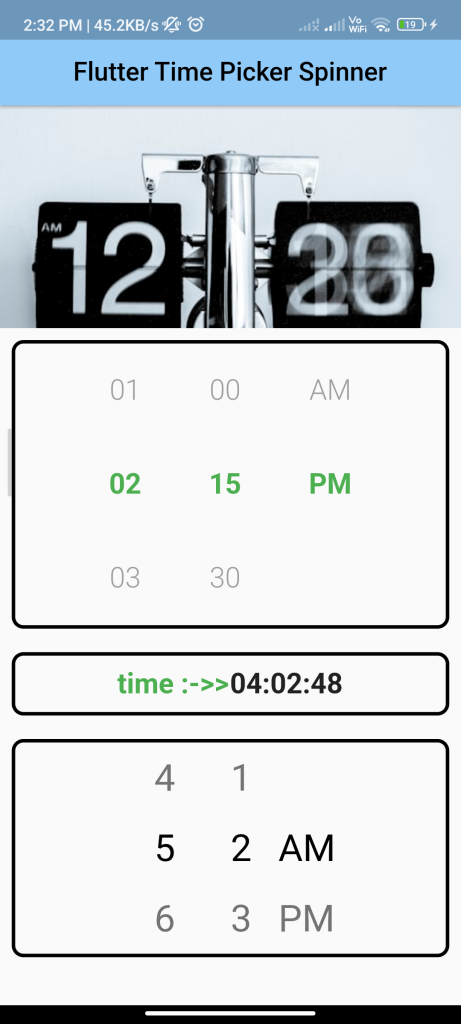
Introduction:
Implementing a time picker spinner in Flutter allows users to select a time value using a spinner interface. The flutter_time_picker_spinner
package provides a simple way to integrate a time picker spinner into your app. This tutorial will guide you through implementing a time picker spinner using the flutter_time_picker_spinner
package.
Content:
Step 1: Add the dependency:
Include the flutter_time_picker_spinner
package in your pubspec.yaml
file:
dependencies:
flutter_time_picker_spinner: ^2.0.0
Run flutter pub get
to install the package.
Step 2: Import the package:
Import the flutter_time_picker_spinner
package in your Dart file:
import 'package:flutter/material.dart';
import 'package:flutter_time_picker_spinner/flutter_time_picker_spinner.dart';
Step 3: Create a TimePickerSpinner widget:
Define a stateful widget and implement the TimePickerSpinner
widget to provide a time picker spinner:
class TimePickerSpinnerScreen extends StatefulWidget {
@override
_TimePickerSpinnerScreenState createState() =>
_TimePickerSpinnerScreenState();
}
class _TimePickerSpinnerScreenState extends State<TimePickerSpinnerScreen> {
DateTime _selectedTime = DateTime.now();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Time Picker Spinner Example'),
),
body: Center(
child: TimePickerSpinner(
is24HourMode: false,
normalTextStyle: TextStyle(fontSize: 24, color: Colors.deepOrange),
highlightedTextStyle: TextStyle(fontSize: 24, color: Colors.green),
spacing: 50,
itemHeight: 80,
isForce2Digits: true,
onTimeChange: (time) {
setState(() {
_selectedTime = time;
});
},
),
),
);
}
}
In this example, the TimePickerSpinner
widget is created with various customization options such as 24-hour mode, text styles, spacing, item height, and force 2 digits for hour and minute. The onTimeChange
callback updates the selected time.
Step 4: Run the app:
Run your Flutter app to see the time picker spinner in action. You should see a spinner interface that allows you to select a time.
Sample Code:
// ignore_for_file: prefer_interpolation_to_compose_strings, prefer_const_constructors, unnecessary_new
import 'package:flutter/material.dart';
import 'package:flutter_time_picker_spinner/flutter_time_picker_spinner.dart';
class Spinner_time_picker extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Time Picker Spinner Demo',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
home: MyHomePage(
title: 'Flutter Time Picker Spinner Demo',
),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, required this.title}) : super(key: key);
// This widget is the home page of your application. It is stateful, meaning
// that it has a State object (defined below) that contains fields that affect
// how it looks.
// This class is the configuration for the state. It holds the values (in this
// case the title) provided by the parent (in this case the App widget) and
// used by the build method of the State. Fields in a Widget subclass are
// always marked "final".
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
DateTime _dateTime = DateTime.now();
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
child: new Column(
children: <Widget>[
Container(
height: 190,
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(
"https://images.unsplash.com/photo-1456574808786-d2ba7a6aa654?w=500&auto=format&fit=crop&q=60&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxzZWFyY2h8MTZ8fHRpbWV8ZW58MHx8MHx8fDA%3D"),
fit: BoxFit.cover)),
),
// hourMinute12H(),
// hourMinuteSecond(),
Container(
margin: EdgeInsets.symmetric(vertical: 10, horizontal: 10),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(10), border: Border.all(color: Colors.black, width: 3)),
child: hourMinute12HCustomStyle()),
new Container(
margin: EdgeInsets.symmetric(vertical: 10, horizontal: 10),
padding: EdgeInsets.symmetric(vertical: 10, horizontal: 10),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(10), border: Border.all(color: Colors.black, width: 3)),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"time :->>",
style: TextStyle(fontSize: 24, color: Colors.green, fontWeight: FontWeight.bold),
),
new Text(
_dateTime.hour.toString().padLeft(2, '0') +
':' +
_dateTime.minute.toString().padLeft(2, '0') +
':' +
_dateTime.second.toString().padLeft(2, '0'),
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold),
),
],
),
),
Container(
margin: EdgeInsets.symmetric(vertical: 10, horizontal: 10),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(10), border: Border.all(color: Colors.black, width: 3)),
child: hourMinute12H(),
// hourMinute15Interval()
),
],
),
));
}
/// SAMPLE
Widget hourMinute12H() {
return new TimePickerSpinner(
is24HourMode: false,
onTimeChange: (time) {
setState(() {
_dateTime = time;
});
},
);
}
Widget hourMinuteSecond() {
return new TimePickerSpinner(
isShowSeconds: true,
onTimeChange: (time) {
setState(() {
_dateTime = time;
});
},
);
}
Widget hourMinute15Interval() {
return new TimePickerSpinner(
spacing: 40,
minutesInterval: 15,
onTimeChange: (time) {
setState(() {
_dateTime = time;
});
},
);
}
Widget hourMinute12HCustomStyle() {
return new TimePickerSpinner(
is24HourMode: false,
normalTextStyle: TextStyle(fontSize: 24, color: Colors.grey, fontWeight: FontWeight.w300),
highlightedTextStyle: TextStyle(fontSize: 24, fontWeight: FontWeight.bold, color: Colors.green),
spacing: 40,
itemHeight: 80,
isForce2Digits: true,
alignment: Alignment.center,
minutesInterval: 15,
onTimeChange: (time) {
setState(() {
_dateTime = time;
});
},
);
}
}
Output:
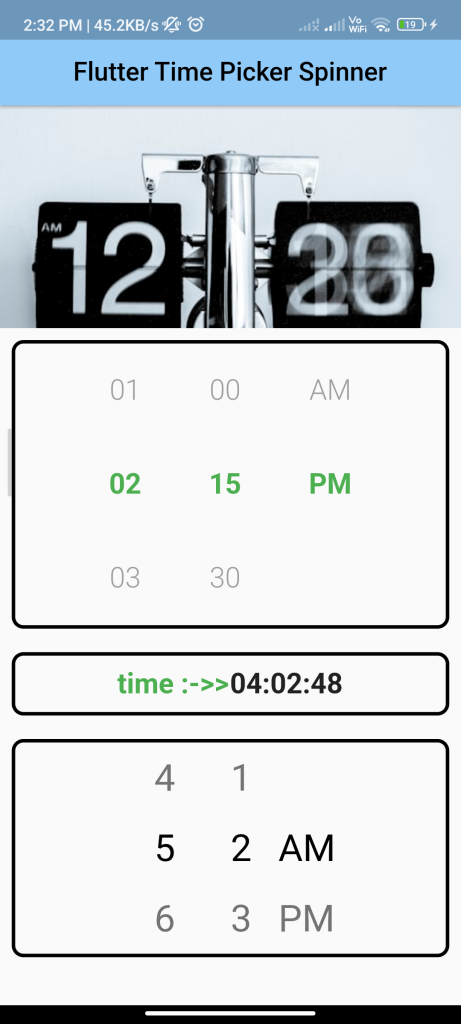
Conclusion:
By following these steps, you can easily implement a time picker spinner in Flutter using the flutter_time_picker_spinner
package. This provides a user-friendly interface for selecting time values in your app.