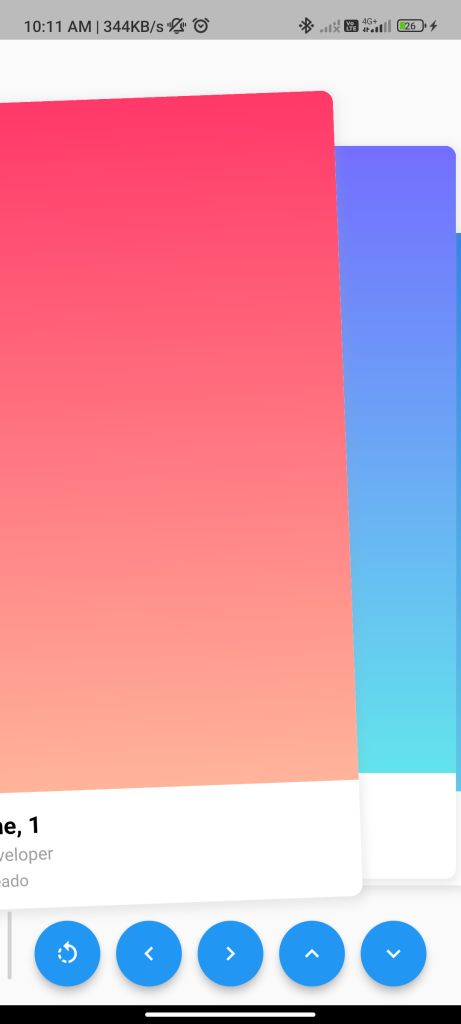
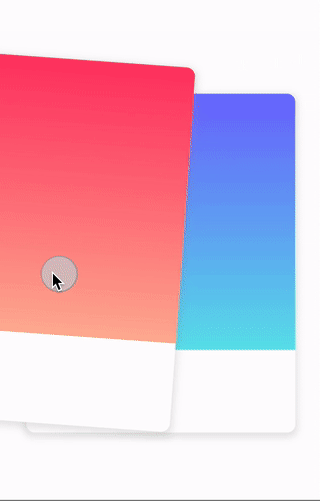
Introduction
The card_swiper
package enables you to create a card swiper interface in your Flutter app, akin to popular dating apps. This guide will demonstrate how to utilize this package to implement a card swiper in your app.
Content
1.Add the card_swiper
dependency:
Open your pubspec.yaml
file and add the card_swiper
dependency.
dependencies:
card_swiper: ^latest_version
Run flutter pub get
to install the package.
2.Import the package:
Import the card_swiper
package in your Dart file.
import 'package:card_swiper/card_swiper.dart';
3.Create a list of cards:
Define a list of widgets representing the cards to display in the swiper.
List<Widget> cards = [
Card(child: Text('Card 1')),
Card(child: Text('Card 2')),
Card(child: Text('Card 3')),
];
Replace Card(child: Text('...'))
with your custom card widgets.
4.Use the CardSwiper
widget:
Utilize the CardSwiper
widget to display the swiper with the cards.
CardSwiper(
cards: cards,
height: 400, // Set the height of the swiper
initialIndex: 0, // Set the initial index of the swiper
enableLoop: false, // Set to true to enable looping
onSwipeLeft: (index) => print('Swiped left on card $index'),
onSwipeRight: (index) => print('Swiped right on card $index'),
)
Customize the height
, initialIndex
, and other properties as needed for your app. You can also listen to swipe events using the onSwipeLeft
and onSwipeRight
callbacks.
Sample Code
import 'package:flutter/material.dart';
import 'package:flutter_card_swiper/flutter_card_swiper.dart';
void main() {
runApp(
const MaterialApp(
debugShowCheckedModeBanner: false,
home: Example(),
),
);
}
class Example extends StatefulWidget {
const Example({
super.key,
});
@override
State<Example> createState() => _ExamplePageState();
}
class _ExamplePageState extends State<Example> {
final CardSwiperController controller = CardSwiperController();
final cards = candidates.map(ExampleCard.new).toList();
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Column(
children: [
Flexible(
child: CardSwiper(
controller: controller,
cardsCount: cards.length,
onSwipe: _onSwipe,
onUndo: _onUndo,
numberOfCardsDisplayed: 3,
backCardOffset: const Offset(40, 40),
padding: const EdgeInsets.all(24.0),
cardBuilder: (
context,
index,
horizontalThresholdPercentage,
verticalThresholdPercentage,
) =>
cards[index],
),
),
Padding(
padding: const EdgeInsets.all(16.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
FloatingActionButton(
onPressed: controller.undo,
child: const Icon(Icons.rotate_left),
),
FloatingActionButton(
onPressed: () => controller.swipe(CardSwiperDirection.left),
child: const Icon(Icons.keyboard_arrow_left),
),
FloatingActionButton(
onPressed: () => controller.swipe(CardSwiperDirection.right),
child: const Icon(Icons.keyboard_arrow_right),
),
FloatingActionButton(
onPressed: () => controller.swipe(CardSwiperDirection.top),
child: const Icon(Icons.keyboard_arrow_up),
),
FloatingActionButton(
onPressed: () => controller.swipe(CardSwiperDirection.bottom),
child: const Icon(Icons.keyboard_arrow_down),
),
],
),
),
],
),
),
);
}
bool _onSwipe(
int previousIndex,
int? currentIndex,
CardSwiperDirection direction,
) {
debugPrint(
'The card $previousIndex was swiped to the ${direction.name}. Now the card $currentIndex is on top',
);
return true;
}
bool _onUndo(
int? previousIndex,
int currentIndex,
CardSwiperDirection direction,
) {
debugPrint(
'The card $currentIndex was undod from the ${direction.name}',
);
return true;
}
}
class ExampleCard extends StatelessWidget {
final ExampleCandidateModel candidate;
const ExampleCard(
this.candidate, {
super.key,
});
@override
Widget build(BuildContext context) {
return Container(
clipBehavior: Clip.hardEdge,
decoration: BoxDecoration(
borderRadius: const BorderRadius.all(Radius.circular(10)),
color: Colors.white,
boxShadow: [
BoxShadow(
color: Colors.grey.withOpacity(0.2),
spreadRadius: 3,
blurRadius: 7,
offset: const Offset(0, 3),
),
],
),
alignment: Alignment.center,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Flexible(
child: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
colors: candidate.color,
),
),
),
),
Padding(
padding: const EdgeInsets.all(16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
candidate.name,
style: const TextStyle(
color: Colors.black,
fontWeight: FontWeight.bold,
fontSize: 20,
),
),
const SizedBox(height: 5),
Text(
candidate.job,
style: const TextStyle(
color: Colors.grey,
fontSize: 15,
),
),
const SizedBox(height: 5),
Text(
candidate.city,
style: const TextStyle(color: Colors.grey),
),
],
),
),
],
),
);
}
}
class ExampleCandidateModel {
final String name;
final String job;
final String city;
final List<Color> color;
ExampleCandidateModel({
required this.name,
required this.job,
required this.city,
required this.color,
});
}
final List<ExampleCandidateModel> candidates = [
ExampleCandidateModel(
name: 'One, 1',
job: 'Developer',
city: 'Areado',
color: const [Color(0xFFFF3868), Color(0xFFFFB49A)],
),
ExampleCandidateModel(
name: 'Two, 2',
job: 'Manager',
city: 'New York',
color: const [Color(0xFF736EFE), Color(0xFF62E4EC)],
),
ExampleCandidateModel(
name: 'Three, 3',
job: 'Engineer',
city: 'London',
color: const [Color(0xFF2F80ED), Color(0xFF56CCF2)],
),
ExampleCandidateModel(
name: 'Four, 4',
job: 'Designer',
city: 'Tokyo',
color: const [Color(0xFF0BA4E0), Color(0xFFA9E4BD)],
),
];
Output
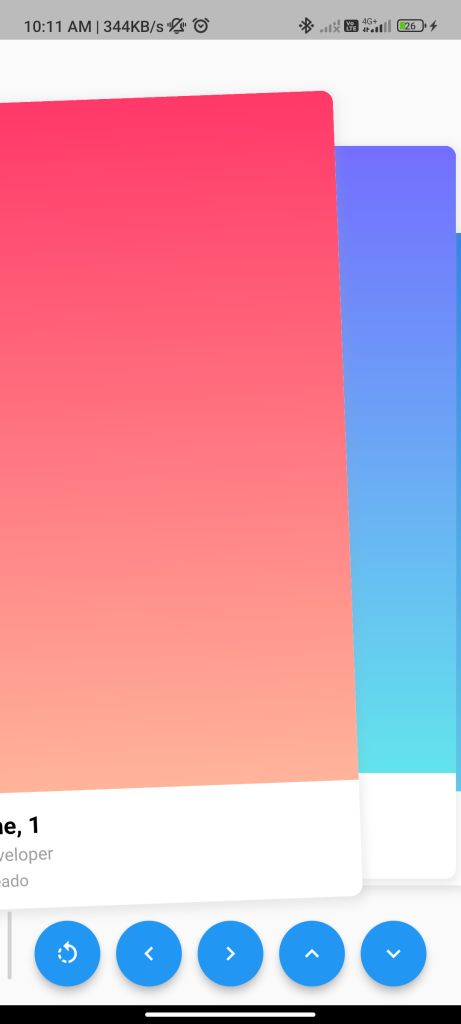
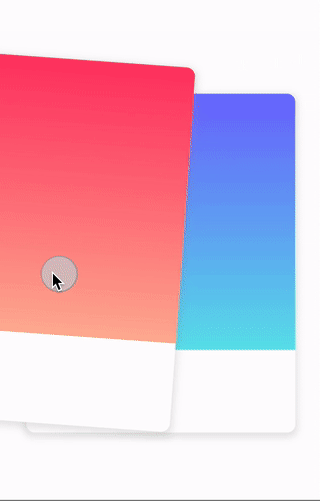
Conclusion
By following these steps, you can easily implement a card swiper in your Flutter app using the card_swiper
package. This allows you to create a swipeable card interface for various purposes in your app.