The Image
widget in Flutter is a versatile tool that allows you to display and style images in your app with ease. In this article, we’ll explore different examples and techniques for using the Image
widget to create visually appealing image layouts.
Example 1: Basic Image Display
To display a basic image in Flutter, you can use the Image
widget and provide the image asset path using the AssetImage
class.
Image(
image: AssetImage('assets/images/image1.jpg'),
)
Highlighted Points:
- Use the
AssetImage
class to specify the asset path of the image. - Wrap the
Image
widget with aContainer
orClipRRect
widget for additional styling if needed.
Example 2: Creating Rounded Images
To create rounded images, you can wrap the Image
widget with the ClipRRect
widget and set the borderRadius
property to create the desired shape.
ClipRRect(
borderRadius: BorderRadius.circular(10.0),
child: Image(
image: AssetImage('assets/images/image2.jpg'),
),
)
Highlighted Points:
- Use the
ClipRRect
widget to create rounded corners for the image. - Adjust the
borderRadius
property to control the roundness of the image corners.
Example 3: Adding Box Shadows
You can enhance the visual appeal of images by adding box shadows. Wrap the Image
widget with a Container
widget and set the boxShadow
property to create a shadow effect.
Container(
decoration: BoxDecoration(
boxShadow: [
BoxShadow(
color: Colors.grey,
blurRadius: 5.0,
offset: Offset(0, 2),
),
],
),
child: Image(
image: AssetImage('assets/images/image3.jpg'),
),
)
Highlighted Points:
- Use the
Container
widget to wrap theImage
widget and apply additional styling. - Set the
boxShadow
property to create a shadow effect, specifying the color, blur radius, and offset.
Example 4: Image Cards
Creating image cards is a common layout pattern. You can achieve this by using a combination of Card
and Image
widgets.
Card(
elevation: 4.0,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
child: Column(
children: [
Image(
image: AssetImage('assets/images/image4.jpg'),
),
ListTile(
title: Text('Image Title'),
subtitle: Text('Image Description'),
),
],
),
)
Highlighted Points:
- Use the
Card
widget to create a container with a material design-inspired shadow. - Combine the
Card
andImage
widgets with other UI elements likeListTile
to create an image card layout.
Example 5: Responsive Image Layouts
To create responsive image layouts, you can use the LayoutBuilder
widget to adjust the image size based on the available space.
LayoutBuilder(
builder: (context, constraints) {
return Image(
image: AssetImage('assets/images/image5.jpg'),
width: constraints.maxWidth,
height: constraints.maxHeight,
fit: BoxFit.cover,
);
},
)
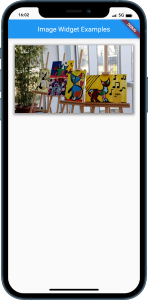
Highlighted Points:
- Use the
LayoutBuilder
widget to access the available constraints of the parent container. - Set the
width
andheight
properties of theImage
widget based on the maximum constraints to create a responsive image layout. - Adjust the
fit
property to control how the image fits within the available space.
With the Image
widget in Flutter, you can easily display and style images in your app. Whether you need basic image display, rounded images, box shadows, image cards, or responsive layouts, the Image
widget provides the necessary tools to create visually appealing image experiences in your Flutter projects.
Experiment with these examples and unleash your creativity to enhance your app’s visuals with stunning images.
Remember, images are powerful visual elements that can greatly impact your app’s user experience, so make sure to choose high-quality images that align with your app’s overall design and branding.
Happy coding and enjoy creating beautiful image layouts in Flutter!