Introduction:
As a beginner in Flutter, one of the key components you’ll need to learn is how to create a signup form. A signup form allows users to register for an application or service, providing their essential details. In this article, we’ll walk you through the process of designing and implementing a signup form in Flutter. By the end of this guide, you’ll have a clear understanding of how to create a user-friendly signup form that seamlessly integrates with your Flutter app.
Understanding the Importance of Signup Forms
Signup forms play a crucial role in the user onboarding process. They act as gateways to the digital world, allowing users to create accounts and access various platforms and services. Whether it’s a social media platform, an e-commerce store, or a productivity app, signup forms are the first interaction point for users. It’s essential to understand the importance of creating a well-designed and intuitive signup form to ensure a smooth user experience.
Key Elements of a Signup Form
Before diving into the code, let’s discuss the key elements that make up a signup form. These elements include:
1. Name Field
The name field allows users to enter their full name. It’s essential to make this field clear and user-friendly by providing proper labeling and validation.
2. Email Field
The email field collects the user’s email address, which serves as their primary contact information. Implement proper email validation to ensure the accuracy of the entered email address.
3. Password Field
The password field allows users to create a secure password to protect their accounts. Consider implementing password strength validation and provide clear instructions on password requirements.
4. Sign Up Button
The sign-up button triggers the registration process. It’s crucial to make the button visually appealing and easily clickable.
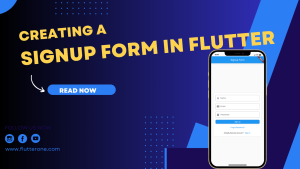
Creating a Seamless Signup Experience: Step-by-Step Guide
Now, let’s dive into the step-by-step process of creating a signup form in Flutter. We’ll cover the UI design and implementation of the signup logic.
Step 1: Set up a new Flutter project
First, create a new Flutter project or navigate to an existing project directory.
Step 2: Design the signup form UI
In the lib
folder, create a new Dart file, such as signup_form.dart
. Open the file and define the signup form UI using Flutter widgets.
// Code snippet for the signup form UI
import 'package:flutter/material.dart';
class SignupForm extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Signup Form'),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
TextField(
decoration: InputDecoration(
labelText: 'Name',
),
),
SizedBox(height: 16.0),
TextField(
decoration: InputDecoration(
labelText: 'Email',
),
),
SizedBox(height: 16.0),
TextField(
decoration: InputDecoration(
labelText: 'Password',
),
obscureText: true,
),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: () {
// TODO: Implement signup logic
},
child: Text('Sign Up'),
),
],
),
),
);
}
}
Step 3: Implement signup logic
Inside the onPressed
callback of the “Sign Up” button, you can implement the logic for signing up the user. This might involve validating the form input, making API calls, and handling success or error scenarios.
Step 4: Integrate the signup form in the app
Open the main.dart
file in the lib
folder and replace the existing code with the following:
// Code snippet for integrating the signup form in the app
import 'package:flutter/material.dart';
import 'signup_form.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Signup Form',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: SignupForm(),
);
}
}
Step 5: Run the app
Save the changes and run the Flutter app using the flutter run
command. The app will launch, and you will see the signup form UI.
Sample Code
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Signup Form',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: SignupPage(),
);
}
}
class SignupPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Signup Form'),
),
body: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('assets/images/background_image.jpg'),
fit: BoxFit.cover,
),
),
child: Center(
child: SingleChildScrollView(
child: Padding(
padding: EdgeInsets.all(16.0),
child: Card(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(16.0),
),
child: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Name',
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(8.0),
),
prefixIcon: Icon(Icons.person),
),
),
SizedBox(height: 16.0),
TextFormField(
decoration: InputDecoration(
labelText: 'Email',
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(8.0),
),
prefixIcon: Icon(Icons.email),
),
keyboardType: TextInputType.emailAddress,
),
SizedBox(height: 16.0),
TextFormField(
decoration: InputDecoration(
labelText: 'Password',
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(8.0),
),
prefixIcon: Icon(Icons.lock),
),
obscureText: true,
),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: () {
// TODO: Implement signup logic
},
child: Text('Sign Up'),
style: ElevatedButton.styleFrom(
primary: Colors.blue,
onPrimary: Colors.white,
padding: EdgeInsets.symmetric(vertical: 16.0),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(8.0),
),
),
),
SizedBox(height: 16.0),
Align(
alignment: Alignment.center,
child: TextButton(
onPressed: () {
// TODO: Implement forgot password logic
},
child: Text('Forgot Password?'),
style: TextButton.styleFrom(
primary: Colors.blue,
),
),
),
SizedBox(height: 16.0),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Already have an account?'),
SizedBox(width: 4.0),
TextButton(
onPressed: () {
// TODO: Implement sign-in logic
},
child: Text('Sign In'),
style: TextButton.styleFrom(
primary: Colors.blue,
),
),
],
),
],
),
),
),
),
),
),
),
);
}
}
Output
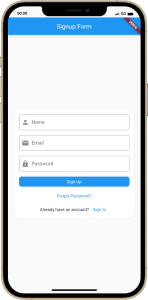
Conclusion
Creating a signup form in Flutter is a fundamental step in building user registration functionality. By following this step-by-step guide, you’ve learned how to design a user-friendly signup form and implement the necessary logic for a seamless registration experience. With this knowledge, you can now confidently create signup forms for your Flutter apps and provide users with a smooth onboarding process.