String _username = '';
@override
Widget build(BuildContext context) {
return Form(
key: _formKey,
child: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Username',
),
validator: (value) {
if (value!.isEmpty) {
return 'Please enter a username';
}
return null;
},
onChanged: (value) {
setState(() {
_username = value;
});
},
),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: () {
if (_formKey.currentState!.validate()) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('Form submitted')),
);
}
},
child: Text('Submit'),
),
SizedBox(height: 16.0),
Text('Username: $_username'),
],
),
),
);
}
}
Output:
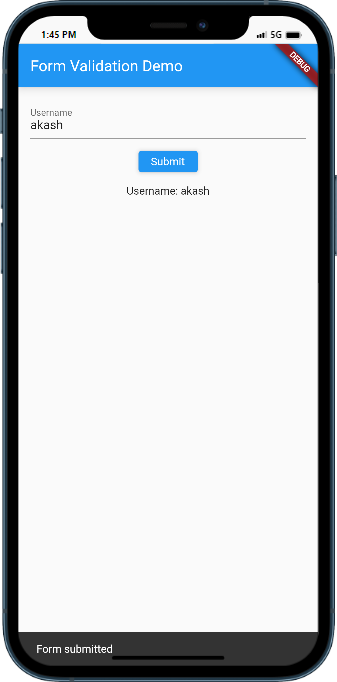
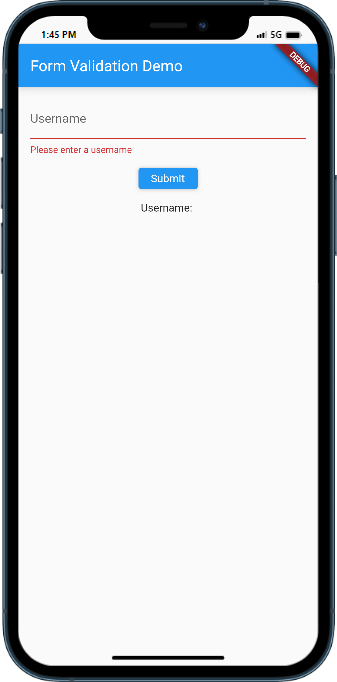
Conclusion:
In this tutorial, you learned how to validate form fields and display error messages when they are empty in Flutter. By implementing form field validation, you can improve your app’s user experience by providing meaningful feedback to users. Remember to encapsulate your form fields within a Form
widget, define a validator function for each form field, and utilize the errorText
property to display error messages.
By using these techniques, you can ensure that users provide valid input and create more robust and user-friendly Flutter applications.