HTTP requests are an essential part of building mobile applications that interact with web services and APIs. In Flutter, the http
package provides a convenient way to make these requests. In this article, we will explore how to utilize the http
package to perform GET and POST requests, handle responses, and manage errors.
Getting Started
Before we dive into the details, make sure you have added the http
package to your Flutter project. Open your project’s pubspec.yaml
file and add the following line under the dependencies
section:
dependencies:
http: ^0.13.3
Remember to run flutter pub get
to fetch the package after modifying your pubspec.yaml
file.
Making a GET Request
To make a GET request using the http
package, follow these steps:
- Import the necessary packages:
import 'package:http/http.dart' as http;
- Define the URL you want to request:
var url = Uri.parse('https://www.example.com/api/data');
- Send the GET request and handle the response:
void makeGetRequest() async {
var response = await http.get(url);
if (response.statusCode == 200) {
// Successful request
print(response.body);
} else {
// Request failed
print('Request failed with status: ${response.statusCode}.');
}
}
Note: Replace 'https://www.example.com/api/data'
with the actual URL you want to make a GET request to.
Making a POST Request
To make a POST request using the http
package, follow these steps:
- Import the necessary packages:
import 'package:http/http.dart' as http;
- Define the URL you want to request:
var url = Uri.parse('https://www.example.com/api/data');
- Send the POST request and handle the response:
void makePostRequest() async {
var response = await http.post(url, body: {'key': 'value'});
if (response.statusCode == 200) {
// Successful request
print(response.body);
} else {
// Request failed
print('Request failed with status: ${response.statusCode}.');
}
}
Note: Replace 'https://www.example.com/api/data'
with the actual URL you want to make a POST request to. You can provide additional parameters in the body
parameter as needed.
Handling Responses and Errors
When making HTTP requests, it’s crucial to handle the responses and potential errors effectively. Here are a few tips:
- Check the response status code: Always verify the status code of the response to determine if the request was successful (status code 200) or encountered an error. Handle different status codes accordingly.
- Parse and utilize the response data: Extract and parse the response body based on the expected data format (e.g., JSON). Use the retrieved data in your application logic or display it to the user.
- Handle request failures: If the request fails due to network issues or server errors, handle the errors gracefully. Notify the user or retry the request based on your application’s requirements.
Remember to implement error handling and exception catching mechanisms specific to your application’s needs.
Example code :
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Product List Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
List<dynamic> _products = [];
@override
void initState() {
super.initState();
fetchProducts();
}
Future<void> fetchProducts() async {
var url = Uri.parse('https://dummyjson.com/products');
var response = await http.get(url);
setState(() {
if (response.statusCode == 200) {
// Successful request
var data = json.decode(response.body);
_products = data['products'];
} else {
// Request failed
_products = [];
}
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Product List'),
),
body: ListView.builder(
itemCount: _products.length,
itemBuilder: (context, index) {
var product = _products[index];
var thumbnailUrl = product['thumbnail'];
var discount = product['discountPercentage'];
return Card(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
elevation: 4,
margin: EdgeInsets.all(8),
child: Column(
children: [
Stack(
children: [
ClipRRect(
borderRadius: BorderRadius.vertical(
top: Radius.circular(10),
),
child: Image.network(
thumbnailUrl,
width: double.infinity,
height: 200,
fit: BoxFit.cover,
),
),
if (discount != null)
Positioned(
top: 8,
right: 8,
child: Container(
padding: EdgeInsets.symmetric(
vertical: 4,
horizontal: 8,
),
decoration: BoxDecoration(
color: Colors.red,
borderRadius: BorderRadius.circular(8),
),
child: Text(
'${discount.toStringAsFixed(2)}% OFF',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
fontSize: 12,
),
),
),
),
],
),
ListTile(
title: Text(
product['title'],
style: TextStyle(
fontWeight: FontWeight.bold,
),
),
subtitle: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('Price: \$${product['price']}'),
if (discount != null)
Text('Discount: ${discount.toStringAsFixed(2)}%'),
if (product['stock'] == 0)
Text(
'Out of Stock',
style: TextStyle(
color: Colors.red,
),
),
],
),
),
],
),
);
},
),
);
}
}
Output
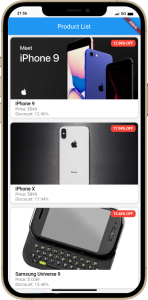
Conclusion
In this article, we explored how to make HTTP requests in Flutter using the http
package. We covered making both GET and POST requests, handling responses, and managing errors effectively. By following these practices, you can build robust Flutter applications that interact seamlessly with web services and APIs.
Remember to optimize your application’s performance by utilizing background isolates, managing request headers, and employing appropriate error handling mechanisms.
Happy coding!
Important Points:
- The
http
package in Flutter provides convenient methods for making HTTP requests. - Always check the response status code to handle successful requests and errors accordingly.
- Parse and utilize the response data based on the expected format (e.g., JSON).
- Implement proper error handling mechanisms for network failures or server errors.
- Optimize your application’s performance by utilizing background isolates and managing request headers.
By following these guidelines, you can effectively make HTTP requests in Flutter using the http
package.
Disclaimer: The code snippets provided in this article are for illustrative purposes and may require modifications based on your specific use case.