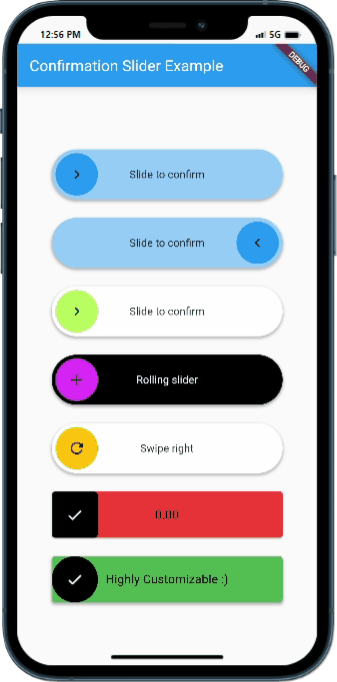
Introduction:
In Flutter, an action slider button is a user interface element that allows users to perform actions by swiping or dragging a button horizontally. It provides an intuitive and engaging way to trigger actions, such as marking an item as complete or deleting a list item. In this tutorial, we will learn how to add an action slider button in Flutter using the action_slider package and explore different customization options.
Content:
To add an action slider button in Flutter, follow these steps:
Step 1.Create a new Flutter project:
Ensure that you have Flutter installed on your machine. Open your terminal and run the following command to create a new Flutter project:
flutter create action_slider_button
Step 2. Add the action_slider dependency
Navigate to the pubspec.yaml
file of your Flutter project and add the action_slider dependency under the dependencies
section:
dependencies:
flutter:
sdk: flutter
action_slider: ^0.6.1
Save the file and run the following command in your terminal to fetch the package:
Step 3. Implement the Action Slider Button
Navigate to the lib
folder of your Flutter project and open the main.dart
file. Replace the existing code with the following implementation:
import 'package:flutter/material.dart';
import 'package:action_slider/action_slider.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Action Slider Button',
theme: ThemeData(primarySwatch: Colors.blue),
home: Scaffold(
appBar: AppBar(
title: Text('Action Slider Button'),
),
body: Center(
child: ActionSlider.standard(
sliderBehavior: SliderBehavior.stretch,
width: 300.0,
child: const Text('Slide to confirm'),
backgroundColor: Colors.white,
toggleColor: Colors.lightGreenAccent,
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
),
),
);
}
}
In this code snippet, we import the action_slider
package and utilize the ActionSlider
widget to create our action slider button. Customize the container’s appearance, such as background color, size, and content, as desired. Implement the necessary logic inside the onSlideStarted
and onSlideCompleted
callbacks to handle the slide events and perform the desired action.
Step 4. Run the App
Save the changes made to the pubspec.yaml
and main.dart
files. Execute the following command in your terminal to run the app:
flutter run
Sample Code:
import 'package:action_slider/action_slider.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Confirmation Slider Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(title: 'Confirmation Slider Example'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final _controller = ActionSliderController();
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ActionSlider.standard(
width: 300.0,
actionThresholdType: ThresholdType.release,
child: const Text('Slide to confirm'),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
const SizedBox(height: 24.0),
ActionSlider.standard(
width: 300.0,
child: const Text('Slide to confirm'),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
direction: TextDirection.rtl,
),
const SizedBox(height: 24.0),
ActionSlider.standard(
sliderBehavior: SliderBehavior.stretch,
width: 300.0,
child: const Text('Slide to confirm'),
backgroundColor: Colors.white,
toggleColor: Colors.lightGreenAccent,
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
const SizedBox(height: 24.0),
ActionSlider.standard(
rolling: true,
width: 300.0,
child: const Text('Rolling slider',
style: TextStyle(color: Colors.white)),
backgroundColor: Colors.black,
reverseSlideAnimationCurve: Curves.easeInOut,
reverseSlideAnimationDuration: const Duration(milliseconds: 500),
toggleColor: Colors.purpleAccent,
icon: const Icon(Icons.add),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
const SizedBox(height: 24.0),
ActionSlider.standard(
sliderBehavior: SliderBehavior.stretch,
rolling: true,
width: 300.0,
child: const Text('Swipe right'),
backgroundColor: Colors.white,
toggleColor: Colors.amber,
iconAlignment: Alignment.centerRight,
loadingIcon: SizedBox(
width: 55,
child: Center(
child: SizedBox(
width: 24.0,
height: 24.0,
child: CircularProgressIndicator(
strokeWidth: 2.0, color: theme.iconTheme.color),
))),
successIcon: const SizedBox(
width: 55, child: Center(child: Icon(Icons.check_rounded))),
icon: const SizedBox(
width: 55, child: Center(child: Icon(Icons.refresh_rounded))),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
const SizedBox(height: 24.0),
ActionSlider.custom(
sliderBehavior: SliderBehavior.stretch,
width: 300.0,
controller: _controller,
height: 60.0,
toggleWidth: 60.0,
toggleMargin: EdgeInsets.zero,
backgroundColor: Colors.green,
foregroundChild: DecoratedBox(
decoration: BoxDecoration(
color: Colors.black,
borderRadius: BorderRadius.circular(5)),
child: const Icon(Icons.check_rounded, color: Colors.white)),
foregroundBuilder: (context, state, child) => child!,
outerBackgroundBuilder: (context, state, child) => Card(
margin: EdgeInsets.zero,
color: Color.lerp(Colors.red, Colors.green, state.position),
child: Center(
child: Text(state.position.toStringAsFixed(2),
style: theme.textTheme.subtitle1)),
),
backgroundBorderRadius: BorderRadius.circular(5.0),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
const SizedBox(height: 24.0),
ActionSlider.custom(
toggleMargin: EdgeInsets.zero,
width: 300.0,
controller: _controller,
toggleWidth: 60.0,
height: 60.0,
backgroundColor: Colors.green,
foregroundChild: Container(
decoration: const BoxDecoration(
color: Colors.black,
borderRadius: BorderRadius.all(Radius.circular(30.0)),
),
child: const Icon(Icons.check_rounded, color: Colors.white)),
foregroundBuilder: (context, state, child) => child!,
backgroundChild: Center(
child: Text('Highly Customizable :)',
style: theme.textTheme.subtitle1),
),
backgroundBuilder: (context, state, child) => ClipRect(
child: OverflowBox(
maxWidth: state.standardSize.width,
maxHeight: state.toggleSize.height,
minWidth: state.standardSize.width,
minHeight: state.toggleSize.height,
child: child!)),
backgroundBorderRadius: BorderRadius.circular(5.0),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 3));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
controller.reset(); //resets the slider
},
),
],
),
),
);
}
}
Output:
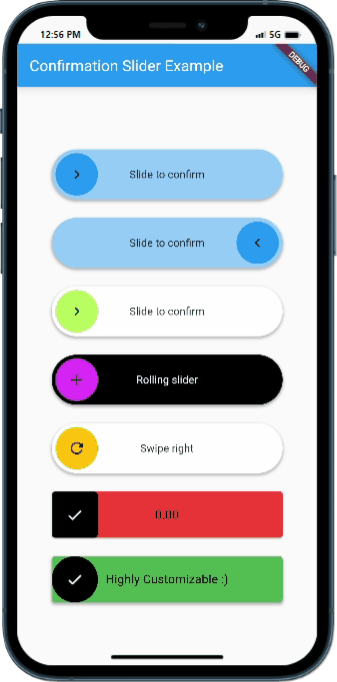
Conclusion:
Congratulations! You have successfully added an action slider button in your Flutter app using the action_slider package. By following this tutorial, you learned how to implement a customizable slider button and handle slide events to trigger actions. Feel free to explore more features and options provided by the action_slider package to enhance the functionality and appearance of your action slider button.